如何在 Python 中從字典中刪除一個鍵
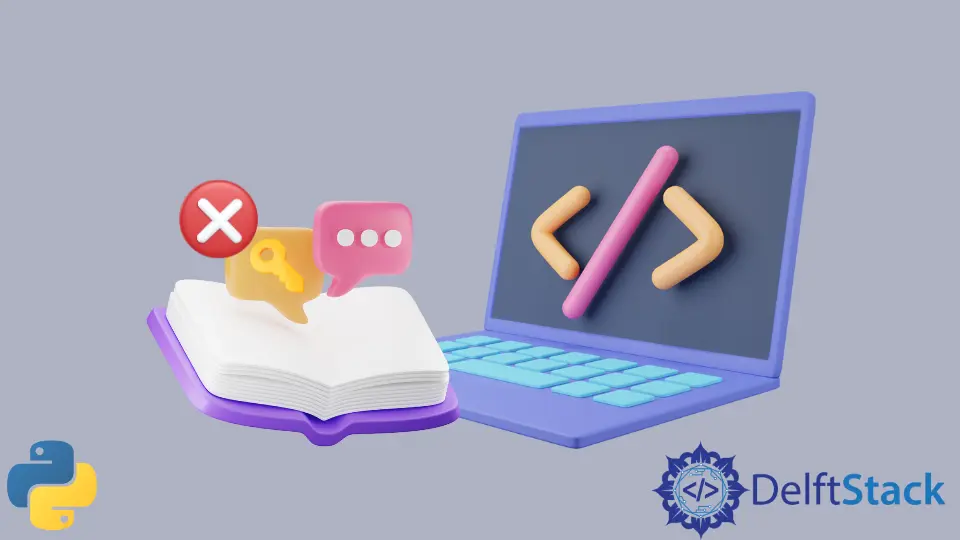
本文將介紹在 Python 中從字典中刪除鍵的方法,如 pop()
函式和 del
關鍵字。
Python 使用 pop()
函式從字典中刪除關鍵字
我們可以使用內建的 Python 函式 pop()
從字典中刪除一個鍵。這個方法的正確語法為:
mydict.pop(key[, default])
這個函式的詳細內容如下。
引數 | 說明 | |
---|---|---|
key |
強制 | 它是我們要從字典中刪除的鍵。如果鍵在字典中不存在,它返回預設 值。如果預設值沒有被傳遞,它將引發一個錯誤。 |
這個函式返回指定的鍵,同時從字典中刪除它,如果它存在的話。否則,它返回預設值。
下面的程式展示了我們如何在 Python 中使用這個函式從一個字典中刪除一個鍵。
mydict = {"1": "Rose", "2": "Jasmine", "3": "Lili", "4": "Hibiscus"}
print(mydict.pop("2", None))
print(mydict)
輸出:
Jasmine
{'1': 'Rose', '3': 'Lili', '4': 'Hibiscus'}
函式已經刪除了鍵 2
,它的值是 Jasmine
。
現在如果我們嘗試刪除一個不存在的鍵,那麼函式將給出以下輸出。
mydict = {"1": "Rose", "2": "Jasmine", "3": "Lili", "4": "Hibiscus"}
print(mydict.pop("5", None))
輸出:
None
該函式返回了預設值。
Python 使用 del
關鍵字從字典中刪除鍵
我們也可以使用 del
關鍵字來從 Python 中的字典中刪除一個鍵。使用這個關鍵字的正確語法如下。
del objectName
它的細節如下。
引數 | 說明 | |
---|---|---|
objectName |
強制 | 它是我們要刪除的物件,可以是任何資料型別或資料結構。它可以是任何資料型別或資料結構。 |
下面的程式顯示了我們如何在 Python 中使用這個關鍵字從一個字典中刪除一個鍵。
mydict = {"1": "Rose", "2": "Jasmine", "3": "Lili", "4": "Hibiscus"}
del mydict["3"]
print(mydict)
輸出:
{'1': 'Rose', '2': 'Jasmine', '4': 'Hibiscus'}
del
關鍵字已經刪除了鍵 3
,它的值是 Lili
。
現在,讓我們嘗試刪除一個不存在的鍵。
mydict = {"1": "Rose", "2": "Jasmine", "3": "Lili", "4": "Hibiscus"}
del mydict["5"]
print(mydict)
輸出:
KeyError: '5'
它丟擲了一個 KeyError
。
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub