How to Divide a List by a Number in Python
-
Use a
for
Loop to Divide a List by a Number in Python -
Use a
while
Loop to Divide a List by a Number in Python - Use List Comprehension to Divide a List by a Number in Python
-
Use the
NumPy
Library to Divide a List by a Number in Python - Conclusion
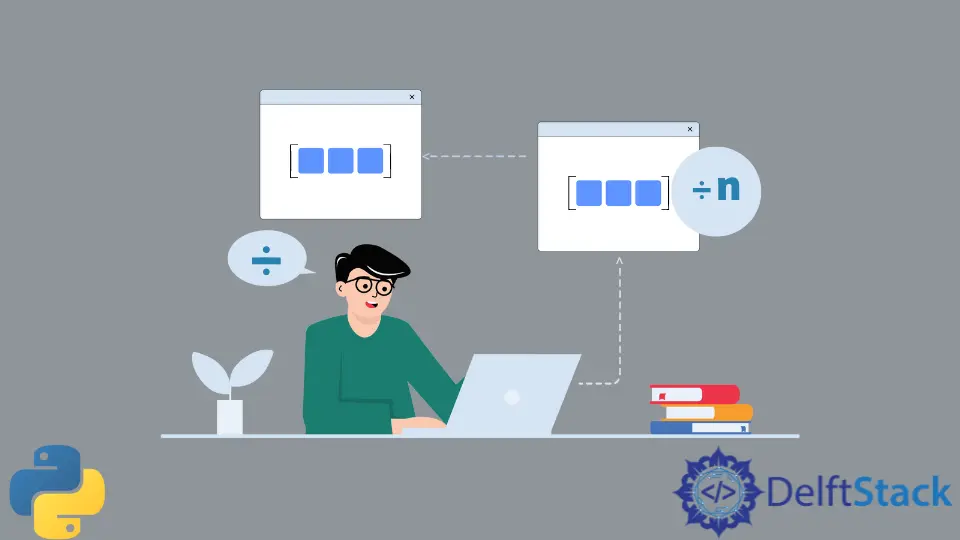
Dividing a list by a number in Python is a fundamental operation that is essential for numerical computing and data manipulation tasks. The process applies the division operation to each element within the list, resulting in a transformed list where each value is divided by a specified number.
In this article, we’ll explore the different ways to divide a list by a number.
Use a for
Loop to Divide a List by a Number in Python
Using loops to divide a list by a number in Python involves iterating through each element in the list and performing the division operation. This approach is useful when you want to apply a specific operation to each item in the list individually.
First, we will be using a for
loop to perform this task. Let’s go through an example in which we will create a list of numbers that we will divide by 5
.
Code 1:
listToDivide = [5, 10, 15, 20, 25, 30, 35, 40, 45, 50]
print("List before dividing by 5: ", listToDivide)
newList = []
for items in listToDivide:
new = items / 5
newList.append(int(new))
print("List after dividing by 5: ", newList)
Output:
List before dividing by 5: [5, 10, 15, 20, 25, 30, 35, 40, 45, 50]
List after dividing by 5: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
In the code above, we start with a list called listToDivide
containing values from 5
to 50
. Then, we use a for
loop to iterate over each item in listToDivide
.
Within the loop, we divide each element by 5
, convert the result to an integer, append it to newList
, and print the modified list (newList
). As we can see from the example above, we can easily divide a list by a specific number using the a for
loop.
But what if we want to save the data with no remainder left after being divided by that number? Let’s use this concept in the following example.
In this following example, we’ll make two different lists to save numbers with and without remainders.
Code 2:
listToDivide = [
3,
5,
7,
10,
13,
15,
17,
20,
23,
25,
29,
30,
33,
35,
37,
40,
41,
45,
47,
50,
]
print("List before dividing by 5: ", listToDivide)
newIntList = []
newFloatList = []
for items in listToDivide:
if items % 5 == 0:
newIntList.append(int(items))
else:
newFloatList.append(items)
print("Numbers divisible by 5: ", newIntList)
print("Numbers not divisible by 5: ", newFloatList)
Output:
List before dividing by 5: [3, 5, 7, 10, 13, 15, 17, 20, 23, 25, 29, 30, 33, 35, 37, 40, 41, 45, 47, 50]
Numbers divisible by 5: [5, 10, 15, 20, 25, 30, 35, 40, 45, 50]
Numbers not divisible by 5: [3, 7, 13, 17, 23, 29, 33, 37, 41, 47]
In this code, we categorize integers from the list listToDivide
into two groups: those divisible by 5
(newIntList
) and those not divisible by 5
(newFloatList
). The original list is printed for reference, and a for
loop efficiently classifies each number based on the modulo operator (% 5
).
The output displays the original list and the two categorized lists, demonstrating the successful segregation of numbers divisible and not divisible by 5
.
Use a while
Loop to Divide a List by a Number in Python
Now, let’s discuss another method that can be used to divide a list by a number. In this method, we will use a while
loop.
So, let’s use a while
loop with the same scenario as we discussed in our first example.
Code 1:
listToDivide = [5, 10, 15, 20, 25, 30, 35, 40, 45, 50]
print("List before dividing by 5: ", listToDivide)
newList = []
a = 0
while a < len(listToDivide):
new = listToDivide[a] / 5
newList.append(int(new))
a = a + 1
print("List after dividing by 5: ", newList)
Output:
List before dividing by 5: [5, 10, 15, 20, 25, 30, 35, 40, 45, 50]
List after dividing by 5: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
In this code, we divide each element in the list listToDivide
by 5
and store the results in a new list called newList
. Using a while
loop, we iterate through the elements, perform the division, and append the integer results to newList
.
The output displays both the original and modified lists, showcasing the successful element-wise division.
As we can see, we can easily divide a list by a specific number using the while
loop. The results are the same as in the for
loop.
Now, let’s use the concept in our second example and implement it by using the while
loop and saving the results in two lists based on whether the element is divisible by a number. Let’s have an example where we can use the while
loop for the same purpose.
Code 2:
listToDivide = [
3,
5,
7,
10,
13,
15,
17,
20,
23,
25,
29,
30,
33,
35,
37,
40,
41,
45,
47,
50,
]
print("List before dividing by 5: ", listToDivide)
newIntList = []
newFloatList = []
a = 0
while a < len(listToDivide):
if listToDivide[a] % 5 == 0:
newIntList.append(int(listToDivide[a]))
a = a + 1
else:
newFloatList.append(listToDivide[a])
a = a + 1
print("Numbers divisible by 5: ", newIntList)
print("Numbers not divisible by 5: ", newFloatList)
Output:
List before dividing by 5: [3, 5, 7, 10, 13, 15, 17, 20, 23, 25, 29, 30, 33, 35, 37, 40, 41, 45, 47, 50]
Numbers divisible by 5: [5, 10, 15, 20, 25, 30, 35, 40, 45, 50]
Numbers not divisible by 5: [3, 7, 13, 17, 23, 29, 33, 37, 41, 47]
In this code, we categorize integers from the list listToDivide
into two groups: those divisible by 5
(newIntList
) and those not divisible by 5
(newFloatList
). The original list is printed for reference, and a while
loop efficiently classifies each number based on the modulo operator (% 5
).
The output displays the original list and the two categorized lists, showcasing the successful segregation of numbers divisible and not divisible by 5
.
As we can see, we can implement the same logic with both for
and while
loops and get the same results. Loops make it easier for us to iterate through each list element and perform any task with it as we want.
Use List Comprehension to Divide a List by a Number in Python
Another way to divide a list by a number is using list comprehension. This method is a one-line method; we write the code in one line so that it is executed.
It is a very complex method to use in our second example, in which we will be separating the elements based on whether they are divisible by a specific number or not.
So, let’s use list comprehension to divide a list by a number.
Code:
listToDivide = [5, 10, 15, 20, 25, 30, 35, 40, 45, 50]
print("List before dividing by 5: ", listToDivide)
modifiedlist = []
[modifiedlist.append(int(i / 5)) for i in listToDivide]
print("List after dividing by 5: ", modifiedlist)
Output:
List before dividing by 5: [5, 10, 15, 20, 25, 30, 35, 40, 45, 50]
List after dividing by 5: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
In this code, we use list comprehension to efficiently divide each element in the listToDivide
by 5
and store the results in a new list called modifiedlist
. The original list is printed for reference, and the output displays both the original (ListToDivide
) and modified list (modifiedlist
), showcasing the successful element-wise division.
Use the NumPy
Library to Divide a List by a Number in Python
The NumPy
is a powerful open-source library in Python for numerical computing. It provides assistance for large, multi-dimensional arrays and matrices, coupled with a set of advanced mathematical functions designed to perform operations on these arrays.
NumPy
simplifies the process by allowing us to perform the division operation directly on the entire array. If we haven’t installed NumPy
, we can do so using the following command in our terminal.
Command:
pip install numpy
Now, let’s have an example of using the NumPy
library to divide a list by a number.
Code:
import numpy as np
def divide_list_by_number(lst, divisor):
return np.array(lst) / divisor
listToDivide = [5, 10, 15, 20, 25, 30, 35, 40, 45, 50]
divisor = 5
print("List before dividing by 5: ", listToDivide)
newList = divide_list_by_number(listToDivide, divisor)
print("List after dividing by 5: ", newList)
Output:
List before dividing by 5: [5, 10, 15, 20, 25, 30, 35, 40, 45, 50]
List after dividing by 5: [ 1. 2. 3. 4. 5. 6. 7. 8. 9. 10.]
In this code, we utilize the NumPy
library to efficiently divide each element in the list listToDivide
by 5
. We define a function, divide_list_by_number
, which performs the division using NumPy’s array division.
The original list (listToDivide
) is printed for reference, and the output displays the modified list (newList
) obtained by dividing each element in the original list by 5
using NumPy
.
Conclusion
In conclusion, we explored various methods to divide a list by a number in Python. Using a for
loop allows us to operate on each element individually, offering flexibility in customization.
The while
loop provides an alternative approach, achieving the same results as the for
loop. List comprehension offers a concise, one-line solution, simplifying the code for straightforward tasks.
Additionally, leveraging the NumPy
library streamlines the process, enabling direct and efficient division operations on entire arrays. These methods provide flexibility in handling element-wise operations on lists in Python depending on the specific requirements and preferences.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python