How to Raise Exception in Python
- Raise Exception in Python
-
The
try
Statement in Python -
Manually Raise Exceptions With the
raise
Statement in Python -
The
else
Clause in Python
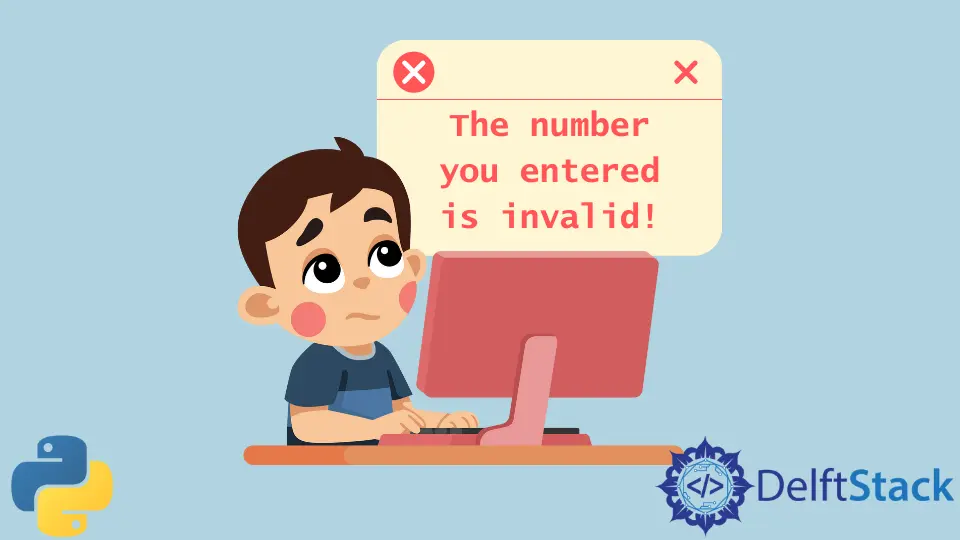
We will introduce different ways to raise an exception in Python.
Raise Exception in Python
When we write Python programs and run them, it gives an output or an error if something is missing in the program or something is wrong in the code. Exceptions are something like that in the Python programming language.
Python has many built-in exceptions raised when our program is doing something wrong. If any exceptions occur when we are trying to run the program, it will stop the program and display the exception that occurred when the program tried to run the code.
This article demonstrates how to handle exceptions in our Python program using try
, except
, and finally
statements by doing some simple programs.
The try
Statement in Python
In Python, we can handle the exceptions by using the try
statements inside our code to minimize the chances of exceptions. The try
clause contains the critical operation that can produce an exception.
The except
clause contains the code that resolves exceptions.
Now, let’s go through an example and use a try
statement to raise an exception if the user enters the wrong value.
# python
Any_List = [2, "Husnain", 4, 6, 8]
for value in Any_List:
try:
print("The value is", value)
raci = 1 / int(value)
print("The reciprocal of", value, "is", raci)
print("")
except:
print("Oops! There is a string in this list.")
print("The reciprocal of", value, "cannot be done!")
print("")
Output:
The example above shows this program goes through the entries from the Any_List
, and if there are no exceptions, the except
block will be skipped, and the code will smoothly run until it has reached to last element from the Any_List
.
But, if there is some exception while going through all the elements from the Any_List
, the except
block will be executed, and it will print the values as shown in the above result.
Now, let’s have an example in which we will create three different except
cases. We mention the try
statement with only one except
block in the above program.
But in this program, we use three except
blocks which give more options to select the clause.
# python
VAR0 = 10
try:
VAR1 = int("Husnain")
VAR2 = int(56)
result = (int(VAR1) * int(VAR2)) / (VAR0 * int(VAR2))
except ValueError as ve:
print(ve)
exit()
except TypeError as te:
print(te)
exit()
except:
print("Unexpected Error!")
exit()
print(result)
Output:
This is a program that contains the three exceptions blocks. Now, let’s go through another method, raise
, that can be used to raise an exception in Python.
Manually Raise Exceptions With the raise
Statement in Python
When there are some errors in code during runtime in Python programming, exceptions are raised. We can use the raise
keyword to raise exceptions manually.
We can also pass the values to the exception to provide more information about the exception and why the program raised it.
Let’s have an example in which we will use the raise
keyword to raise an error manually.
# python
try:
num = int(-23)
if num <= 0:
raise ValueError("entred number is not positive")
except ValueError as ve:
print(ve)
Output:
The example above shows that entering the negative number raises an exception that we set through the raise
keyword.
Now, let’s go through another example in which we will use the else
clause to raise an exception manually.
The else
Clause in Python
In some situations, when we want to run a program under the try
statement without any error, we will use the else
statement to complete the program processing.
# python
try:
number = int(21)
assert number % 2 == 0
except:
print("It is not a even number!")
else:
reciprocal = 1 / number
print(reciprocal)
Output:
As you can see from the above example, if the entered number is even, then the program outputs the reciprocal of the number, and if the number is not even, it will raise an exception, as shown in the above results.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn