用 Python 将两个列表相乘
Muhammad Waiz Khan
2023年1月30日
Python
Python List
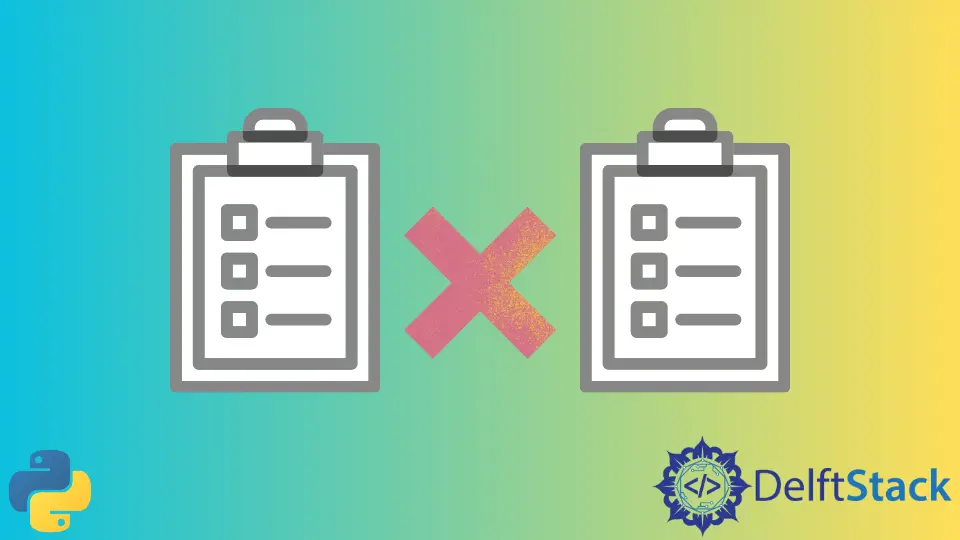
本教程将演示在 Python 中执行两个列表元素相乘的各种方法。假设我们有两个维度相同的整数列表,我们想将第一个列表中的元素与第二个列表中相同位置的元素相乘,得到一个维度相同的结果列表。
在 Python 中使用 zip()
方法将两个列表相乘
在 Python 中内置的 zip()
方法接受一个或多个可迭代对象并将可迭代对象聚合成一个元组。像列表 [1,2,3]
和 [4,5,6]
将变成 [(1, 4), (2, 5), (3, 6)]
。使用 map()
方法,我们按元素访问两个列表,并使用列表推导方法得到所需的列表。
下面的代码示例展示了如何使用 zip()
与列表推导式来复用 1D 和 2D 列表。
list1 = [2, 4, 5, 3, 5, 4]
list2 = [4, 1, 2, 9, 7, 5]
product = [x * y for x, y in zip(list1, list2)]
print(product)
输出:
[8, 4, 10, 27, 35, 20]
2D 列表的乘法:
list1 = [[2, 4, 5], [3, 5, 4]]
list2 = [[4, 1, 2], [9, 7, 5]]
product = [[0] * 3] * 2
for x in range(len(list1)):
product[x] = [a * b for a, b in zip(list1[x], list2[x])]
print(product)
输出:
[[8, 4, 10], [27, 35, 20]]
在 Python 中使用 numpy.multiply()
方法对两个列表进行乘法
Python 中 NumPy
库的 multiply()
方法,将两个数组/列表作为输入,进行元素乘法后返回一个数组/列表。这个方法很直接,因为我们不需要为二维乘法做任何额外的工作,但是这个方法的缺点是没有 NumPy
库就不能使用。
下面的代码示例演示了如何在 Python 中使用 numpy.multiply()
方法对 1D 和 2D 列表进行乘法。
- 1D 乘法:
import numpy as np
list1 = [12, 3, 1, 2, 3, 1]
list2 = [13, 2, 3, 5, 3, 4]
product = np.multiply(list1, list2)
print(product)
输出:
[156 6 3 10 9 4]
- 2D 乘法:
import numpy as np
list1 = [[12, 3, 1], [2, 3, 1]]
list2 = [[13, 2, 3], [5, 3, 4]]
product = np.multiply(list1, list2)
print(product)
输出:
[[156 6 3]
[ 10 9 4]]
在 Python 中使用 map()
函数将两个列表相乘
map
函数接收一个函数和一个或多个迭代数作为输入,并返回一个迭代数,将所提供的函数应用于输入列表。
我们可以在 Python 中使用 map()
函数,通过将两个列表作为参数传递给 map()
函数,对两个列表进行 1D 和 2D 元素乘法。下面的代码示例演示了我们如何使用 map()
对两个 Python 列表进行乘法。
一维乘法的示例代码:
list1 = [2, 4, 5, 3, 5, 4]
list2 = [4, 1, 2, 9, 7, 5]
product = list(map(lambda x, y: x * y, list1, list2))
print(product)
输出:
[8, 4, 10, 27, 35, 20]
2D 乘法的示例代码:
list1 = [[2, 4, 5], [3, 5, 4]]
list2 = [[4, 1, 2], [9, 7, 5]]
product = [[0] * 3] * 2
for x in range(len(list1)):
product[x] = list(map(lambda a, b: a * b, list1[x], list2[x]))
print(product)
输出:
[[8, 4, 10], [27, 35, 20]]
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe