用 Python 查詢列表中元素的所有索引
-
使用
for
迴圈查詢元素的所有出現的指數 -
使用
numpy.where()
函式查詢 Python 中一個元素的所有出現的索引 -
使用
more_itertools.locate()
函式查詢元素的所有出現的指數
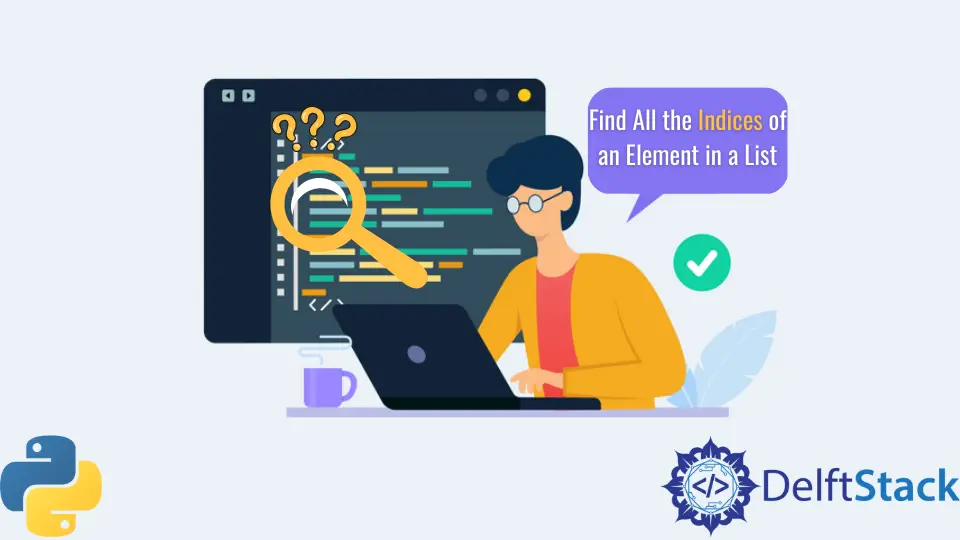
在 Python 中,列表用於在一個名稱下儲存多個元素。每個元素可以通過它在列表中的位置來訪問。一個元素可以出現在列表中的多個位置。
在本教程中,我們將介紹如何查詢列表中特定元素的所有出現的索引。我們將在下面的列表中查詢元素 1
的所有索引。
l1 = [1, 5, 1, 8, 9, 15, 6, 2, 1]
使用 for
迴圈查詢元素的所有出現的指數
我們可以很容易地對列表進行迭代,並將每個元素與所需元素進行比較,並找到其索引。我們可以將最終結果儲存在一個新的列表中。在下面的例子中,我們使用 range()
函式對列表進行迭代。
l1 = [1, 5, 1, 8, 9, 15, 6, 2, 1]
pos = []
x = 1 # The required element
for i in range(len(l1)):
if l1[i] == x:
pos.append(i)
print(pos)
輸出:
[0, 2, 8]
實現上述程式碼的更高效、更緊湊的方法是使用下面的列表推導。
l1 = [1, 5, 1, 8, 9, 15, 6, 2, 1]
pos = [i for i in range(len(l1)) if l1[i] == 1]
print(pos)
輸出:
[0, 2, 8]
同樣,我們也可以使用 enumerate()
函式,將索引和值一起返回。比如說
l1 = [1, 5, 1, 8, 9, 15, 6, 2, 1]
pos = [i for i, x in enumerate(l1) if x == 1]
print(pos)
輸出:
[0, 2, 8]
使用 numpy.where()
函式查詢 Python 中一個元素的所有出現的索引
NumPy
庫有 where()
函式,用於根據某些條件返回一個陣列中元素的索引。對於這個方法,我們必須將列表作為一個陣列傳遞。最終的結果也是以陣列的形式出現。下面的程式碼片段展示了我們如何使用這個方法。
import numpy as np
l1 = [1, 5, 1, 8, 9, 15, 6, 2, 1]
pos = np.where(np.array(l1) == 1)[0]
print(pos)
輸出:
[0 2 8]
使用 more_itertools.locate()
函式查詢元素的所有出現的指數
more_itertools
是一個第三方的方便模組。它有許多功能,可以在處理可迭代元素時建立高效和緊湊的程式碼。該模組中的 locate()
函式返回條件為 True
的元素的索引。它返回一個 itertools
物件。下面的程式碼片段解釋了我們如何使用這個方法。
from more_itertools import locate
l1 = [1, 5, 1, 8, 9, 15, 6, 2, 1]
pos = list(locate(l1, lambda x: x == 1))
print(pos)
輸出:
[0, 2, 8]
我們使用 list()
函式來確保最終結果是一個列表的形式。
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn