How to Disable Path Length Limit in Python
- Disable Path Limit in Python During Python Installation
- Disable Path Limit in Python by Modifying the Registry Key
-
Disable Path Limit in Python by Overriding the
os.path.exists()
Function - Conclusion
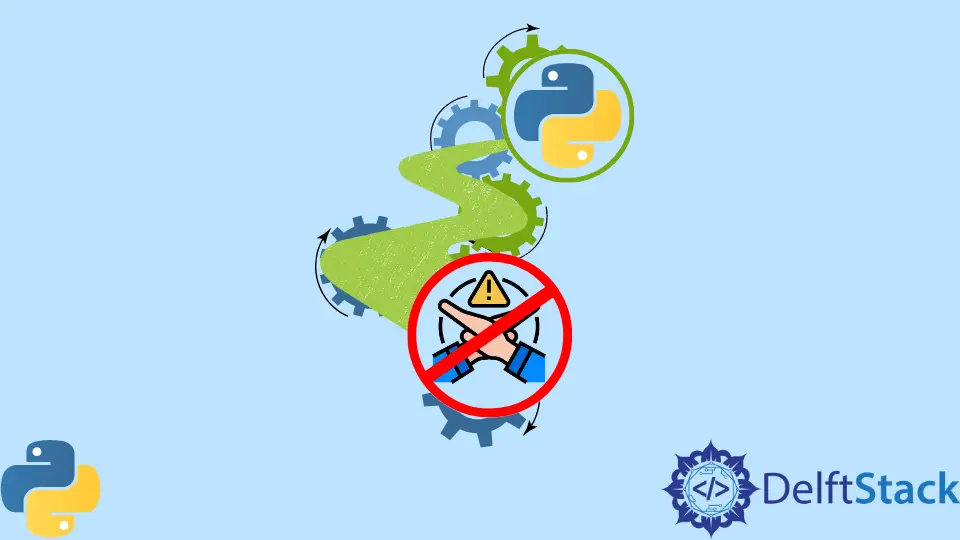
In programming, we deal with problems where we have to work with external files and modules. Therefore, we need to know the location of files stored on the disk specified by the file path.
When working with file paths in Python on a Windows system, you might encounter the notorious MAX_PATH limit
or file name or path length of file being too long
, which restricts paths to 260 characters. This limitation can lead to issues when dealing with deeply nested directories or long file names.
This issue is not a problem for macOS or Linux-based systems and exists only in Windows. This limit was put in place due to the path limit restriction in the initial versions of Windows OS.
To overcome such issues, we can shorten the path or filename to meet the required length.
However, suppose someone wants to remove this limit, especially if they installed Python in a directory that exceeds the path length limit. In that case, it is recommended to disable it when the option is provided.
There are several methods to disable the path length limit in Python, providing greater flexibility and compatibility with longer paths.
Disable Path Limit in Python During Python Installation
During the Python installation process, you have the option to enable the "Add Python to PATH"
setting.
While this doesn’t directly address the MAX_PATH limit
, it ensures that the Python executable is easily accessible from any directory. By having Python in the system’s PATH
, you can use shorter, relative paths to your Python scripts.
On the other hand, there is an option to "Disable path length limit"
. This option removes the Windows filesystem limitation of 260 characters for paths that include a folder path with the filename and its extension.
Disable Path Limit in Python by Modifying the Registry Key
To disable the MAX_PATH limit
using the Windows Registry Editor, you can follow the following:
-
Press Win + R to open the Run dialog and type
regedit
, then press Enter in order to open the Registry Editor. -
Navigate to the following key inside the Registry Editor:
HKEY_LOCAL_MACHINE\SYSTEM\CurrentControlSet\Control\FileSystem
. -
In the right pane, double-click on the
LongPathsEnabled
value. -
Set the value data to
1
and clickOK
. -
Close the Registry Editor Window and restarting the computer is a must.
-
After disabling the path length limit, reinstall Python and make sure to
Disable path length limit
during the installation process
Disable Path Limit in Python by Overriding the os.path.exists()
Function
You can use the os.path.exists()
function to check if a file or directory exists, regardless of the length of the path. This temporarily disables the path length limit on a Windows system.
By default, the function raises a FileNotFoundError
if the path does not exist, but this can be overridden by using the os.path.exists()
function with a lambda function that always returns True
, effectively disabling the path length limit.
Here is an example:
import os
# Disable the path length limit
os.path.exists = lambda x: True
# Check if a file exists with a long path
long_path = "C:/path/to/a/long/file"
if os.path.exists(long_path):
print(f"The file {long_path} exists.")
else:
print(f"The file {long_path} does not exist.")
# Restore the default behavior of the os.path.exists() function
os.path.exists = os.path.exists
Output:
The file C:/path/to/a/long/file exists.
This code may be used in scenarios where dealing with long file paths on Windows is necessary, and temporarily bypassing the path length limitation is acceptable for specific operations.
However, such manipulations should be done with caution, considering potential implications on system behavior and file operations.
Conclusion
In this article, we explored various methods to disable the MAX_PATH
limit in Python, providing solutions for handling longer paths on Windows systems. Each method offers a unique approach, from configuring Python installation options and modifying the Windows Registry to overriding the os.path.exist()
Function.
Developers can guarantee compatibility with longer paths by learning and applying these techniques; however, when employing long file/directory names and paths, disabling this option may cause your programs to become incompatible with older Windows 10 and lower versions of the operating system.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn