How to Import Class From Another File in Python
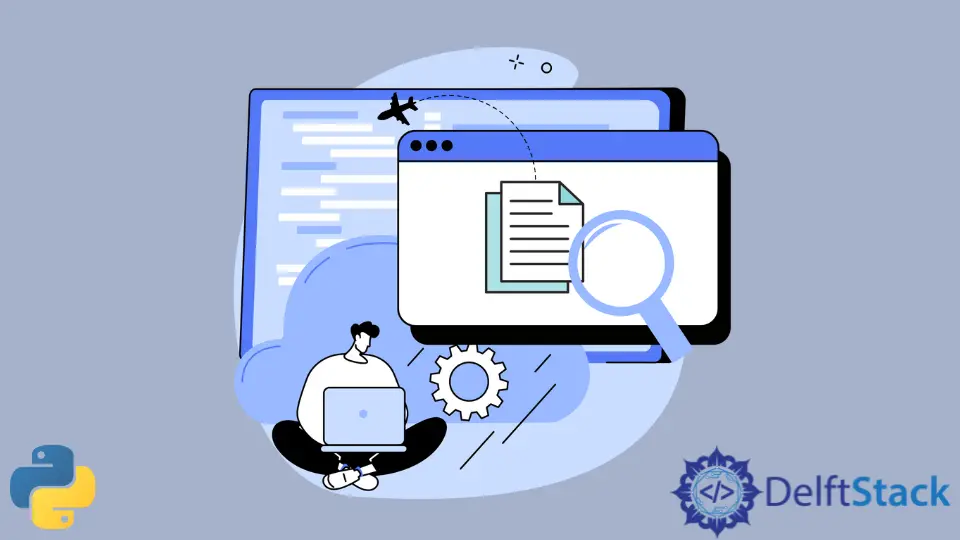
This tutorial will explain various methods to import a class from another file in Python. We need to import a class from another file when we want to use methods of another class in our code. It saves time as we do not have to implement the methods again and makes the code looks clean as we do not have to copy-paste the methods in the current project.
Import Class in Python
We frequently encounter situations in programming where we must repeat specific processes. It is time-consuming to write code for them every time.
We employ the concept of Object-Oriented Programming (OOPs) to avoid such scenarios in which we use the program frequently whenever we need it while working in Python.
This article will demonstrate how to import a class from another file in Python using different methods.
The OOPs in Python
Object-oriented programming (OOPs) is a programming model in Python that employs objects and classes. Its goal is to use programming to create real-world concepts like inheritance, polymorphisms, and encapsulation.
The primary idea behind OOPs is to combine data and the algorithms that operate with it into a single unit, even though no other parts of the program may access it.
Now, let us go through an example in which we will develop a function to perform some operations, and we will try to import it from that file to our new file.
First, let us create a new file, Numsum
, with a class performing some functions as shown below.
# python
class Operations:
def __init__(self):
self.sum_ = 0
def To_sum(self, a, b):
self.sum_ = a + b
return self.sum_
We will import this file into another file where we want to use the class and its functions. Both files should be located in the same folder to make them easier to import.
Once we have imported the file, we will use the class operations
and make a sum of 2 numbers, as shown below.
# python
from Numsum import Operations
obj = Operations()
Sum = obj.To_sum(4, 12)
print("Sum is :", Sum)
Output:
From the above example, we easily imported the class operations
and used its function to create a sum of 2 numbers.
Import Multiple Classes From Another File in Python
Now, we’ll look at how to import all of the classes from another file. It might be necessary to import all of the classes from other files.
We can read it using the command from file name import all
. This indicates that we have imported all the classes from the supplied file.
We use the from file name> import *
statement in this situation. This statement imports all classes from the file, as shown below.
# python
class Operations:
def __init__(self):
self.sum = 0
def To_sum(self, a, b):
self.sum = a + b
return self.sum
class showData:
def __init__(self):
self.DATA = ""
def show_data(self, INFO):
self.INFO = INFO
return self.INFO
Now we will import all the classes from our file and use them in a function. As shown below, we use *
instead of a class name to import all the classes from a file.
# python
from NumSum import *
obj1 = Operations()
print(obj1.To_sum(15, 16))
obj2 = showData()
print(obj2.show_data("Importing Multiple classes from NumSum"))
Output:
Import Class From Another Folder in Python
Now, suppose we want to import a class from a file located in another folder. Let’s understand it better with an example.
We will use the Operations
class in Numsum.py, located in another folder, i.e., NewFolder. We must also specify the path of NewFolder.
For that, we will use the sys
module. Take a look at the code below.
# python
from NewFolder.Numsum import Operations
import sys
sys.path.insert(1, "##")
obj = Operations()
print(obj.To_sum(15, 16))
Output:
As you can see, we can also import classes from a file placed in some other folder using the Python module sys
.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn