Python numpy.argmax()
-
Syntax of
numpy.argmax()
: -
Example Codes:
numpy.argmax()
Method to Find Indices of Largest Values in an Array -
Example Codes: Set
axis
Parameter innumpy.argmax()
Method to Find Indices of Largest Values in an Array -
Example Codes: Set
out
Parameter innumpy.argmax()
Method to Find Indices of Largest Values in an Array
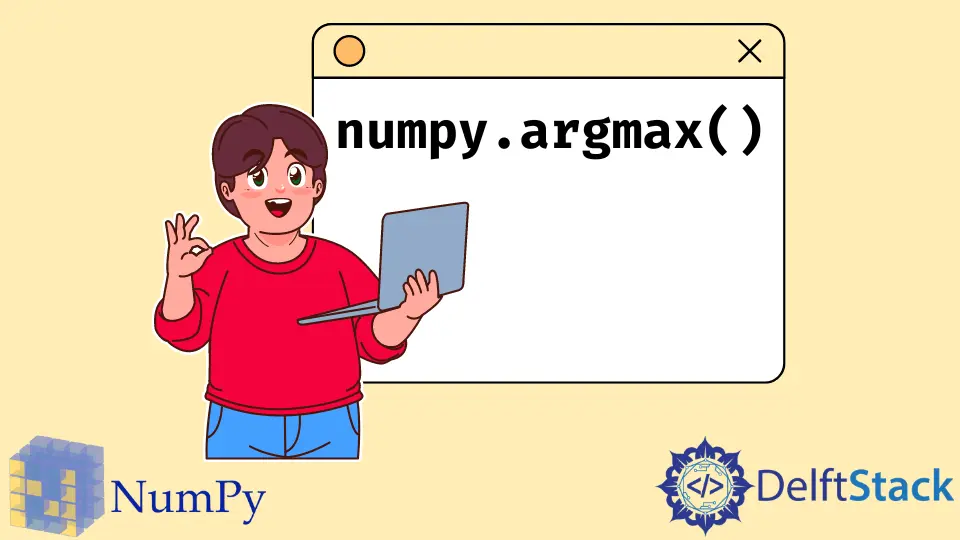
Python Numpy numpy.argmax()
returns the indices of values with the highest values in the given NumPy array.
Syntax of numpy.argmax()
:
numpy.argmax(a, axis=None, out=None)
Parameters
a |
Array or Object that can be converted to array in which we need to find indices of highest values. |
axis |
find index of the greatest value along the row (axis=0) or column (axis=1). By default, the index of the greatest value is found by flattening the array. |
out |
placeholder for the result of the np.argmax method. It must be of the appropriate size to hold the result. |
Return
An array of indices of elements with the highest value in the entire array.
Example Codes: numpy.argmax()
Method to Find Indices of Largest Values in an Array
Find the Index of the Highest Value in a 1-D Array
import numpy as np
a=np.array([2,6,1,5])
print("Array:")
print(a)
req_index=np.argmax(a)
print("\nIndex with the largest value:")
print(req_index)
print("\nThe largest value in the array:")
print(a[req_index])
Output:
Array:
[2 6 1 5]
Index with the largest value:
1
The largest value in the array:
6
It gives the index of the element with the largest value in the given input array.
We can also find the largest value in the array using the index returned from the np.argmax()
method.
If we have two largest values in the array, the method returns the index of the largest element which appears first in the array.
import numpy as np
a=np.array([2,6,1,6])
print("Array:")
print(a)
req_index=np.argmax(a)
print("\nIndex with the largest value:")
print(req_index)
print("\nThe largest value in the array:")
print(a[req_index])
Output:
Array:
[2 6 1 5]
Index with the largest value:
1
The largest value in the array:
6
Here, 6
is the highest value which occurs twice in the array, but the np.argmax()
returns the index of 6
at index 1
since it comes first in the array.
Find the Index of the Highest Value in a 2-D Array
import numpy as np
a=np.array([[2,1,6],
[7,4,5]])
print("Array:")
print(a)
req_index=np.argmax(a)
print("\nIndex with the largest value:")
print(req_index)
Output:
Array:
[[2 1 6]
[7 4 5]]
Index with the largest value:
3
Here, as the axis
parameter is not given, the array gets flattened and the index of the largest element in the flattened array is returned.
Example Codes: Set axis
Parameter in numpy.argmax()
Method to Find Indices of Largest Values in an Array
Find Indices of the Highest Element Along the Column Axis
import numpy as np
a=np.array([[2,1,6],
[7,4,5]])
print("Array:")
print(a)
req_index=np.argmax(a,axis=0)
print("\nIndices with the largest value along column axis:")
print(req_index)
Output:
Array:
[[2 1 6]
[7 4 5]]
Index with the largest value:
[1 1 0]
If we set axis=0
, it gives indices of the highest value along each column. Here the first column has the highest value at index 1
, the second column has the highest value at index 1
and the third column has the highest value at index 0
.
Find Indices of the Highest Elements Along the Row Axis
import numpy as np
a=np.array([[2,1,6],
[7,4,5]])
print("Array:")
print(a)
req_index=np.argmax(a,axis=1)
print("\nIndices with the largest value along row axis:")
print(req_index)
Output:
Array:
[[2 1 6]
[7 4 5]]
Indices with the largest value along the row axis:
[2 0]
If we set axis=1
, it gives indices of the highest values along each row.
Example Codes: Set out
Parameter in numpy.argmax()
Method to Find Indices of Largest Values in an Array
import numpy as np
a=np.array([[2,1,6],
[7,4,5]])
req_index=np.array(0)
print("Array:")
print(a)
np.argmax(a,out=req_index)
print("\nIndex with the largest value:")
print(req_index)
Output:
Array:
[[2 1 6]
[7 4 5]]
Index with the largest value:
3
Here, the variable req_index
acts as a placeholder for the required value of the index and we must make sure that the size of output must be equal to req_index
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn