How to Count Elements in List Python
-
Using the
count()
Method - Using a Dictionary to Count Elements
- Using the Counter Class from Collections
- Conclusion
- FAQ
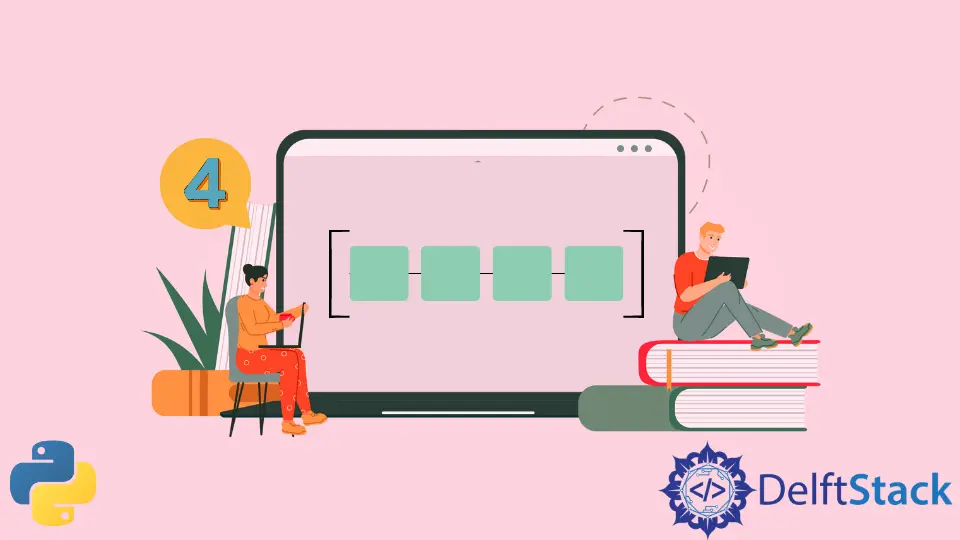
Counting elements in a list is a fundamental task in Python programming that every developer should master. Whether you’re analyzing data, tracking occurrences, or simply curious about the contents of your list, knowing how to count elements efficiently can save you time and effort.
In this tutorial, we’ll explore various methods to count elements in a list using Python, making it easy for you to choose the right approach for your needs. From the built-in count()
method to utilizing collections, we’ll cover it all. Let’s dive in and enhance your Python skills!
Using the count()
Method
One of the simplest ways to count elements in a list is by using the built-in count()
method. This method allows you to count the occurrences of a specific element in the list.
my_list = [1, 2, 3, 2, 1, 4, 2]
count_of_twos = my_list.count(2)
print(count_of_twos)
Output:
3
The count()
method is straightforward and efficient for counting specific elements. In the example above, we created a list named my_list
containing several integers, including the number 2
. By calling my_list.count(2)
, we retrieve the total number of times 2
appears in the list, which is 3
. This method is particularly useful when you only need to check the frequency of a single element without traversing the entire list manually.
However, if you need to count multiple elements or get a frequency distribution of all elements, this method may not be the most efficient choice. It requires separate calls for each element you want to count, which can become cumbersome with larger datasets.
Using a Dictionary to Count Elements
For counting all unique elements in a list, using a dictionary is a more efficient approach. This method allows you to create a frequency dictionary that maps each unique element to its count.
my_list = [1, 2, 3, 2, 1, 4, 2]
element_count = {}
for element in my_list:
if element in element_count:
element_count[element] += 1
else:
element_count[element] = 1
print(element_count)
Output:
{1: 2, 2: 3, 3: 1, 4: 1}
In this example, we initialize an empty dictionary called element_count
. We then loop through each element in the list my_list
. If the element is already a key in the dictionary, we increment its count. If not, we add it to the dictionary with a count of 1
. The result is a dictionary that shows how many times each unique element appears in the list. This method is efficient and scalable, especially when dealing with larger lists, as it only requires a single pass through the list.
Using the Counter Class from Collections
Python’s collections
module provides a powerful tool called Counter
, which simplifies the process of counting elements in a list. This class is designed specifically for counting hashable objects and returns a dictionary-like object.
from collections import Counter
my_list = [1, 2, 3, 2, 1, 4, 2]
element_count = Counter(my_list)
print(element_count)
Output:
Counter({2: 3, 1: 2, 3: 1, 4: 1})
By importing Counter
from the collections
module, we can easily count the elements in our list. When we pass my_list
to Counter
, it automatically counts the occurrences of each element and returns a Counter
object. The output shows how many times each element appears, similar to a dictionary, but with a more intuitive representation. This method is highly efficient and often preferred for its simplicity and readability, especially when working with larger datasets.
Conclusion
Counting elements in a list is a vital skill for any Python programmer. In this tutorial, we explored three effective methods: using the built-in count()
method, employing a dictionary for frequency counting, and utilizing the Counter
class from the collections
module. Each method has its strengths and is suitable for different scenarios. By mastering these techniques, you can efficiently analyze data and gain insights from your lists. Keep practicing, and you’ll find that counting elements becomes second nature in your Python programming journey!
FAQ
-
How do I count all elements in a list?
You can use a dictionary or the Counter class from the collections module to count all unique elements in a list. -
Can I count strings in a list using the count() method?
Yes, the count() method works with any data type, including strings.
-
Is the Counter class more efficient than a dictionary for counting?
Yes, the Counter class is optimized for counting and provides a more concise syntax. -
What happens if I try to count an element that is not in the list?
The count() method will return 0 if the element is not found. -
Can I count elements in a nested list?
You would need to flatten the nested list first before counting the elements.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python