Python Math.floor() Method
-
Syntax of Python
math.floor()
Method -
Example Code: Use of the
math.floor()
Method -
Example Code: Difference Between the
math.floor()
andmath.ceil()
Methods -
Example Code: Difference Between the
int()
andfloor()
Methods -
Example Code: Python Double Slash (
//
) Operator vs.math.floor()
Method for Floor Division -
Example Code: Cause of the
TypeError
When Using themath.floor()
Method
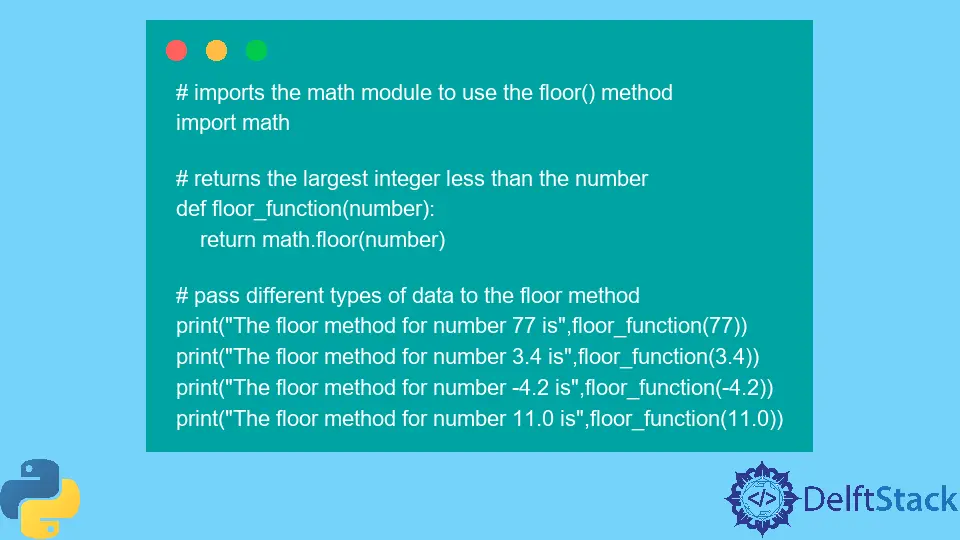
In Python, the math
module provides different mathematical functions for our use. The math.floor()
method is one of its functions, which takes a single parameter as a float number and returns the greatest integer number less than the provided number.
If the specified number is an integer, the method returns the same number as it is. The method math.floor()
was introduced in Python 2.0 and used to return a float number.
However, in Python 3.0 and later versions, the method math.floor
always returns the integer data type. The math.floor()
method is also part of theoretical and representation functions in Python documentation.
Syntax of Python math.floor()
Method
math.floor(number)
Parameter
number |
This is a required parameter in numeric form. This parameter represents the number that needs to round down. |
Return
This method returns the greatest or largest integer less than the provided parameter in integer form. Before Python version 3.0, it produces a float value.
Example Code: Use of the math.floor()
Method
To use the method floor()
, we must import the math
module in Python. The correct way to use the method is to write the math.floor()
and pass a numeric value to it.
As shown in the below example, the math.floor()
method returns the largest integer less than the provided numeric parameter.
# imports the math module to use the floor() method
import math
# returns the largest integer less than the number
def floor_function(number):
return math.floor(number)
# pass different types of data to the floor method
print("The floor method for number 77 is", floor_function(77))
print("The floor method for number 3.4 is", floor_function(3.4))
print("The floor method for number -4.2 is", floor_function(-4.2))
print("The floor method for number 11.0 is", floor_function(11.0))
Output:
# return integer values for different types of input values
The floor method for number 77 is 77
The floor method for number 3.4 is 3
The floor method for number -4.2 is -5
The floor method for number 11.0 is 11
Example Code: Difference Between the math.floor()
and math.ceil()
Methods
We have already seen how the floor()
method works. The math.ceil()
method also takes a single required numeric parameter and returns the smallest integer greater than or equal to the provided numeric parameter.
To differentiate between the floor()
and ceil()
methods of the math
module, the below example illustrates the results.
import math
# function returns the greatest integer value but less than the provided number
def floor_function(number):
return math.floor(number)
# function returns the smallest integer value but greater than the provided number
def ceil_function(number):
return math.ceil(number)
# function test on positive and negative numbers each
print("The ceil method for number -1.56 is", ceil_function(-1.56))
print("The floor method for number -1.56 is", floor_function(-1.56))
print("The ceil method for number 23.22 is", ceil_function(23.22))
print("The floor method for number 23.22 is", floor_function(23.22))
Output:
# ceil returns the smallest integer less than the provided number
The ceil method for number -1.56 is -1
The floor method for number -1.56 is -2
The ceil method for number 23.22 is 24
# floor returns the largest integer greater than the provided number
The floor method for number 23.22 is 23
Example Code: Difference Between the int()
and floor()
Methods
As we know from Python 3.0 and later versions, the floor()
method returns the integer value. Similarly, the built-in Python method int()
also returns the integer.
So the question is, what is the difference between the floor()
and int()
methods now?
The below example shows the same output when a positive numeric value passes. However, both method shows different results when a negative numeric value is given.
import math
def floor_function(number):
return math.floor(number)
print("The floor method for a positive number is", floor_function(1.6))
print("The built-in int() method for a positive number is", int(1.6))
# int() and floor() difference can be seen with negative input value
print("The floor method for a negative number is", floor_function(-1.6))
print("The built-in int() method for a negative number is", int(-1.6))
Output:
The floor method for a positive number is 1
The built-in int() method for a positive number is 1
# different return value when input is a negative number
The floor method for a negative number is -2
The built-in int() method for a negative number is -1
Example Code: Python Double Slash (//
) Operator vs. math.floor()
Method for Floor Division
In Python, the double slash //
operator divides the numbers and outputs the floor float number. Similarly, if we use the divide operator /
, it only divides the two numbers, and then we use the floor()
method to output the floor number.
The difference appears in the outputs of both methods, where the floor()
method returns the integer type, and the double slash //
operator returns the float number.
import math
num1 = -10.1
num2 = -3.22
# double slash always returns float value
print("The result of the double slash operator is", num1 // num2)
print("The result of the floor() method is", math.floor(num1 / num2))
Output:
# double slash // operator returns a float number
The result of the double slash operator is 3.0
# floor() method returns an integer
The result of the floor() method is 3
Example Code: Cause of the TypeError
When Using the math.floor()
Method
In math.floor()
, we require a single parameter to be a numeric value. If we pass anything except a numeric value, it returns a TypeError
.
The math.floor()
method only takes integer or float values as a parameter.
import math
# this function collects the incorrect data type for math.floor method
def data_type(value):
return math.floor(value)
print("String data type is pass ", data_type("Hello"))
Output:
# method returns type error for the string data
TypeError: must be real number, not str