How to Print Data in Tabular Format in Python
-
Use the
format()
Function to Print Data in Table Format in Python -
Use the
tabulate
Module to Print Data in Table Format in Python -
Use the
pandas.DataFrame()
Function to Print Data in Table Format in Python
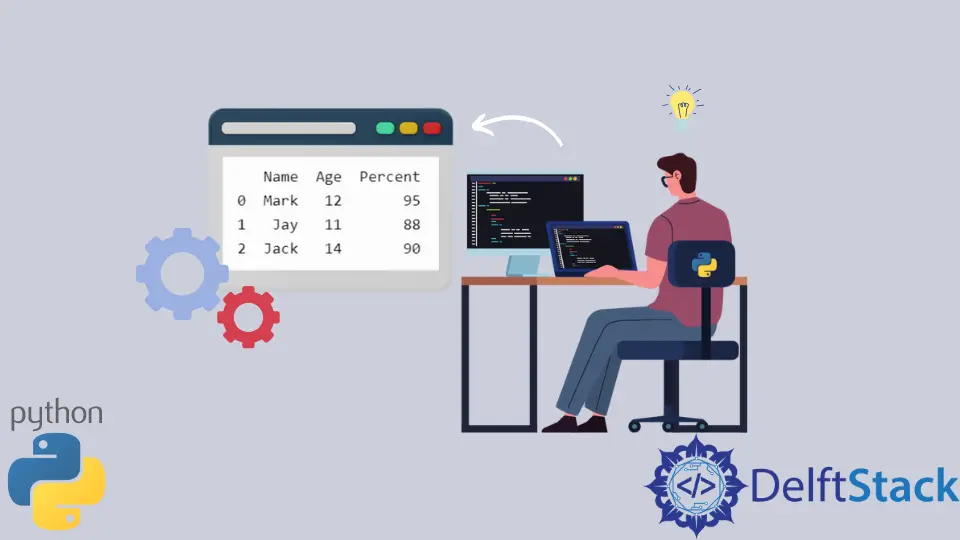
Lists can store multiple elements in a specific order. However, when we print a list it can be a little unclear if we are working with data formatted in rows. Data from lists can also be printed in tabular format. This way, the data, when viewed, will be neat and organized since everything is arranged beautifully into rows and columns.
This tutorial will introduce how to print data from a list collection in a table format.
Use the format()
Function to Print Data in Table Format in Python
Python allows us to perform efficient string-formatting using the format()
function. It gives us the freedom to make sure we get the output in our desired format.
For showing data in a tabular format, we specify the spaces efficiently between the columns and print the data from the list in the same format.
For example,
d = [["Mark", 12, 95], ["Jay", 11, 88], ["Jack", 14, 90]]
print("{:<8} {:<15} {:<10}".format("Name", "Age", "Percent"))
for v in d:
name, age, perc = v
print("{:<8} {:<15} {:<10}".format(name, age, perc))
Output:
Name Age Percent
Mark 12 95
Jay 11 88
Jack 14 90
Use the tabulate
Module to Print Data in Table Format in Python
The tabulate
module has methods available to print data in simple-elegant table structures.
We only have to specify the data, and the column names to the tabulate()
function from this module, and it will do the rest.
For example,
from tabulate import tabulate
d = [["Mark", 12, 95], ["Jay", 11, 88], ["Jack", 14, 90]]
print(tabulate(d, headers=["Name", "Age", "Percent"]))
Output:
Name Age Percent
------ ----- ---------
Mark 12 95
Jay 11 88
Jack 14 90
Note that there are other modules also available in Python which can print data in different table styles. Some of them are PrettyTable
, termtable
, texttable
, and more.
Use the pandas.DataFrame()
Function to Print Data in Table Format in Python
The pandas
library allows us to create DataFrames in Python. These DataFrames are frequently used to store datasets and enable efficient handling of the data stored in them. We can also perform various types of operations on the DataFrame.
We can very easily create a DataFrame using data from a list and print it as shown below.
import pandas as pd
d = [["Mark", 12, 95], ["Jay", 11, 88], ["Jack", 14, 90]]
df = pd.DataFrame(d, columns=["Name", "Age", "Percent"])
print(df)