How to Create a List of Range of Dates in Python
- Using Pandas to Create a List of Range of Dates in Python
- Create the List of Range of Dates Manually in Python
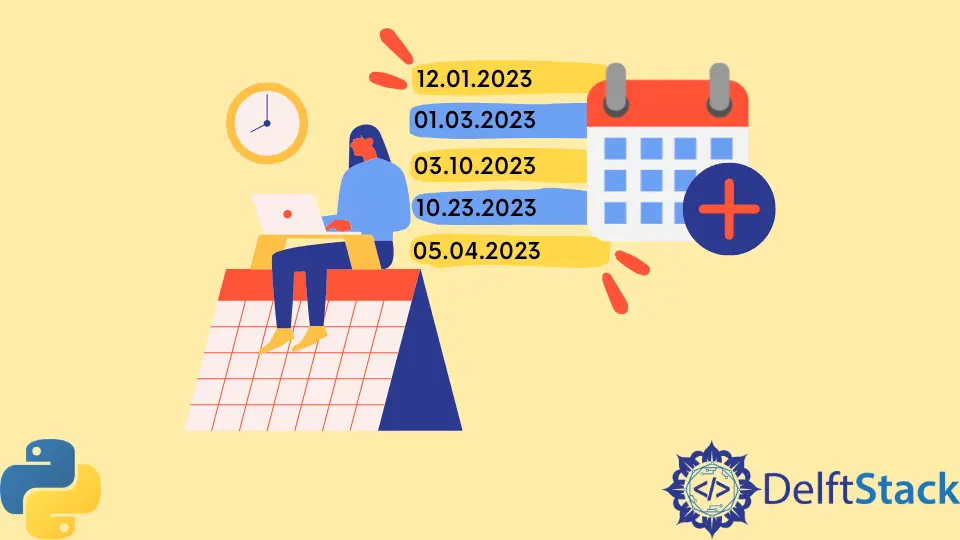
We will introduce some methods for generating a list of a range of dates. One way to do so is to use the pandas
library. Pandas has direct support for achieving the task. The other way is to design your own logic manually using for loop to generate the dates.
We will look at some examples like creating the list of a range of dates by input start date and k days after that or we can input start date and end date.
Using Pandas to Create a List of Range of Dates in Python
We will use the date_range()
function of pandas in which we will pass the start date and the number of days after that (known as periods). Here we have also used the datetime
library to format the date so that we can output the date in this format DD-MM-YY
. We can also define a time stamp along with the date.
Example Codes:
In this example, we are passing the start date in the format of DD-MM-YY
and periods. So in output, we can see the list of a range of days starting from the start date and the number of periods
after that.
# python 3.x
import datetime
import pandas as pd
start = datetime.datetime.strptime("01-12-2021", "%d-%m-%Y")
date_generated = pd.date_range(start, periods=5)
print(date_generated.strftime("%d-%m-%Y"))
Output:
Index(['01-12-2021', '02-12-2021', '03-12-2021', '04-12-2021', '05-12-2021'], dtype='object')
Now we will look at another example in which we will pass the start date
and end date
. The program will output the range of dates between these two dates.
import datetime
import pandas as pd
start = datetime.datetime.strptime("01-12-2021", "%d-%m-%Y")
end = datetime.datetime.strptime("07-12-2021", "%d-%m-%Y")
date_generated = pd.date_range(start, end)
print(date_generated.strftime("%d-%m-%Y"))
Output:
Index(['01-12-2021', '02-12-2021', '03-12-2021', '04-12-2021', '05-12-2021',
'06-12-2021', '07-12-2021'],
dtype='object')
Create the List of Range of Dates Manually in Python
With the help of for loop and timedelta()
, we can generate the list manually. timedelta()
is a function defined under the datetime
library. Here delta means difference. With its help, we can add or subtract delta with a date to get the next or previous date, respectively. Let’s say we have a date 01-12-2021. Now, if we add 1 to it, our date would be 02-12-2021. We will use that logic in for loop. For loop is iterated, and we will add the variable with the start date to get the next date, and then we append the currently generated date to our list until we get our complete date range.
Example Code:
# python 3.x
import datetime
start = datetime.date(2021, 12, 10)
periods = 5
daterange = []
for day in range(periods):
date = (start + datetime.timedelta(days=day)).isoformat()
daterange.append(date)
print(daterange)
Output:
['2021-12-10', '2021-12-11', '2021-12-12', '2021-12-13', '2021-12-14']
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn