How to Import Class From Subdirectories in Python
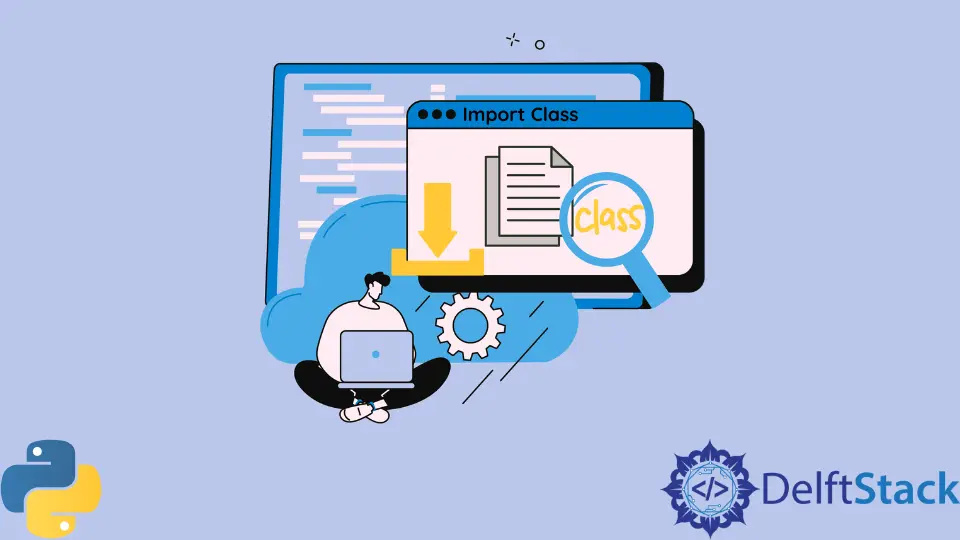
In this tutorial, we will discuss methods to import classes from sub-directories in Python.
Import Classes From Sub-Directories With import
Statement in Python3
In Python 3.x, importing classes from the current or any sub-directories is very easy. We will use the following directory structure in this tutorial.
Main/
main.py
A.py
B/
B.py
The files A.py
and B.py
contain two classes, Aclass
and Bclass
, which we will import to the main.py
class. The code of both A.py
and B.py
are shown below.
A.py
file:
class Aclass:
a = 5
def show(this):
print("Hello! this is class A")
B.py
file:
class Bclass:
b = 5
def show(this):
print("Hello! this is class B")
The import
statement imports the Aclass
and the Bclass
in main.py
. The following code example shows us how to import classes from sub-directories with the import
statement in Python.
from A import Aclass
from B.B import Bclass
var1 = Aclass()
var2 = Bclass()
var1.show()
var2.show()
Output:
Hello! this is class A
Hello! this is class B
In the above code, we import both Aclass
and Bclass
in the main.py
file with the import
statement. For the files in the same directory, we have to use the following notation.
from filename import classname
The filename
is the name of the file, and the classname
is the name of the class to be imported. For the files in the sub-directory, we have to follow the following notation.
from dirname.filename import classname
The dirname
is the name of the directory in which the file is located, the filename
is the name of the file, and the classname
is the class’s name to be imported. For the files in sub-directories or sub-sub-directories, we have to add another .subdirname
as shown below.
from dirname.subdirname.filename import classname
The dirname
is the name of the directory, subdirname
is the name of the sub-directory containing the file, the filename
is the name of the file, and the classname
is the name of the class to be imported.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn