How to Import a File in Python
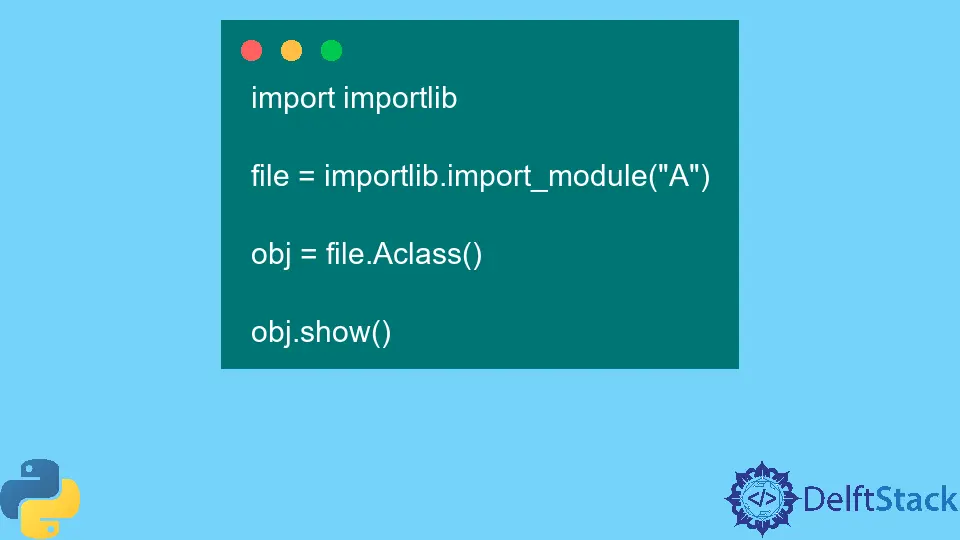
Importing files in Python is a fundamental skill for any developer. Whether you are working on a small script or a large application, knowing how to efficiently import files can enhance your productivity and code organization. In Python, there are three primary methods to import files: the import statement, the importlib module, and the from clause. Each of these methods has its unique use cases and advantages.
In this article, we will explore these methods in detail, providing clear examples and explanations to help you master file imports in Python. Let’s dive in!
Using the Import Statement
The most common way to import a file in Python is by using the import statement. This method allows you to bring in entire modules, making their functions, classes, and variables available for use in your script. The syntax is straightforward: you simply use the keyword import
followed by the module name.
Here’s a simple example:
import math
result = math.sqrt(16)
print(result)
Output:
4.0
In this example, we import the built-in math
module, which provides mathematical functions. By calling math.sqrt(16)
, we compute the square root of 16, which returns 4.0. The import statement is particularly useful when you need to access a wide range of functionalities from a module without having to specify each item individually.
One of the key advantages of using the import statement is its simplicity and readability. It’s easy to understand at a glance what modules are being used in your code. However, it does import the entire module, which may not be efficient if you only need a specific function. In such cases, you might consider using the from clause, which we will discuss next.
Utilizing the From Clause
The from clause is another powerful method for importing files in Python. This approach allows you to import specific functions or classes from a module, which can help to keep your namespace clean and improve code readability. The syntax involves using the from
keyword followed by the module name and the specific item you want to import.
Here’s how it works:
from math import sqrt
result = sqrt(25)
print(result)
Output:
5.0
In this example, we use the from clause to import only the sqrt
function from the math
module. This means we can call sqrt(25)
directly without needing to prefix it with math.
. This method is particularly beneficial when you only need a few items from a module, as it reduces the amount of code and can enhance performance.
The from clause also allows for better readability, especially in larger scripts. By importing only what you need, you can avoid potential name conflicts with other variables or functions in your code. However, it’s essential to be cautious when using this method, as importing multiple items with the same name from different modules can lead to confusion.
Exploring the Importlib Module
The importlib module is a more advanced way to import files in Python, providing a programmatic approach to module importing. This method is particularly useful for dynamic imports, where you may not know the module name until runtime. The importlib module allows for greater flexibility and control over the import process.
Here’s an example:
import importlib
math_module = importlib.import_module('math')
result = math_module.pow(3, 2)
print(result)
Output:
9.0
In this example, we use importlib.import_module()
to import the math
module dynamically. We then call math_module.pow(3, 2)
to compute 3 raised to the power of 2. This method is particularly useful in scenarios where you want to load modules based on user input or configuration files.
The importlib module is a powerful tool for developers looking to build more dynamic and flexible applications. It allows for better management of dependencies and can be particularly beneficial in larger projects where modules may be loaded conditionally. However, it’s essential to ensure that the modules you are importing exist and are accessible, as this method can lead to runtime errors if not handled correctly.
Conclusion
In conclusion, importing files in Python can be accomplished through various methods, each with its strengths and use cases. The import statement is perfect for straightforward imports, while the from clause allows for more selective access to module contents. For dynamic scenarios, the importlib module offers unparalleled flexibility. Understanding these methods will not only enhance your coding skills but also improve the efficiency and organization of your projects. So, the next time you find yourself needing to import a file in Python, you’ll know exactly which method to choose!
FAQ
-
What is the difference between import and from in Python?
The import statement brings in the entire module, while the from clause allows you to import specific functions or classes from a module. -
When should I use importlib?
Use importlib when you need to import modules dynamically at runtime, based on conditions or user input.
-
Can I import multiple functions using the from clause?
Yes, you can import multiple functions by separating them with commas, like this:from module import func1, func2
. -
Is there a performance difference between import and from?
Generally, using from to import specific functions is more efficient since it only loads what you need, while import loads the entire module. -
Can I use importlib to import built-in modules?
Yes, you can use importlib to import built-in modules just like any other module in Python.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn