How to Remove One or Multiple Keys From a Dictionary in Python
-
Remove Keys From a Dictionary Using a
for
Loop - Delete Keys From a Dictionary Using Dictionary Comprehension
-
Remove Keys From a Dictionary Using the
pop()
Method -
Delete Keys From a Dictionary Using the
del
Statement - Conclusion
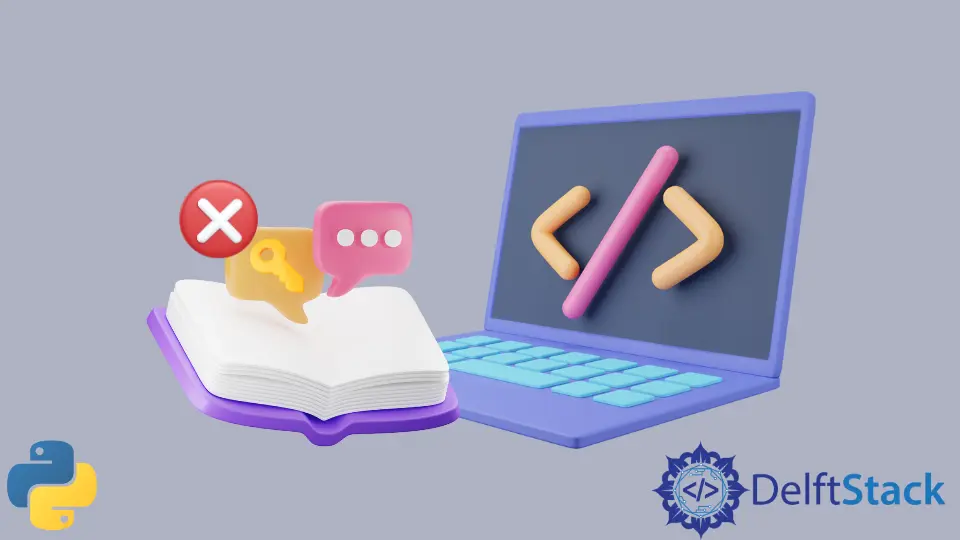
A dictionary is used in Python to store key-value pairs. While programming, we sometimes need to remove some key-value pairs from the dictionary. For that, we can simply remove the key from the dictionary, and the associated value gets deleted automatically. This article will discuss the various ways to remove one or multiple keys from a dictionary in Python.
Remove Keys From a Dictionary Using a for
Loop
The most naive way to remove one or multiple keys from a dictionary is to create a new dictionary leaving out the keys that need to be deleted. For this, we will first create an empty dictionary. Then, we will use a for
loop to traverse over each key in the existing dictionary. Whenever we find the key that needs to be removed, we will not include the key-value pair in the new dictionary. Otherwise, we will put the key-value pairs in the new dictionary.
For instance, suppose that we have the following dictionary.
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
If we want to remove the key Author
from the dictionary, we can do this using a for
loop as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
newDict = dict()
print("The original dictionary is:")
pprint.pprint(myDict)
for key in myDict:
if key != "Author":
newDict[key] = myDict[key]
print("The updated dictionary is:")
pprint.pprint(newDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
To remove multiple keys using a for
loop, we will create a list named keys_to_delete
that consists of keys that need to be removed. While traversing the original dictionary, we will omit all the key-value pairs whose keys are present in keys_to_delete
. In this way, we can remove the key from the dictionary as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
newDict = dict()
print("The original dictionary is:")
pprint.pprint(myDict)
keys_to_delete = ["Author", "Topic"]
for key in myDict:
if key not in keys_to_delete:
newDict[key] = myDict[key]
print("The updated dictionary is:")
pprint.pprint(newDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Keyword': 'Remove key from dictionary in python',
'Website': 'DelftStack.com'}
Delete Keys From a Dictionary Using Dictionary Comprehension
Instead of using a for
loop, we can use dictionary comprehension to create a new dictionary after removing the keys from an existing dictionary. The syntax for dictionary comprehension is as follows.
newDict = {key: value for (key, value) in iterable_obejct if condition}
Here,
iterable
can be any object from which we can create key-value pairs. In our case, it will be the existing dictionary from which we have to remove the keys.- The
condition
here will be that thekey
should not be thekey
that needs to be removed from the existing dictionary.
To remove a key from a given dictionary, we can use dictionary comprehension as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
newDict = dict()
print("The original dictionary is:")
pprint.pprint(myDict)
newDict = {key: value for (key, value) in myDict.items() if key != "Author"}
print("The updated dictionary is:")
pprint.pprint(newDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
We can also remove multiple keys at once. For that, we will declare a list named keys_to_delete
with all the keys that need to be deleted. After that, we will mention in the condition that the key to be included in the new dictionary should not be present in keys_to_delete
as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
newDict = dict()
print("The original dictionary is:")
pprint.pprint(myDict)
keys_to_delete = ["Author", "Topic"]
newDict = {key: value for (key, value) in myDict.items() if key not in keys_to_delete}
print("The updated dictionary is:")
pprint.pprint(newDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Keyword': 'Remove key from dictionary in python',
'Website': 'DelftStack.com'}
Remove Keys From a Dictionary Using the pop()
Method
Python also provides us with an inbuilt pop()
method to remove a key from a given dictionary. When invoked on a dictionary, the pop()
method takes the key that needs to be removed as its first argument and a default value as its second input argument. The syntax for the pop()
method is as follows.
myDict.pop(key, default_value)
Here,
myDict
is the existing dictionary.key
is the key that needs to be deleted.default_value
is the value that thepop()
method returns when thekey
is not present inmyDict
.
If the key is present in myDict
, the pop()
method removes the key from the dictionary as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
newDict = dict()
print("The original dictionary is:")
pprint.pprint(myDict)
myDict.pop("Author")
print("The updated dictionary is:")
pprint.pprint(myDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
If the key to be removed is not present in myDict
, the pop()
method returns the default value that we pass as the second input argument.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
newDict = dict()
print("The original dictionary is:")
pprint.pprint(myDict)
output = myDict.pop("Class", 10)
print("The output from pop method is", output)
print("The updated dictionary is:")
pprint.pprint(myDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
The output from pop method is 10
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
In case the key is not present in myDict
and we do not pass the default value, the program will raise the KeyError
exception as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
newDict = dict()
print("The original dictionary is:")
pprint.pprint(myDict)
output = myDict.pop("Class")
print("The output from pop method is", output)
print("The updated dictionary is:")
pprint.pprint(myDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 13, in <module>
output = myDict.pop("Class")
KeyError: 'Class'
We can also remove multiple keys from the given dictionary using the pop()
method. For this, we will create a list of keys that we need to delete. After that, we will use a for
loop to remove the keys one by one as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
print("The original dictionary is:")
pprint.pprint(myDict)
keys_to_delete = ["Author", "Topic"]
for key in keys_to_delete:
myDict.pop(key)
print("The updated dictionary is:")
pprint.pprint(myDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Keyword': 'Remove key from dictionary in python',
'Website': 'DelftStack.com'}
Delete Keys From a Dictionary Using the del
Statement
The del
statement can be used to delete an object in python using the following syntax.
del object_name
Here, object_name
is the name of the object that needs to be deleted.
We can also use it to remove a key from a dictionary. For this, we will delete the entire key value-pair as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
newDict = dict()
print("The original dictionary is:")
pprint.pprint(myDict)
del myDict["Author"]
print("The updated dictionary is:")
pprint.pprint(myDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
We can also remove multiple keys from a dictionary using a for
loop and the del
statement as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
print("The original dictionary is:")
pprint.pprint(myDict)
keys_to_delete = ["Author", "Topic"]
for key in keys_to_delete:
del myDict[key]
print("The updated dictionary is:")
pprint.pprint(myDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Keyword': 'Remove key from dictionary in python',
'Website': 'DelftStack.com'}
Here, if the key is present in the dictionary, the del
statement simply removes the key from the dictionary. Otherwise, it raises a KeyError
exception as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
newDict = dict()
print("The original dictionary is:")
pprint.pprint(myDict)
del myDict["Class"]
print("The updated dictionary is:")
pprint.pprint(myDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 13, in <module>
del myDict["Class"]
KeyError: 'Class'
You can avoid the KeyError
exception using a try-except
block. Here, we will execute the del
statement in the try
block and handle the exception in the except
block.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
print("The original dictionary is:")
pprint.pprint(myDict)
keys_to_delete = ["Author", "Topic"]
key = "Class"
try:
del myDict[key]
except KeyError:
print("{} not present as key in the dictionary.".format(key))
print("The updated dictionary is:")
pprint.pprint(myDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
Class not present as key in the dictionary.
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
Alternatively, you can use the if-else
statement to check if the key is present in the dictionary before executing the del
statement as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
print("The original dictionary is:")
pprint.pprint(myDict)
keys_to_delete = ["Author", "Topic"]
key = "Class"
if key in myDict:
del myDict[key]
else:
print("{} not present as key in the dictionary.".format(key))
print("The updated dictionary is:")
pprint.pprint(myDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
Class not present as key in the dictionary.
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
Here, we have made sure that the key is present in the dictionary before we execute the del
statement. This helps us in avoiding the KeyError
exception. But, we have to check each key in order to remove the key from the dictionary. For a single key, either the if-else
statement or the try-except
block works fine. On the contrary, if we have to remove multiple keys from a dictionary, then only one of them will work efficiently based on the situation. We can choose the appropriate approach if we have an idea about the keys that we have to remove from the dictionary.
If we have to remove multiple keys in which most of the keys are supposed to be present in the dictionary, we will remove the keys using the del
statement and the try-except
block as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
print("The original dictionary is:")
pprint.pprint(myDict)
keys_to_delete = ["Author", "Topic", "Class"]
for key in keys_to_delete:
try:
del myDict[key]
except KeyError:
print("{} not present as key in the dictionary".format(key))
print("The updated dictionary is:")
pprint.pprint(myDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
Class not present as key in the dictionary
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Keyword': 'Remove key from dictionary in python',
'Website': 'DelftStack.com'}
This approach is more efficient compared to the if-else
statement as we do not need to check for the presence of each key. Also, we have assumed that most of the keys that have to be deleted are already present in the dictionary. Therefore, the program will also raise the KeyError
exception very few times, leading to faster execution of the program.
On the other hand, if we have to remove multiple keys from a dictionary and we know that most of the keys might not be present in the dictionary, we will remove the keys using the if-else
statement and the del
statement as follows.
import pprint
myDict = {
"Article": "Remove One or Multiple Keys From a Dictionary in Python",
"Topic": "Python Dictionary",
"Keyword": "Remove key from dictionary in python",
"Website": "DelftStack.com",
"Author": "Aditya Raj",
}
print("The original dictionary is:")
pprint.pprint(myDict)
keys_to_delete = ["Author", "Topic", "Class"]
for key in keys_to_delete:
if key in myDict:
del myDict[key]
else:
print("{} not present as key in the dictionary".format(key))
print("The updated dictionary is:")
pprint.pprint(myDict)
Output:
The original dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Author': 'Aditya Raj',
'Keyword': 'Remove key from dictionary in python',
'Topic': 'Python Dictionary',
'Website': 'DelftStack.com'}
Class not present as key in the dictionary
The updated dictionary is:
{'Article': 'Remove One or Multiple Keys From a Dictionary in Python',
'Keyword': 'Remove key from dictionary in python',
'Website': 'DelftStack.com'}
This choice depends on the reason that handling an exception is costlier than checking whether the key is present in the dictionary. So, if we know that most of the keys are not present in the dictionary, we will use the if-else
statement with the del
statement.
Conclusion
In this article, we learned four ways to remove one or multiple keys from a dictionary in Python. If you have to choose any of the discussed approaches, you can choose the for
loop or dictionary comprehension approach to remove a key from a given dictionary. If allowed to modify the existing dictionary, it is best to use the pop()
method with a default value. Otherwise, you can also use the approach using the del
statement to remove the keys from a dictionary. But, you need to make sure that KeyError
should not occur in this case using the if-else
statements or try-except
block.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub