List vs. Dictionary in Python
- List vs. Dictionary in Python Based on Structure
- List vs. Dictionary in Python Based on Accessing Elements
- List vs. Dictionary in Python Based on Time
- List vs. Dictionary in Python Based on Operations
- Conclusion
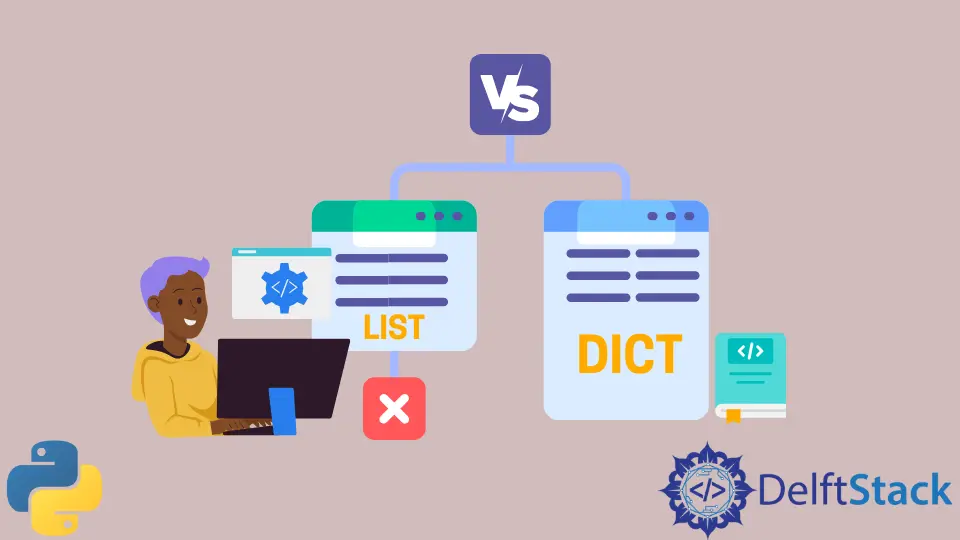
Dictionaries and lists have many similarities and are used in the JSON format. We can store and access elements with relative ease with both objects.
Both dictionaries and lists are mutable, which means we can change them and update the elements as the code goes on. This is not a common property because other objects like tuples are immutable in Python.
This tutorial will explain the differences between a list and a dictionary in Python. This can be useful to determine which object to use in different situations.
List vs. Dictionary in Python Based on Structure
The first difference is associated with how elements are stored - a list stores elements as a collection with an array-like structure.
These elements can be of any type, and there can be a mix of different element types in a list. To create lists, we use the square brackets []
.
However, a dictionary stores elements as key-value pairs, each having an associated key.
The key can access the given element in a list. Curly braces {}
are used to create a dictionary in Python.
Example:
l = [1, 8, 9, 5, 2]
d = {"a": 1, "b": 8, "c": 9, "d": 5, "e": 2}
print(l, "\n", d)
Output:
[1, 8, 9, 5, 2]
{'a': 1, 'b': 8, 'c': 9, 'd': 5, 'e': 2}
A list can also store duplicate elements, whereas, in a dictionary, every key should be unique.
List vs. Dictionary in Python Based on Accessing Elements
Elements are stored in a given order with a specific index in a list. We use the index to access them in a list.
A dictionary used to be an unordered collection. However, in Python 3.6, a few changes were made, and order is preserved in a dictionary to some extent.
To access elements, we use their associated keys.
See the code below.
l = [1, 8, 9, 5, 2]
d = {"a": 1, "b": 8, "c": 9, "d": 5, "e": 2}
print(l[4], d["b"])
Output:
2 8
The above example demonstrates how elements are accessed in a list and dictionary.
List vs. Dictionary in Python Based on Time
There is also a difference in the time and space required while accessing elements between them. Dictionaries are more efficient in this case.
A dictionary stores elements in hash tables with keys mapping to different elements. Due to this, they can be accessed without any space-time tradeoff.
The entire list is traversed to access any element, so it usually takes up more time and space. This is more visible when we work with a large dataset.
Time and space should be factored in carefully before selecting one of the two.
List vs. Dictionary in Python Based on Operations
Several common operations can apply to a list efficiently. The list
class has different functions like max()
, min()
, len()
, and many more.
We can also traverse lists easily using a for
loop. Other mutable object operations like del
, append()
, insert()
, and more are also available for lists.
Primarily, the dictionary stores and maps elements with their keys, so it does not have many associated operations. However, the dict
class is a sub-class for other dictionary-like objects such as UnorderedDict
and defaultdict
.
We will use some of these in the code below.
from collections import defaultdict
l = [1, 8, 9, 5, 2]
d = defaultdict()
d["a"] = 1
d["c"] = 3
d["e"] = 5
print(max(l), len(l))
print(d)
Output:
9 5
defaultdict(None, {'a': 1, 'c': 3, 'e': 5})
We find the maximum element and length of the string in the above example. Also, we used the defaultdict
class from the collections module.
Conclusion
Lists are favorable when we wish to store data in a specific order and perform different array-like operations on the elements. Dictionaries are useful for storing large amounts of data with easy access and mapping the elements in a hash table.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python