How to Convert Dictionary to String in Python
-
Use the
for
Loop to Convert Dictionary to String in Python -
Use the
str()
Function to Convert Dictionary to String in Python -
Use the
json
Module to Convert Dictionary to String in Python -
Use the
pprint
Module to Convert Dictionary to String in Python - Conclusion
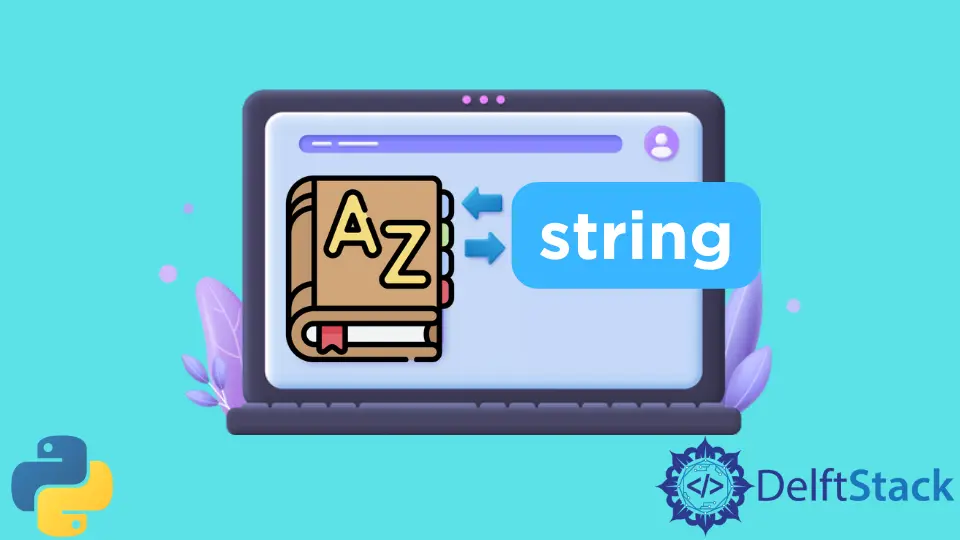
Dictionaries are an essential data structure in Python, allowing us to store and retrieve data in a key-value format. They provide a powerful way to organize and manipulate data efficiently.
However, a common challenge arises when displaying or transmitting dictionary data: converting a dictionary to a string representation. Unlike lists or tuples, dictionaries have a unique structure that requires special handling to convert them to a human-readable string format.
To illustrate the importance of this problem, let’s consider a real-world example. Imagine we are building an e-commerce platform and need to send customers an order confirmation email.
The order details, such as item names, quantities, and prices, are stored in a dictionary. However, email services typically require the email content to be in string format.
Thus, converting the order dictionary to a string becomes vital to include the order details in the email body.
In this tutorial, we will explore different approaches to efficiently convert dictionaries to strings in Python, equipping us with the knowledge and techniques necessary to tackle this common challenge effectively.
Use the for
Loop to Convert Dictionary to String in Python
When converting a dictionary to a string in Python, one simple and effective approach is to utilize a for
loop. This method allows us to iterate over the key-value pairs in the dictionary and construct a string representation of the data.
The following code example demonstrates how we can convert a dictionary to a string using Python’s conventional for
loop.
def dict_to_string(dictionary):
string_representation = ""
for key, value in dictionary.items():
string_representation += str(key) + ": " + str(value) + ", "
string_representation = string_representation.rstrip(", ")
return string_representation
dict = {"Hello": 60}
s = dict_to_string(dict)
print(type(s))
print(s)
Output:
The code defines a function called dict_to_string
that takes a dictionary as input. It initializes an empty string, string_representation
.
It then iterates over each key-value pair in the dictionary using a for
loop. Inside the loop, it converts the key and value to strings and appends them to string_representation
with a colon and space separator.
After the loop, it uses the rstrip()
method to remove the trailing comma and space. The function returns the resulting string representation.
The code’s main body creates a dictionary with a single key-value pair. The dict_to_string
function is called with this dictionary, and the returned string is stored in variable s
.
Finally, the type of s
is printed, followed by the string representation.
Use the str()
Function to Convert Dictionary to String in Python
In Python, the str()
function provides a straightforward and convenient way to convert a dictionary to a string representation. The str()
function takes an object as a parameter and returns its string representation.
It converts the dictionary to a string format when applied to a dictionary.
Syntax:
string_representation = str(dictionary)
The str()
function converts the dictionary to a string by representing its contents in a formatted string representation. The resulting string will include the curly braces { }
to denote a dictionary and the key-value pairs in a readable format.
Unlike the previously discussed for
loop method, the str()
function provides a more concise and direct approach to converting a dictionary to a string. It handles the conversion automatically without requiring manual iteration or string concatenation.
The following code example demonstrates how we can convert a dictionary to a string using Python’s str()
function.
dict = {"Hello": 60}
s = str(dict)
print(type(s))
print(s)
Output:
The above code creates a dictionary dict
with a single key-value pair, where the key is 'Hello'
, and the value is 60
. The str()
function is then applied to the dictionary, converting it to a string representation.
The resulting string is stored in the variable s
. The print(type(s))
statement displays the type of s
, confirming that it is a string.
Finally, the print(s)
statement outputs the string representation of the dictionary, which includes the curly braces {}
and the key-value pair 'Hello': 60
.
Use the json
Module to Convert Dictionary to String in Python
In Python, the json
module provides a powerful and versatile way to convert a dictionary to a string representation. The json.dumps()
function, short for dump string
, allows us to serialize a dictionary into a JSON-formatted string.
JSON (JavaScript Object Notation) is a widely used data interchange format that is human-readable and easily interpreted by many programming languages.
Syntax:
import json
string_representation = json.dumps(dictionary)
The json.dumps()
function takes a dictionary as its parameter and returns a string representation of the dictionary in JSON format. It serializes the dictionary by converting its keys and values into a string representation that adheres to the JSON syntax.
The json.dumps()
function also supports additional parameters to customize its behavior. Some of the commonly used parameters are:
indent
: Specifies the number of spaces used for indentation in the resulting JSON string.sort_keys
: When set toTrue
, it alphabetically sorts the keys in the output JSON string.
Comparing the json.dumps()
function with the previously discussed for
loop and str()
function methods, the json
module offers distinct advantages. It provides a standardized and widely supported format (JSON) for data interchange, ensuring compatibility across different platforms and programming languages.
Additionally, it automatically handles the conversion process, eliminating the need for manual iteration or string concatenation. The json.dumps()
method provides more flexibility and control over the resulting string representation.
Utilizing its optional parameters, we can format the JSON string to meet specific requirements, such as pretty-printing with an indentation or sorting the keys.
The following code example demonstrates how we can convert a dictionary to a string using Python’s json
module.
import json
dict = {"Hello": 60}
s = json.dumps(dict)
print(type(s))
print(s)
Output:
The provided code first imports the json
module. It then creates a dictionary named dict
with a single key-value pair, where the key is 'Hello'
, and the value is 60
.
The json.dumps()
function converts the dictionary to a JSON-formatted string assigned to the variable s
. The print(type(s))
statement displays the type of s
, confirming that it is a string.
Finally, the print(s)
statement outputs the JSON string representation of the dictionary, which includes the key-value pair "Hello": 60
.
Use the pprint
Module to Convert Dictionary to String in Python
The pprint
module in Python provides a convenient way to convert a dictionary to a string representation with improved readability. The pprint.pformat()
function, short for pretty format
, allows us to format a dictionary as a string in a more structured and visually appealing manner.
Syntax:
import pprint
string_representation = pprint.pformat(dictionary)
The pprint.pformat()
function takes a dictionary as its parameter and returns a string representation of the dictionary with improved formatting. It indents the keys and values, making the resulting string easier to read and comprehend.
The pprint
module does not have additional parameters for the pformat()
function. However, it provides optional parameters for controlling the formatting when using the pprint.pprint()
function to print the dictionary directly.
These parameters include indent
, width
, depth
, and compact
. Comparing the pprint
module with the previously discussed methods offers distinct advantages.
The pprint
module automatically handles the formatting and indentation of the dictionary, eliminating the need for manual iteration or string concatenation. It produces a well-structured string representation that improves readability and makes it easier to analyze complex dictionaries.
The following code example demonstrates how we can convert a dictionary to a string using Python’s pprint
module.
import pprint
dict = {"Hello": 60}
s = pprint.pformat(dict)
print(type(s))
print(s)
Output:
The above code begins by importing the pprint
module. It then creates a dictionary named dict
with a single key-value pair, where the key is 'Hello'
, and the value is 60
.
The pprint.pformat()
function converts the dictionary to a formatted string representation. The resulting formatted string is assigned to the variable s
.
The print(type(s))
statement displays the type of s
, confirming that it is a string. Finally, the print(s)
statement outputs the formatted string representation of the dictionary, which includes the key-value pair "Hello": 60
with a proper indentation for improved readability.
Conclusion
We have explored multiple methods for converting a dictionary to a string in Python: the for
loop, the str()
function, the json
module, and the pprint
module. Each method has its advantages and disadvantages, depending on the specific requirements and preferences of the task.
The for
loop method provides flexibility and control over the string representation. It allows formatting customization and is suitable for cases where a specific string format is desired. However, it requires manual iteration and concatenation, which can be cumbersome and less efficient.
The str()
function provides a concise approach to converting a dictionary to a string. It automatically handles the conversion process but may lack customization options for formatting the string representation according to specific needs.
The json
module is beneficial for interoperability and data interchange. It converts dictionaries to JSON strings, widely supported and compatible with various platforms and programming languages. However, it may not provide the same level of control over formatting as the for
loop method.
The pprint
module improves readability by creating visually appealing and well-structured string representations. It automatically handles indentation and formatting, making complex dictionaries more comprehensible. However, it does not convert dictionaries to a specific format like JSON.
Related Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python