How to Get List Intersection in Python
-
Get List Intersection in Python With the
&
Operator -
Get List Intersection in Python With the
intersection()
Method
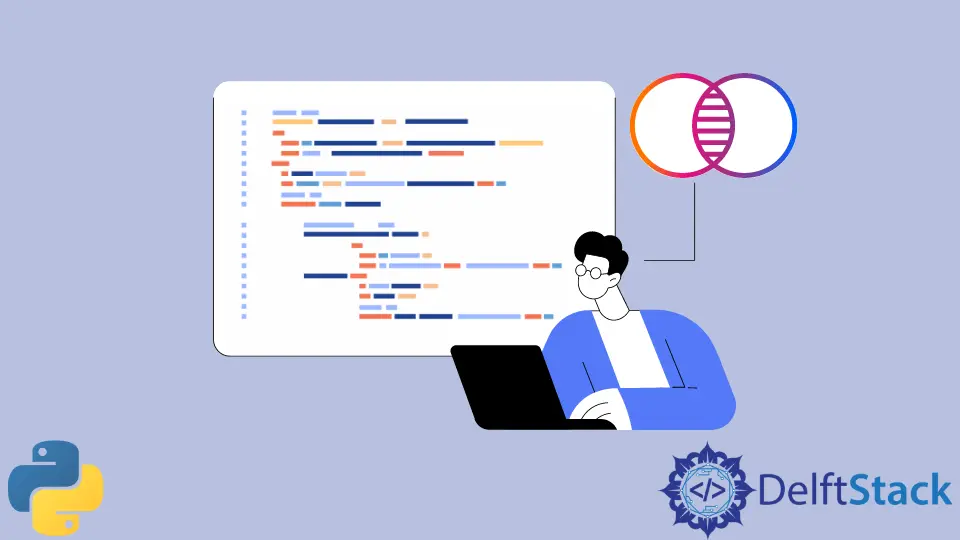
In this tutorial, we will discuss several methods to get the intersection between two lists in Python.
Intersection (or AND) is basically an operation specifically designed for sets
. It works by selecting the common elements in two sets. For exmaple,
setA = {1, 2, 3, 4, 5}
setB = {0, 2, 4, 6}
print(setA & setB)
Output:
{2, 4}
In the above example, setA
and setB
are two sets ,and the &
operator performs intersection operation on the sets.
By default, Python does not support performing direct intersection on lists. But with a little tweaking, we can perform intersection on lists as well.
Get List Intersection in Python With the &
Operator
The &
operator, as discussed above, cannot be used with lists. So, we have to change our lists to sets using set()
method.
list1 = [1, 2, 3, 4, 5]
list2 = [0, 2, 4, 6]
set1 = set(list1)
set2 = set(list2)
set3 = set1 & set2
list3 = list(set3)
print(list3)
Output:
[2, 4]
The set()
function converts a list to a set. The &
operation returns a set that contains all the common elements in both sets. As we know, we have to perform this operation on lists rather than on sets. So, we have to convert this set into a list using the list()
function.
Get List Intersection in Python With the intersection()
Method
The intersection()
method of the set
class is another way to perform intersection on sets in Python. Similar to the &
operator, it is also limited to just sets. But by converting the list1
into a set, we can also use intersection()
with list1
.
list1 = [1, 2, 3, 4, 5]
list2 = [0, 2, 4, 6]
set1 = set(list1)
set2 = set(list2)
set3 = set(list1).intersection(list2)
list3 = list(set3)
print(list3)
Output:
[2, 4]
The intersection()
method also returns a set of all the common elements. So, we have to convert it into a list using the list()
function.
Get List Intersection in Python With the List Comprehensions
Both methods discussed above are designed to work with sets rather than lists. As we all know that a set cannot have recurring values, and its elements are unsorted, these functions don’t work well if we have recurring values in our lists or we want to retain the order in our lists.
The drawbacks of the previous two methods are demonstrated in this coding example.
list1 = [1, 2, 3, 2, 4, 5]
list2 = [0, 2, 2, 4, 6]
setintersection = list(set(list1) & set(list2))
intersectionmethod = list(set(list1).intersection(list2))
print("The result of set intersection :")
print(setintersection)
print("The result of intersection() method :")
print(intersectionmethod)
Output:
The result of set intersection :
[2, 4]
The result of intersection() method :
[2, 4]
As shown above, we expect [2, 2, 4]
as the right result but only get [2, 4]
.
The list comprehension is another way of performing the AND
operation on lists in Python. It can handle recurring values and also keep the order of elements, which is not kept in the above two methods.
# Solution 2 Using list comprehensions
list1 = [1, 2, 3, 2, 4, 5]
list2 = [0, 2, 2, 4, 6]
list3 = [x for x in list1 if x in list2]
# all the x values that are in A, if the X value is in B
print(list3)
Output:
[2, 2, 4]
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python