List Remove by Index in Python
- Remove Items From a List by Index in Python
-
Use the
pop()
Function in Python -
Use the
del
Function in Python -
Use the
remove()
Function in Python -
Use the
clear()
Method in Python - Use the Comprehension Method in Python
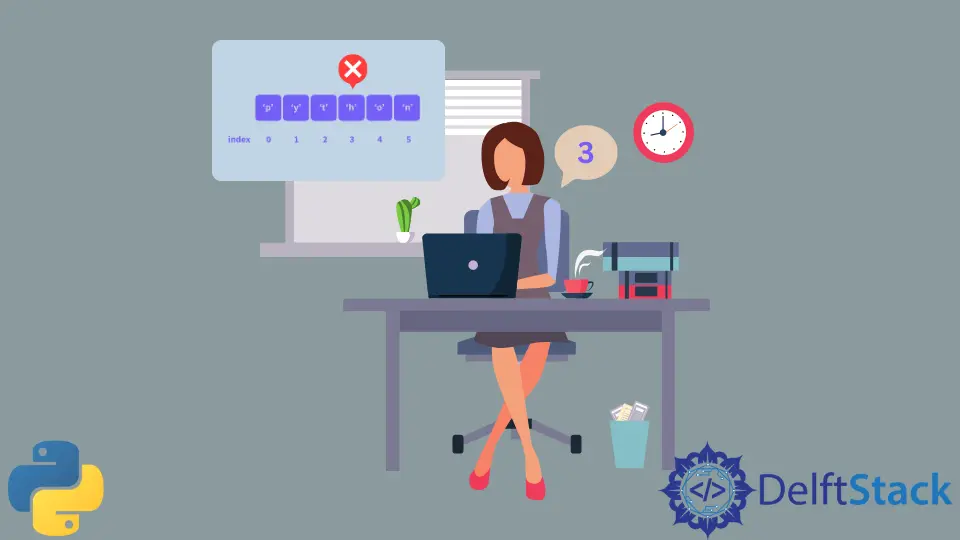
We will introduce different methods with examples to remove items from a list by index in Python.
Remove Items From a List by Index in Python
When working on applications, we have to remove the element from a given list based on their index in that list for various reasons, and we want to know which methods can be best for different situations. Many Python methods remove the element from a given list based on its index.
In this tutorial, we’ll discuss some of them. Let’s discuss each of these options individually.
pop()
functiondel[]
functionremove()
functionclear()
function- List comprehension Method
Use the pop()
Function in Python
The pop()
function removes a number from any index from a list. Any element from the list can be removed using the pop()
function.
Let’s go through an example in which we will remove the element at index 4
, which is "python"
by using the pop()
function.
Code:
# python
remove_list = ["My", "favorite", "language", "is", "python"]
remove_list.pop(4)
print(remove_list)
Output:
Using the pop()
function, we can easily remove an element from the list based on the index. Remember that the array’s index always starts from 0
.
Use the del
Function in Python
The del
function is another built-in python function that can also be used to remove an element from the list in python. We can use the del
function to delete a single or several elements from a list based on the index.
In the following example, delete "python"
from the list we used in the above example.
Code:
# python
remove_list = ["My", "favorite", "language", "is", "python"]
del remove_list[4]
print(remove_list)
Output:
If we want to delete multiple elements from a list, we can use the del
function.
# python
remove_list = ["My", "favorite", "language", "is", "python"]
del remove_list[2:5]
print(remove_list)
Output:
We can use the index to delete multiple items from the list from the above example. We will provide the starting index, from which python will start deleting the elements from the list.
We’ll also provide the ending index, till which all the items will be deleted, leaving the ending index as it is that we provided in the method.
Use the remove()
Function in Python
In the remove()
function, we can delete the element by directly entering the element into the function. This function will delete the item from the list by matching the item from the list.
If the same item is duplicated, and we want to delete it from the list, this function will delete the item only once when it appears while searching through the list.
In the next example with the same list, we will add "python"
twice and check how the remove()
function works with duplicates, as shown below.
Code:
# python
remove_list = ["My", "favorite", "python", "language", "is", "python"]
remove_list.remove("python")
print(remove_list)
Output:
When we tried to remove the word python from the list from the above example, it only deleted the "python"
when it appeared first in the index order of the list, and it left the second python.
Use the clear()
Method in Python
The clear()
method is used in Python programming to erase the entire list. This function does not delete a single or several elements from a list.
Instead, it deletes the entire list. After applying this function to the list, it gives an empty list as an output.
Code:
# python
remove_list = ["My", "favorite", "python", "language", "is", "python"]
remove_list.clear()
print(remove_list)
Output:
The clear()
method can only be used when we want to delete the whole list of items instead of a single item or multiple items.
Use the Comprehension Method in Python
In the comparison method, we can delete the elements by comparing them with a specific element. In this method, if we duplicate elements that we are trying to delete from the list, it will delete all the elements that’ll match the element.
Let’s use the above list and try to delete "python"
from the list.
Code:
# python
remove_list = ["My", "favorite", "python", "language", "is", "python"]
for i in remove_list:
if i == "python":
remove_list.remove("python")
print(remove_list)
Output:
We can delete multiple items that match our criteria using this comprehension method. This method can delete all the instances of the same element from the list.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python