How to Import Text File in Python
-
Use the
open()
Function to Import a File in Python -
Use the
numpy.genfromtxt()
Function to Import a File in Python
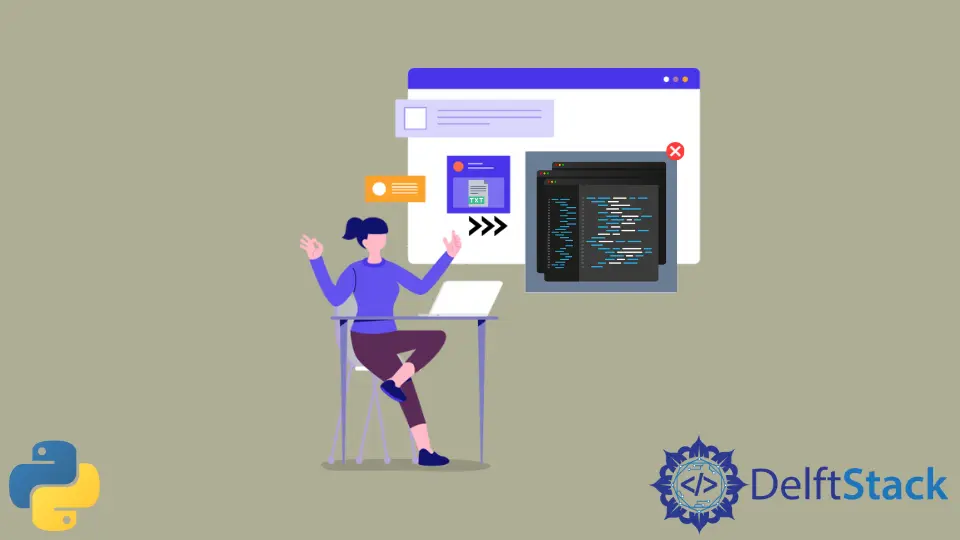
Like in the other popular programming languages like C, C++, etc., Python supports file handling. It allows the programmers to deal with files and essentially perform some basic operations like reading, writing, and some other file handling options to operate on files.
There is no requirement for importing external libraries to read and write files in Python. Python provides built-in functions for reading, writing, and creating files.
The text or binary files can be opened for reading, writing, and modifying, but they cannot be imported. The word import
might be a little misleading here, so we will refer to it as opening a file in the whole article.
Use the open()
Function to Import a File in Python
The open()
function, as its name suggests, is used to open a text or binary file in Python. It is a built-in function, and therefore, it can be used without importing any module.
The syntax of the open()
function is below.
open(path_to_file, mode)
The open()
function has a couple of parameters, but the most important ones are the first two, namely path_to_file
and mode
.
The path_to_file
mode specifies the path or name of the file, while the mode
parameter specifies the mode in which we want to open the file.
The following code uses the open()
function to open a text file in Python.
f = open("file1.txt", "r")
This line of code opens the file named file.txt
in reading mode.
The opened file will remain open until it is manually closed by the programmer using the close()
function. Closing the file that is no longer in use is essential as if it is not closed, the file might get corrupted, or the whole program might crash.
The following code uses the close()
function to close the file in Python.
f.close()
Use the numpy.genfromtxt()
Function to Import a File in Python
The NumPy
library needs to be imported to use the genfromtxt()
function.
NumPy
, an abbreviation for Numerical Python, is a library used in Python that consists of multidimensional array objects and an assembly of methods for processing these arrays. Logical and mathematical operations can be performed on arrays using NumPy
.
The genfromtxt()
function is used to load data from a text file. It is especially helpful in dealing with numbers and comes in handy when some missing values need to handled as specified.
The following code uses the genfromtxt()
function to open a text file in Python.
import numpy as np
...
f = np.genfromtxt(fname="file1.txt")
import
gives access to other modules in the Python program. On the other hand, the files are either text or Binary, and they are not modules. Modules can be imported into the Python code, but the files can only be opened using the two commands mentioned in this article.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn