How to Perform Element-Wise Addition in Python
- Element-Wise Addition in Python
-
Perform Element-Wise Addition Using List Comprehension and
zip()
Function in Python -
Perform Element-Wise Addition Using the
map()
Function in Python -
Perform Element-Wise Addition Using
NumPy
in Python - Conclusion
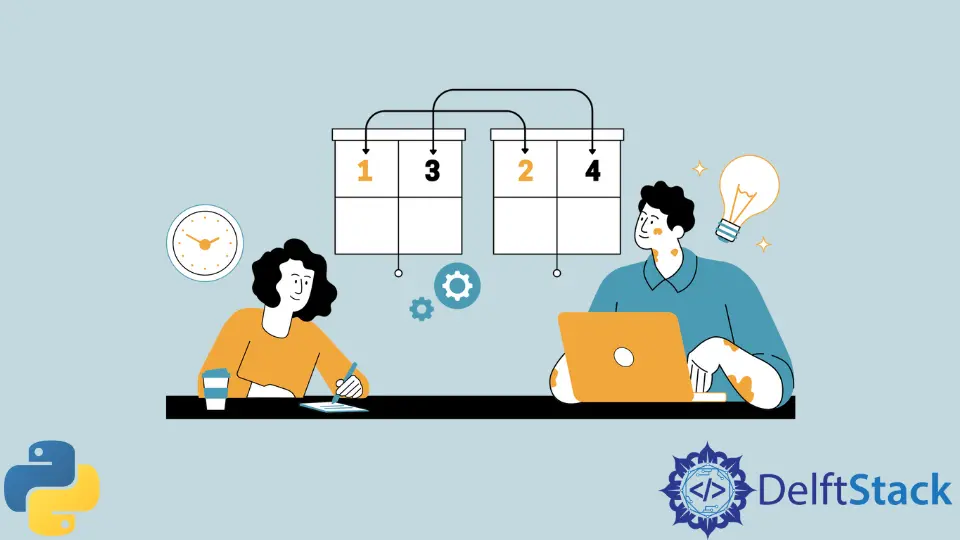
We will introduce different methods to add two lists element-wise in Python with examples.
Element-Wise Addition in Python
While working with lists in Python, there may be situations where the need arises to add both lists element-wise. Element-wise addition is a fundamental operation in various numerical and data manipulation tasks.
Python offers versatile solutions for tackling this common requirement. In the sections below, we will explore different effective methods, accompanied by code examples.
Perform Element-Wise Addition Using List Comprehension and zip()
Function in Python
The zip()
function in Python combines elements from multiple iterables into tuples, pairing up elements at the same positions. It creates an iterator of tuples, stopping when the shortest input iterable is exhausted.
Executing element-wise addition using list comprehension and the zip()
function in Python involves creating a new list by adding corresponding elements from two or more lists. Again, the zip()
function pairs up the elements at the same positions in multiple lists, and then list comprehension is used to iterate through these pairs and generate a new list containing the sums.
Code 1:
firstList = (1, 2, 9, 8, 99, 89)
secondList = (14, 24, 56, 38, 97, 11)
additionList = [sum(element) for element in zip(firstList, secondList)]
print(additionList)
Output:
[15, 26, 65, 46, 196, 100]
In this code above, we have two lists, firstList
and secondList
, each containing numerical elements. Next, we use the zip()
function to combine elements from both lists into tuples, and the list comprehension iterates through these tuples.
Then, the sum(element)
part calculates the sum of each tuple, representing the element-wise addition. The result is a new list named additionList
.
The output [15, 26, 65, 46, 196, 100]
, where each element corresponds to the sum of the respective elements from firstList
and secondList
.
Let’s have another example where we use the operator +
instead of sum()
function in the above example.
Code 2:
firstList = (1, 2, 9, 8, 99, 89)
secondList = (14, 24, 56, 38, 97, 11)
result = [x + y for x, y in zip(firstList, secondList)]
print(result)
Output:
[15, 26, 65, 46, 196, 100]
In this code, we initialize two lists, firstList
and secondList
, each containing numerical elements. Next, the zip()
function combines elements from both lists, creating tuples that pair up corresponding elements.
The list comprehension [x + y for x, y in zip(firstList, secondList)]
then iterates through these tuples, adding the elements together. The resulting list, named result
, encapsulates the element-wise sums.
When we print result
, the output is [15, 26, 65, 46, 196, 100]
. Each element in this list represents the sum of the respective elements from firstList
and secondList
.
Perform Element-Wise Addition Using the map()
Function in Python
The map()
is another function in Python that sums up one or two iterables. It takes a return function and takes one or more iterables as the input and works on it to provide a new tuple or set which contains the sum of the two tuples.
It works on all the iterables based on the index of elements in their respective lists. Every iteration chooses one element and passes it to the return function, which, in this case, is the lambda
function that returns only one expression at a time.
The values or elements from the return function are stored in an object of the map class and converted into linear values.
Code:
firstList = (1, 2, 9, 8, 99, 89)
secondList = (14, 24, 56, 38, 97, 11)
additionList = list(map(lambda x, y: x + y, firstList, secondList))
print(additionList)
Output:
[15, 26, 65, 46, 196, 100]
In this code, we begin with two lists, firstList
and secondList
, each containing numerical elements. Next, the map()
function applies the lambda
function to corresponding elements from both lists, generating a new iterable.
The lambda
function lambda x, y: x + y
defines the addition operation for each pair of elements. By converting the result to a list using list()
, we obtain the additionList
.
When we print additionList
, the output is [15, 26, 65, 46, 196, 100]
, where each element signifies the sum of the respective elements from firstList
and secondList
.
Perform Element-Wise Addition Using NumPy
in Python
We can also use NumPy
to add the elements from two lists element-wise. NumPy
can deal with complex numbers.
It is the standard trigonometric function. It will convert the lists to a NumPy
array after applying different operations in case of addition and then convert this NumPy
array to the list again.
Let’s go through an example and implement the same scenario we used in the above examples. First, we will install NumPy
using the following command shown.
Command:
pip install numpy
As shown below, we will import it inside our program and use it to perform the element-wise addition of two lists.
Code:
import numpy as np
firstList = (1, 2, 9, 8, 99, 89)
secondList = (14, 24, 56, 38, 97, 11)
additionList = list(np.array(firstList) + np.array(secondList))
print(additionList)
Output:
[15, 26, 65, 46, 196, 100]
In this code, we import the NumPy
library. Then, we initialize two lists, firstList
and secondList
, each containing numerical elements. Next, we execute element-wise addition on these lists by converting the lists to NumPy
arrays using np.array()
and then directly adding them together.
The resulting array represents the element-wise sums of the corresponding elements from firstList
and secondList
. To obtain the final additionList
, we convert this NumPy
array back to a Python list using list()
.
When we print additionList
, the output is [15, 26, 65, 46, 196, 100]
, where each element signifies the sum of the respective elements from the original lists.
Conclusion
In conclusion, this article introduced three methods for performing element-wise addition of two lists in Python. The methods explored include using list comprehension with the zip()
function, the map()
function with lambda expressions, and utilizing the NumPy
Library.
Each method was accompanied by illustrative code examples, demonstrating the versatility of Python in addressing element-wise addition scenarios.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python