How to Delete Element From List in Python
-
Delete Element Using the
remove()
Function in Python -
Delete Element From the List Using the
del()
Function in Python -
Delete Element From the List Using the
pop()
Function in Python
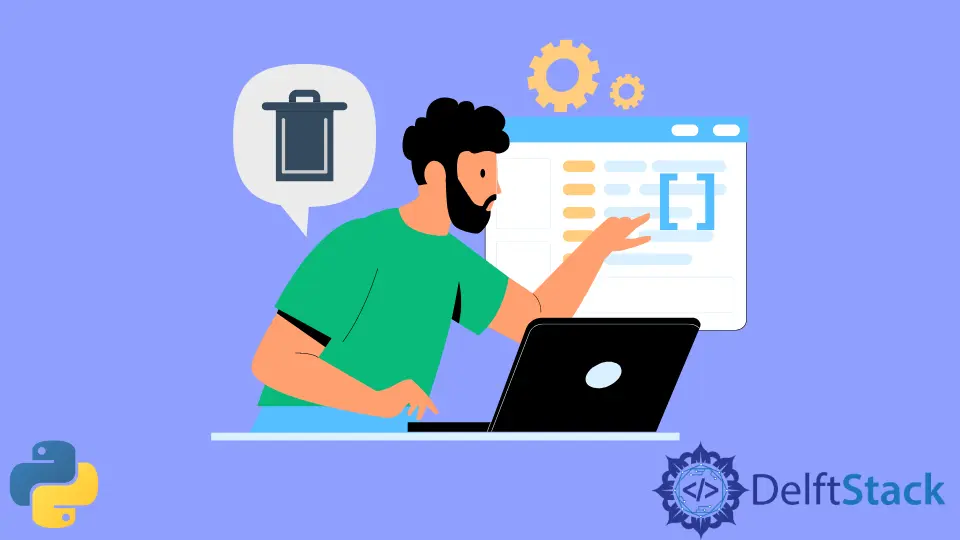
If you are working with the list, and you want to remove an element from the list without making lots of effort and want to save your time, you can use the Python built-in functions to remove that particular element from the list.
In this article, we will learn how to delete an element in a list by value in a very simple way. When we work with list operations, there are times when we need to delete certain elements from the list permanently. Fortunately, Python has many different yet simple and convenient ways to remove those particular elements from the list.
There are various ways to delete or remove the element from the list, for example, remove()
, del()
, and pop()
methods etc.
Delete Element Using the remove()
Function in Python
In this code block, we will delete the list element by using the remove()
built-in method. The remove()
method deletes the first found element with the matched value in a given list. This method is mandatory to use when you are sure that you want to delete a specific value despite the index. In the code below, we will delete 18 from the list so that we will pass it in the remove()
method.
list = [4, 6, 18, 4, 9, 11]
print("List before calling remove() function:")
print(list)
list.remove(18)
print("List after calling remove() function:")
print(list)
Output:
List before calling remove() function:
[4, 6, 18, 4, 9, 11]
List after calling remove() function:
[4, 6, 4, 9, 11]
Delete Element From the List Using the del()
Function in Python
In this code block, we will delete the list element by using the del()
built-in method. The del()
method deletes the given index value in the list. This method is mandatory when you are very sure that you want to delete specific index values according to the requirements. In the code below, we will delete the fourth index (starting from the 0, so it will be 3) from the list so that we will pass it in the del()
method of the Python. For this attempt, let’s look at how we can delete a value at a specific index with the del()
keyword:
list = [1, 4, 6, 2, 6, 1]
print("List before calling del() function:")
print(list)
del list[3]
print("List after calling del() function:")
print(list)
Output:
List before calling del() function:
[1, 4, 6, 2, 6, 1]
List after calling del() function:
[1, 4, 6, 6, 1]
Delete Element From the List Using the pop()
Function in Python
In this code block, we will delete the list element by using the pop()
built-in method. The pop()
method deletes the given index value in the list. This method is mandatory when you are very sure that you want to delete specific index values according to the requirements. In the code below, we will delete the 4th index (starting from the 0, so it will be 3) from the list so that we will pass it in the pop()
method of the Python. For this attempt, let’s look at how we can delete a value at a certain index with the del()
keyword:
list = [1, 4, 6, 2, 6, 1]
print("List before calling pop() function:")
print(list)
list.pop(0)
print("List after calling pop() function with index :")
print(list)
list.pop()
print("List after calling pop() function without index :")
print(list)
Output:
List before calling pop() function:
[1, 4, 6, 2, 6, 1]
List after calling pop() function with index :
[4, 6, 2, 6, 1]
List after calling pop() function without index :
[4, 6, 2, 6]
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python