How to Declare an Array in Python
-
Python Declaration of Arrays as Represented by the Class
list
-
Declare an Array in Python by Importing the
array
Module
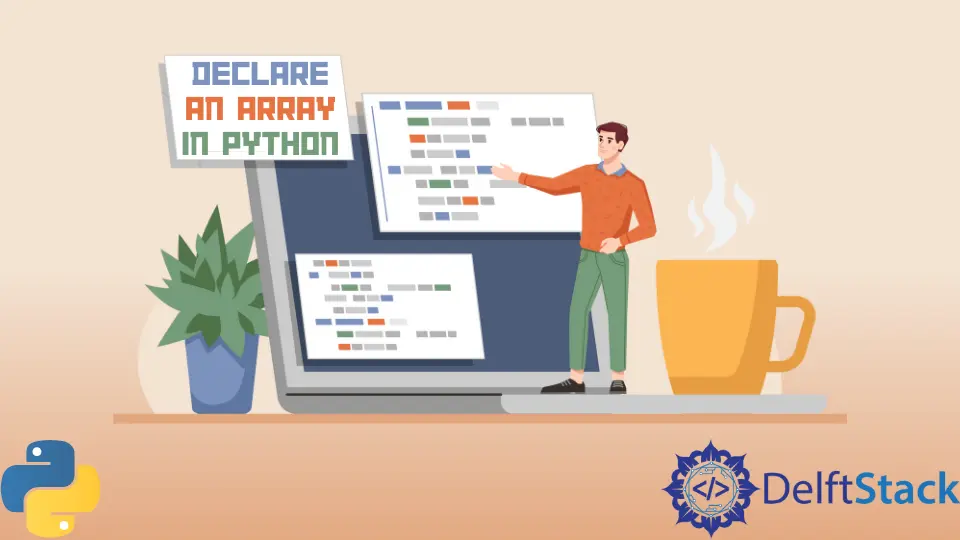
This tutorial will enlist different methods to declare an array in Python. The array concept is usually mixed with the concept of a list, as lists can contain different types of values. The concept of an array is rarely used as it provides C language type functionalities.
This tutorial will explain how we can declare the list and arrays in Python.
Python Declaration of Arrays as Represented by the Class list
The concept of the array is often mixed with the concept of the list. A list is a collection of heterogeneous items with dynamic length, in which the data types can be different. However, an array is a collection of objects that deals explicitly with the homogenous collection of items. In simple terms, arrays are represented by the list
class, and they can be assigned simply with the assignment operator with square brackets on the other side.
The items of the list can be accessed by simply passing the required index. For example, temp[2]
will access the third item. An item can be appended using the temp.append()
module with the item to be appended at the end. Moreover, the insert(x, y)
function can be used to insert an element at the required index. The index can be provided with x
value, and the value will be provided with y
value. The index value can be negative also to access the elements in reverse order.
The example code below demonstrates how to define an array as a list in Python and what functionalities can be used.
temp = [1, 2, 3, "s", 5]
print(temp[-2])
temp.insert(4, 6)
print(temp[-2])
temp.append("f")
print(temp)
Output:
s
6
[1, 2, 3, 's', 6, 5, 'f']
Declare an Array in Python by Importing the array
Module
If you really want to initialize an array with the capability of containing only homogenous elements, the array
module is imported from the array
library. The array is defined with the parentheses and essentially two parameters. The first parameter is a type code
that defines the type of the elements, and another parameter is the list of elements enclosed in the square brackets. There’s no need to declare things in Python pre-requisitely.
An element of the array can be accessed by the array.index(x)
function where x
is the index of the array. Similarly, the insertion operation can also be performed on the array with the array.insert(i,x)
function, where i
is the index and x
is the value to be inserted.
An example code is given below to demonstrate how an array is created in Python.
from array import array
temp_array = array("i", [1, 2, 3, 4, 5])
print(temp_array.index(2))
temp_array.insert(2, 6)
print(temp_array)
Output:
1
array('i', [1, 2, 6, 3, 4, 5])
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn