First Class Functions in Python
- What Are First Class Functions?
- Passing Functions as Arguments
- Returning Functions from Functions
- Using Functions in Data Structures
- Practical Applications of First Class Functions
- Conclusion
- FAQ
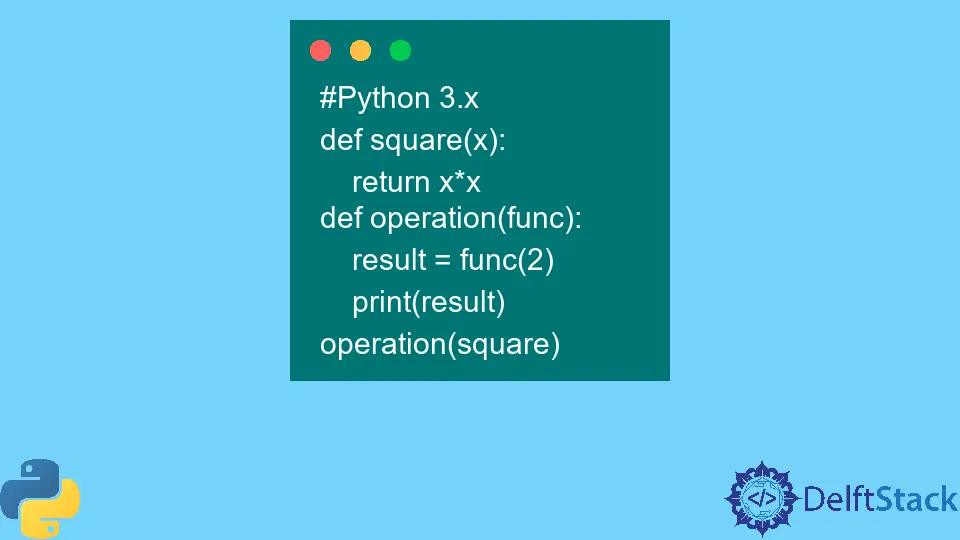
In the world of programming, functions are often seen as mere blocks of code designed to perform specific tasks. However, in Python, functions are treated as first-class citizens. This means they can be passed around and manipulated just like any other object.
In this tutorial, we will dive into what first-class functions are, how they work in Python, and why they are essential for writing clean, efficient, and flexible code. By the end of this article, you will have a solid understanding of first-class functions and be ready to incorporate them into your own projects. Let’s get started!
What Are First Class Functions?
First-class functions are a fundamental concept in Python, allowing functions to be treated like any other variable. This means you can assign functions to variables, pass them as arguments to other functions, return them from other functions, and store them in data structures. This capability opens the door to powerful programming techniques, such as higher-order functions, closures, and decorators, all of which enhance the flexibility and reusability of your code.
To illustrate this concept, let’s start with a simple example that assigns a function to a variable and calls it.
def greet(name):
return f"Hello, {name}!"
greeting = greet
print(greeting("Alice"))
Output:
Hello, Alice!
In this example, we define a function greet
that takes a name as an argument and returns a greeting. We then assign this function to the variable greeting
. When we call greeting("Alice")
, it behaves just like the original greet
function, demonstrating that functions in Python can be treated as first-class objects.
Passing Functions as Arguments
One of the most powerful aspects of first-class functions is the ability to pass them as arguments to other functions. This feature enables the creation of higher-order functions, which can take other functions as input and return new functions as output. Here’s a straightforward example to illustrate this concept.
def apply_function(func, value):
return func(value)
def square(x):
return x * x
result = apply_function(square, 5)
print(result)
Output:
25
In this code, we define a function apply_function
that takes two parameters: a function func
and a value value
. Inside apply_function
, we call the passed function with the given value. We also define a simple square
function that squares its input. By calling apply_function(square, 5)
, we pass the square
function as an argument, which results in the output of 25. This demonstrates how first-class functions allow for greater flexibility and modularity in coding.
Returning Functions from Functions
Another fascinating capability of first-class functions in Python is the ability to return a function from another function. This allows you to create closures, which can encapsulate a function along with its environment. Let’s look at a practical example.
def outer_function(message):
def inner_function():
return f"Message: {message}"
return inner_function
my_function = outer_function("Hello, World!")
print(my_function())
Output:
Message: Hello, World!
In this example, outer_function
takes a message
as an argument and defines an inner_function
that uses this message. The inner_function
is then returned by outer_function
. When we call outer_function("Hello, World!")
, it returns the inner_function
, which we assign to my_function
. When we call my_function()
, it retains access to the message
variable, demonstrating how closures work in Python.
Using Functions in Data Structures
First-class functions can also be stored in data structures like lists or dictionaries. This capability allows for dynamic function calls and can be particularly useful in various programming scenarios. Let’s explore how to store and utilize functions within a list.
def add(x):
return x + 1
def subtract(x):
return x - 1
functions = [add, subtract]
for func in functions:
print(func(10))
Output:
11
9
In this example, we define two functions, add
and subtract
, which perform simple arithmetic operations. We then store these functions in a list called functions
. By iterating over this list and calling each function with the value 10, we get the results of both operations. This demonstrates how first-class functions can be used to create a flexible collection of behaviors that can be easily manipulated and executed.
Practical Applications of First Class Functions
Understanding first-class functions is not just an academic exercise; it has real-world applications. For instance, they are extensively used in frameworks and libraries to create callbacks, event handlers, and even in functional programming paradigms. By leveraging first-class functions, you can write cleaner and more maintainable code.
Imagine a scenario where you need to filter a list of numbers based on specific criteria. You can use first-class functions to create a filter function that can accept various criteria as parameters. This flexibility allows you to create reusable and composable code that can adapt to different situations.
def filter_numbers(numbers, condition):
return [num for num in numbers if condition(num)]
even_condition = lambda x: x % 2 == 0
odd_condition = lambda x: x % 2 != 0
numbers = [1, 2, 3, 4, 5, 6]
print(filter_numbers(numbers, even_condition))
print(filter_numbers(numbers, odd_condition))
Output:
[2, 4, 6]
[1, 3, 5]
Here, we create a filter_numbers
function that takes a list of numbers and a condition function. We then define two lambda functions, even_condition
and odd_condition
, to filter even and odd numbers, respectively. This example showcases how first-class functions can enhance code reusability and clarity.
Conclusion
First-class functions are a powerful feature of Python that allows for greater flexibility and modularity in programming. By treating functions as first-class objects, you can pass them as arguments, return them from other functions, and store them in data structures. This capability opens up a world of possibilities for writing cleaner, more efficient code. As you continue to explore Python, remember the importance of first-class functions and how they can enhance your programming skills.
FAQ
-
What are first-class functions in Python?
First-class functions are functions that can be treated as objects, allowing them to be passed as arguments, returned from other functions, and assigned to variables. -
How can I use first-class functions in my code?
You can use first-class functions to create higher-order functions, closures, and more flexible code structures by passing functions as arguments or returning them from other functions. -
Can I store functions in data structures?
Yes, you can store functions in lists, dictionaries, and other data structures, enabling dynamic function calls and enhancing code reusability. -
What are some practical applications of first-class functions?
First-class functions can be used for callbacks, event handling, filtering data, and implementing functional programming techniques, making your code more modular and maintainable. -
Are first-class functions unique to Python?
No, first-class functions are a feature of many programming languages, including JavaScript, Ruby, and Lisp, though their implementation may vary.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn