How to Use If-Elif-Else in List Comprehension in Python
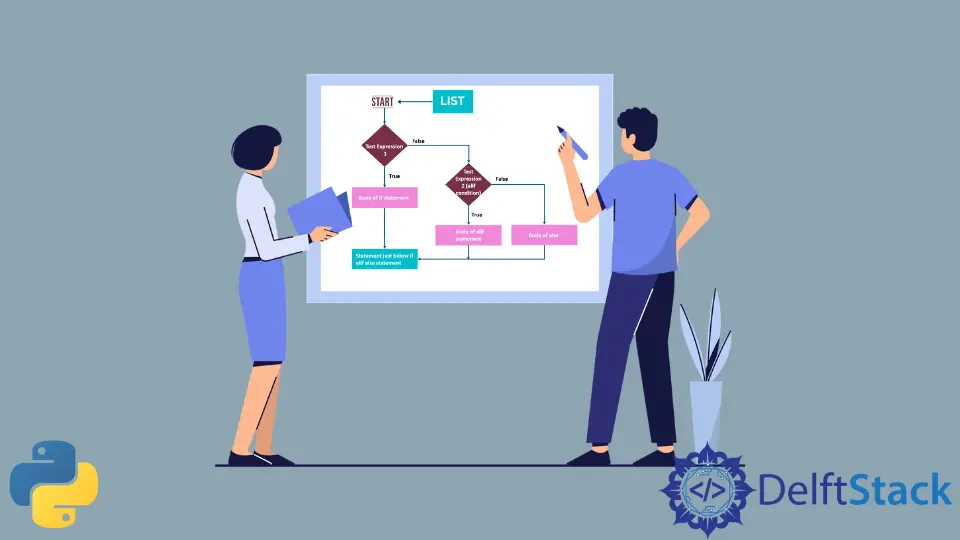
We will learn with this explanation about what chaining is and, in Python, how to apply if-elif-else
or chaining in the list comprehension.
Use if-elif-else
in List Comprehension With the Help of Chaining in Python
An interesting question in the Python community is how to use if-elif-else
in a list comprehension, which involves the one line for
loop. We already know how to use if-else
in list comprehension, but we should know that we can not write every code in list comprehension while writing Python script.
Implement Chaining in List Comprehension in Python
There is no concept of if-elif-else
inside the list comprehension, but we can use chaining, which will be an alternative. First of all, we will need to know what chaining is.
Chaining is used when we have multiple conditions, such as:
if condition1:
code1
elif condition2:
code2
elif condition3:
code3
else condition4:
code4
The above code explains that the workflow of chaining does not do anything. This shows that if the first condition does not meet true, the second condition is executed.
The else
block is executed if any condition does not meet true.
Let’s convert these if-elif-else
into chaining, but it will work when every condition contains a single statement. To create chaining, we will start with code1
and check if the instruction meets true, then the control will check the second chaining that is else code2 if condition2
.
code1 if condition1 else code2 if condition2 else code3 if consition3 else condition4
If else code2 if condition2
meets true, then the control goes on third chaining, else code3 if consition3
. And, if it also meets true, then the control goes on to the fourth chaining, which would be else condition4
.
This is called chaining and is how it works.
List Comprehension With if-elif-else
in Python
Let’s take an example, jump into the Python script, and look at how to apply this scenario for chaining.
Suppose we have a program that stores the status if any condition meets true. In this program, we will iterate over a list, check several conditions, and append the result in a blank list called status
.
list = [1, 0, 2, -3, 11, 0, -1]
status = []
for i in list:
if i > 0:
status.append("Positive")
elif i < 0:
status.append("Negative")
else:
status.append("Zero")
print(status)
Output:
['Positive', 'Zero', 'Positive', 'Negative', 'Positive', 'Zero', 'Negative']
We can convert the above code into the list comprehension, which will be one line of code. Remember that list comprehension does not work with every piece of code and does not allow an elif
block or clause, but we will use elif
by chaining.
To create a list comprehension, we will create an empty list and place three chains. We also have looked above- in the pseudo-code how to work chaining.
List comprehension does not allow the append()
function, but it appends the element itself. We can place more than several conditions by using chaining.
print(["Positive" if i > 0 else "Negative" if i < 0 else "zero" for i in list])
Output:
['Positive', 'zero', 'Positive', 'Negative', 'Positive', 'zero', 'Negative']
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python