Python numpy.argmax()
Suraj Joshi
2023年1月30日
-
numpy.argmax()
的语法 -
示例代码:
numpy.argmax()
寻找数组中最大值的索引的方法 -
示例代码: 在
numpy.argmax()
方法中设置axis
参数以查找数组中最大值的索引 -
示例代码:在
numpy.argmax()
方法中设置out
参数查找数组中最大值的索引
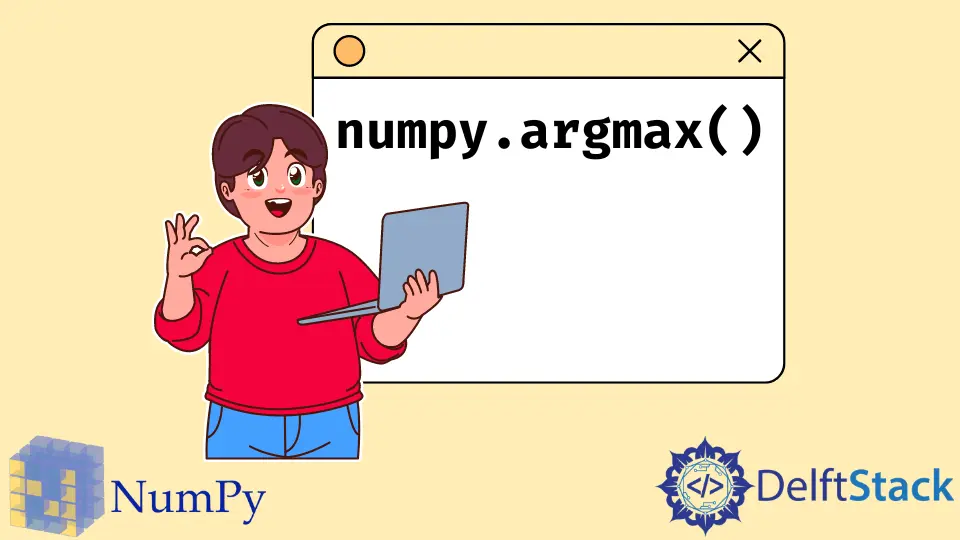
Python Numpy numpy.argmax()
返回给定 NumPy 数组中具有最大值的索引。
numpy.argmax()
的语法
numpy.argmax(a, axis=None, out=None)
参数
a |
可以转换为数组的数组或对象,我们需要在其中找到最高值的索引。 |
axis |
沿着行(axis=0 )或列(axis=1 )查找最大值的索引。默认情况下,通过对数组进行展平可以找到最大值的索引。 |
out |
np.argmax 方法结果的占位符。它必须有适当的大小以容纳结果。 |
返回值
一个数组,包含整个数组中数值最大的元素的索引。
示例代码: numpy.argmax()
寻找数组中最大值的索引的方法
找出一维数组中最高值的索引
import numpy as np
a=np.array([2,6,1,5])
print("Array:")
print(a)
req_index=np.argmax(a)
print("\nIndex with the largest value:")
print(req_index)
print("\nThe largest value in the array:")
print(a[req_index])
输出:
Array:
[2 6 1 5]
Index with the largest value:
1
The largest value in the array:
6
它给出了给定输入数组中值最大的元素的索引。
我们也可以使用 np.argmax()
方法返回的索引找到数组中的最大值。
如果我们在数组中有两个最大的值,该方法返回数组中最先出现的最大元素的索引。
import numpy as np
a=np.array([2,6,1,6])
print("Array:")
print(a)
req_index=np.argmax(a)
print("\nIndex with the largest value:")
print(req_index)
print("\nThe largest value in the array:")
print(a[req_index])
输出:
Array:
[2 6 1 5]
Index with the largest value:
1
The largest value in the array:
6
这里,6
是数组中出现两次的最高值,但是 np.argmax()
方法返回 6
的索引,在索引 1
处,因为它在数组中排在第一位。
寻找二维数组中最高值的索引
import numpy as np
a=np.array([[2,1,6],
[7,4,5]])
print("Array:")
print(a)
req_index=np.argmax(a)
print("\nIndex with the largest value:")
print(req_index)
输出:
Array:
[[2 1 6]
[7 4 5]]
Index with the largest value:
3
这里,由于没有给出 axis
参数,数组被扁平化,并返回扁平化数组中最大元素的索引。
示例代码: 在 numpy.argmax()
方法中设置 axis
参数以查找数组中最大值的索引
沿着列轴寻找最高元素的索引
import numpy as np
a=np.array([[2,1,6],
[7,4,5]])
print("Array:")
print(a)
req_index=np.argmax(a,axis=0)
print("\nIndices with the largest value along column axis:")
print(req_index)
输出:
Array:
[[2 1 6]
[7 4 5]]
Index with the largest value:
[1 1 0]
如果我们设置 axis=0
,它给出了每列最高值的索引。这里第一列的最高值在索引 1
,第二列的最高值在索引 1
,第三列的最高值在索引 0
。
沿着行轴查找最高元素的索引
import numpy as np
a=np.array([[2,1,6],
[7,4,5]])
print("Array:")
print(a)
req_index=np.argmax(a,axis=1)
print("\nIndices with the largest value along row axis:")
print(req_index)
输出:
Array:
[[2 1 6]
[7 4 5]]
Indices with the largest value along the row axis:
[2 0]
如果我们设置 axis=1
,它给出了每行最高值的索引。
示例代码:在 numpy.argmax()
方法中设置 out
参数查找数组中最大值的索引
import numpy as np
a=np.array([[2,1,6],
[7,4,5]])
req_index=np.array(0)
print("Array:")
print(a)
np.argmax(a,out=req_index)
print("\nIndex with the largest value:")
print(req_index)
输出:
Array:
[[2 1 6]
[7 4 5]]
Index with the largest value:
3
在这里,变量 req_index
作为索引所需值的占位符,我们必须确保输出的大小必须等于 req_index
。
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn