How to Declare 3D Array in Python
- Declare 3D List Using List Comprehensions in Python
- Declare 3D List Using Multiplication Method in Python
-
Declare 3D Array Using the
NumPy
Package in Python
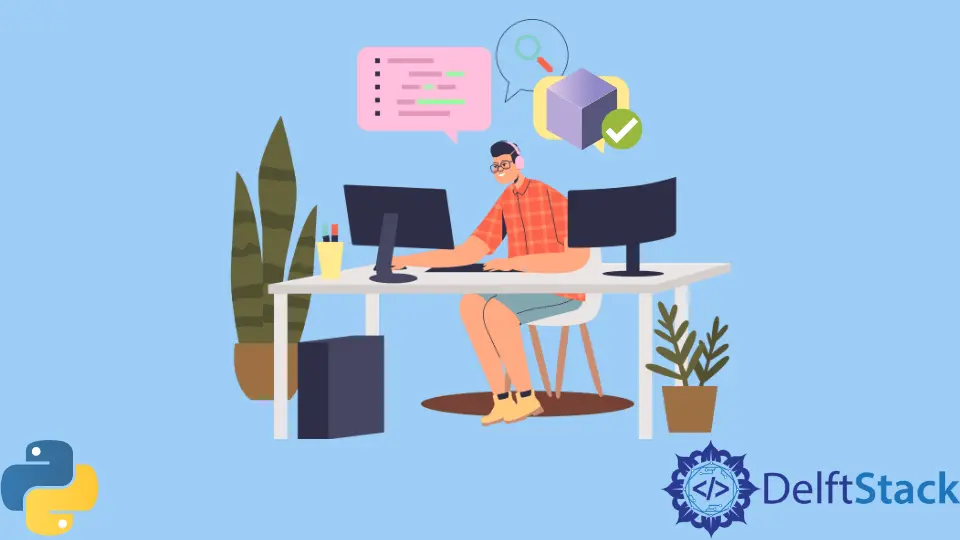
In this tutorial, we will discuss methods to declare 3-dimensional arrays in Python.
Declare 3D List Using List Comprehensions in Python
As far as the basic functionality is concerned, the lists do the same job as arrays in Python. The list comprehension is a way of performing complex operations on lists. List comprehensions can also be used to declare a 3D array. The following code example shows us how we can use the list comprehensions to declare a 3D array in Python.
n = 3
distance = [[[0 for k in range(n)] for j in range(n)] for i in range(n)]
print(distance)
Output:
[[[0, 0, 0], [0, 0, 0], [0, 0, 0]], [[0, 0, 0], [0, 0, 0], [0, 0, 0]], [[0, 0, 0], [0, 0, 0], [0, 0, 0]]]
In the above code, we first declare the number of dimensions and then initialize a list of n
dimensions using list comprehensions.
Declare 3D List Using Multiplication Method in Python
The list comprehension method works fine, but it is a bit code extensive. If we want to minimize our code, we can use another approach called the multiplication method. The following code example shows us how to use the multiplication method to declare a 3D array in Python.
distance = [[[0] * n] * n] * n
print(distance)
Output:
[[[0, 0, 0], [0, 0, 0], [0, 0, 0]], [[0, 0, 0], [0, 0, 0], [0, 0, 0]], [[0, 0, 0], [0, 0, 0], [0, 0, 0]]]
The above code does the same thing as list comprehensions but reduces the code drastically.
Declare 3D Array Using the NumPy
Package in Python
If we want to perform some operations specifically on arrays in Python, we had better use the NumPy
package. It is a package specifically designed to work with arrays in Python.
NumPy
is an external package and does not come pre-installed with Python. We need to install it before using it. The command to install the NumPy
package is given below.
pip install numpy
The following code example shows how we can declare a 3-dimensional array in Python using the NumPy
package.
import numpy as np
i = 3
j = 3
k = 3
new_array = np.zeros((i, j, k))
print(new_array)
Output:
[[[0. 0. 0.]
[0. 0. 0.]
[0. 0. 0.]]
[[0. 0. 0.]
[0. 0. 0.]
[0. 0. 0.]]
[[0. 0. 0.]
[0. 0. 0.]
[0. 0. 0.]]]
In the above code, we first declare the number of elements in each dimension of the array with i
, j
, and k
. After that, we pass these dimensions to np.zeros()
to initialize a 3D array. The np.zeros()
method gives us an array and fills every element with a 0
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python