How to Find All the Indices of an Element in a List in Python
-
Use of the
for
Loop to Find the Indices of All the Occurrences of an Element -
Use the
numpy.where()
Function to Find the Indices of All the Occurrences of an Element in Python -
Use the
more_itertools.locate()
Function to Find the Indices of All the Occurrences of an Element
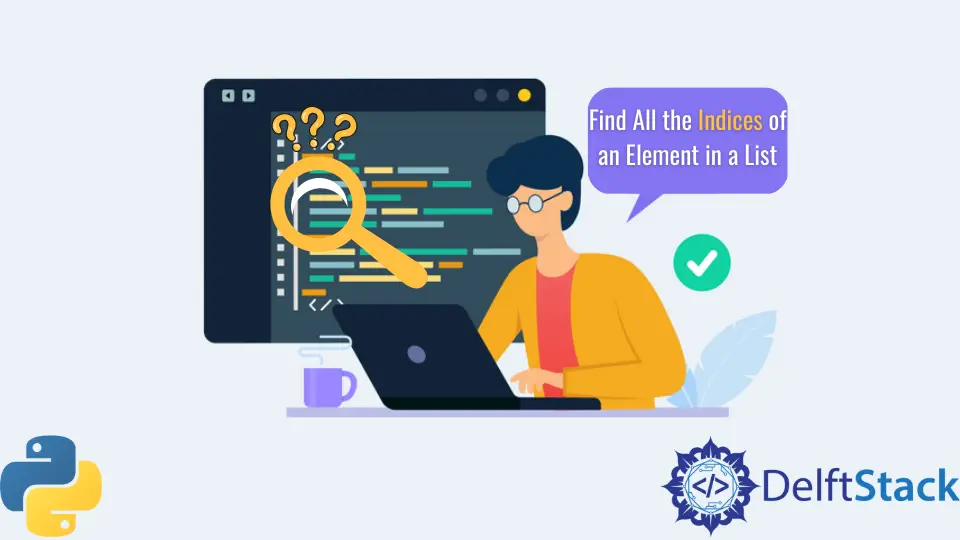
A list is used in Python to store multiple elements under a single name. Each element can be accessed using its position in the list. An element can be present at multiple positions in a list.
In this tutorial, we will introduce how to find the indices of all the occurrences of a specific element in a list. We will work with the following list and find all the indices of the element 1
.
l1 = [1, 5, 1, 8, 9, 15, 6, 2, 1]
Use of the for
Loop to Find the Indices of All the Occurrences of an Element
We can easily iterate over the list and compare each element to the required element and find its indices. We can store the final result in a new list. In the following example, we iterate over the list using the range()
function:
l1 = [1, 5, 1, 8, 9, 15, 6, 2, 1]
pos = []
x = 1 # The required element
for i in range(len(l1)):
if l1[i] == x:
pos.append(i)
print(pos)
Output:
[0, 2, 8]
A more efficient and compact way of implementing the above code is to use the list comprehension below.
l1 = [1, 5, 1, 8, 9, 15, 6, 2, 1]
pos = [i for i in range(len(l1)) if l1[i] == 1]
print(pos)
Output:
[0, 2, 8]
Similarly, we can also use the enumerate()
function, which returns the index and the value together. For example:
l1 = [1, 5, 1, 8, 9, 15, 6, 2, 1]
pos = [i for i, x in enumerate(l1) if x == 1]
print(pos)
Output:
[0, 2, 8]
Use the numpy.where()
Function to Find the Indices of All the Occurrences of an Element in Python
The NumPy
library has the where()
function, which is used to return the indices of an element in an array based on some condition. For this method, we have to pass the list as an array. The final result is also in an array. The following code snippet shows how we can use this method:
import numpy as np
l1 = [1, 5, 1, 8, 9, 15, 6, 2, 1]
pos = np.where(np.array(l1) == 1)[0]
print(pos)
Output:
[0 2 8]
Use the more_itertools.locate()
Function to Find the Indices of All the Occurrences of an Element
The more_itertools
is a third party and handy module. It has many functions that can create efficient and compact code when working with iterables. The locate()
function in this module returns the indices of the elements which are True
for the condition. It returns an itertools
object. The following code snippet explains how we can use this method:
from more_itertools import locate
l1 = [1, 5, 1, 8, 9, 15, 6, 2, 1]
pos = list(locate(l1, lambda x: x == 1))
print(pos)
Output:
[0, 2, 8]
We use the list()
function to ensure that the final result is in the form of a list.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python