How to Find the Index of the Maximum Element in a List in Python
-
Use the
max()
Function andfor
Loop to Find the Index of the Maximum Element in a List in Python -
Use the
max()
andindex()
Functions to Find the Index of the Maximum Element in a List in Python -
Use the
max()
andenumerate()
Functions to Find the Index of the Maximum Element in a List in Python -
Use the
max()
andoperator.itemgetter()
Functions to Find the Index of the Maximum Element in a List in Python -
Use the
max()
and__getitem__()
Functions to Find the Index of the Maximum Element in a List in Python -
Use the
numpy.argmax()
Function to Find the Index of the Maximum Element in a List in Python - Conclusion
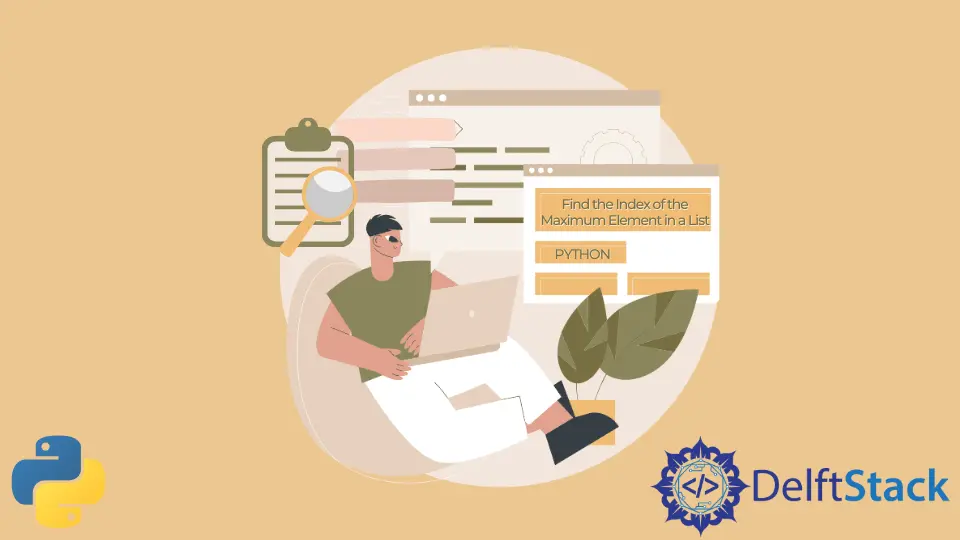
We can store and access elements efficiently using lists; each can be accessed using its index. The list class has different functions to make it easy to manipulate a list.
This article will demonstrate how to find the index of the maximum element in a list in Python.
Use the max()
Function and for
Loop to Find the Index of the Maximum Element in a List in Python
We can use the max()
function to get the maximum element value in a list. We can also find the index of this element with the for
loop.
We will iterate through the list and compare each element. When it matches a given element, we will return the value of the counter variable in the loop and break out with the break
keyword.
Example:
lst = [1, 4, 8, 9, -1]
m = max(lst)
for i in range(len(lst)):
if lst[i] == m:
print(i)
break
Output:
3
Use the max()
and index()
Functions to Find the Index of the Maximum Element in a List in Python
We also have the index()
function in Python that returns the index of the given element if it is present in the list. If not, then it throws a ValueError
.
To get the index of the maximum element in a list, we will first find the maximum element with the max()
function and find its index with the index()
function.
Example:
lst = [1, 4, 8, 9, -1]
m = max(lst)
print(lst.index(m))
Output:
3
Use the max()
and enumerate()
Functions to Find the Index of the Maximum Element in a List in Python
In Python, the enumerate
variable attaches a counter variable to every element of the iterable. It returns an enumerate
type object, which is iterable.
We can create a new list of the previous elements with their counter variables as tuples in a list using list comprehension. Then, we can employ the max()
function to return the maximum element with its index.
Example:
lst = [1, 4, 8, 9, -1]
a, i = max((a, i) for (i, a) in enumerate(lst))
print(i)
Output:
3
Use the max()
and operator.itemgetter()
Functions to Find the Index of the Maximum Element in a List in Python
The itemgetter()
function from the operator
module returns a callable object which can be utilized to get an element from its operand (the operand being iterable).
The max()
function has a key
parameter that can determine the criteria for the comparison on selecting the maximum element. The max()
function will directly return the index of the maximum element with the itemgetter()
function as the value for this parameter.
Example:
import operator
lst = [1, 4, 8, 9, -1]
i = max(enumerate(lst), key=operator.itemgetter(1))[0]
print(i)
Output:
3
This method finds the maximum element and its index in one line; therefore, it is considered a faster approach than the previous methods.
Use the max()
and __getitem__()
Functions to Find the Index of the Maximum Element in a List in Python
The magic function __getitem__()
is called internally by the operator.itemgetter()
method. It can be used in place of the operator.itemgetter()
function as utilized in the previous method.
This magic function can be used directly to avoid importing the operator module making the code run faster.
Example:
lst = [1, 4, 8, 9, -1]
i = max(range(len(lst)), key=lst.__getitem__)
print(i)
Output:
3
Use the numpy.argmax()
Function to Find the Index of the Maximum Element in a List in Python
To find the index of the maximum element in an array, we use the numpy.argmax()
function. This function works with a list and can return the index of the maximum element.
Example:
import numpy as np
lst = [1, 4, 8, 9, -1]
i = np.argmax(lst)
print(i)
Output:
3
Conclusion
In conclusion, several methods can be used to find the index of the maximum element in a list.
All the methods, except the numpy.argmax()
function, uses the max()
function. The methods involving enumerate()
and itemgetter()
are faster and more efficient.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python