Multiple Exceptions in Python
- Use of Commas and Parenthesis for Catching Multiple Exceptions
-
Use the
suppress()
Function for Catching Multiple Exceptions
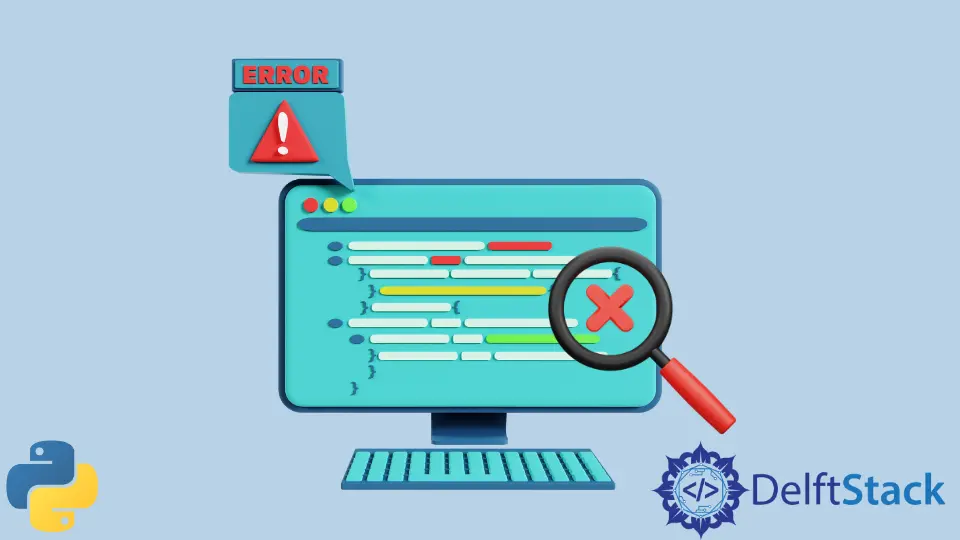
In Programming, an exception is an occurrence that disturbs the normal flow of the program. In Python, it is represented as an error. An exception can be of different types depending on its cause like IndexError, KeyError, and more.
We can use the try
and except
block to deal with exceptions. The try
block contains some code that may raise an exception, and if an exception is raised then we can specify the alternate code in the except
block.
For example,
try:
print(5 / 0)
except:
print(5 / 1)
Output:
5
The 5/0
raises an exception so it executes the code in the except
block.
Note that it is possible to raise exceptions manually also using the raise
keyword.
We can also deal with multiple exceptions in Python. We know that we have different types of exceptions in Python, so we can have multiple except
statements for different exceptions.
For example,
try:
raise ValueError()
except ValueError:
print("Value Error")
except KeyError:
print("Key Error")
Output:
Value Error
It is also possible to catch multiple exceptions with one except
statement. These methods are discussed below.
Use of Commas and Parenthesis for Catching Multiple Exceptions
The first way to achieve this is by separating the exceptions with a comma and putting them in parenthesis. The following code shows how.
try:
raise ValueError()
except (ValueError, KeyError):
print("Error")
Output:
Error
If any exception from the one mentioned in the parenthesis is encountered then the code in this block is executed. We can also assign the exception object (also called the error object) some name. e
is the most commonly used name for the error object. For example,
try:
raise ValueError()
except (ValueError, KeyError) as e:
print("Error")
Output:
Error
The as
keyword creates the alias for the name of the object. Below Python 2.5 it was possible to eliminate the use of the as
keyword by simply separating the name for error object using a comma as shown below.
try:
raise ValueError()
except (ValueError, KeyError), e:
print("Error")
Output:
Error
Use the suppress()
Function for Catching Multiple Exceptions
The contextlib
library provides a very useful function called suppress()
which can also be used for handling multiple exceptions.
This function combines the try
, except
, and pass
statement to one line of code. It is used with the with
statement which is also used in exception handling and makes the code cleaner and readable.
The following code shows how to use this function.
from contextlib import suppress
with suppress(FileNotFoundError):
os.remove("somefile.tmp")
The above code is equivalent to the following program with try
, except
and pass
statements.
try:
os.remove("somefile.tmp")
except FileNotFoundError:
pass
The pass
statement is used when we don’t want to execute anything but avoid any error for empty code.
We can use the suppress
function for multiple exceptions as shown below.
from contextlib import suppress
with suppress(FileNotFoundError, KeyError):
os.remove("somefile.tmp")
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn