How to Copy List in Python
-
Copy List in Python Using the
copy()
Method - Copy List in Python Using the Slicing Method
-
Copy List in Python Using the
list()
Function - Copy List in Python Using the List Comprehension Method
-
Copy List in Python Using the
copy.copy()
Method - Python List Deep Copy vs Shallow Copy
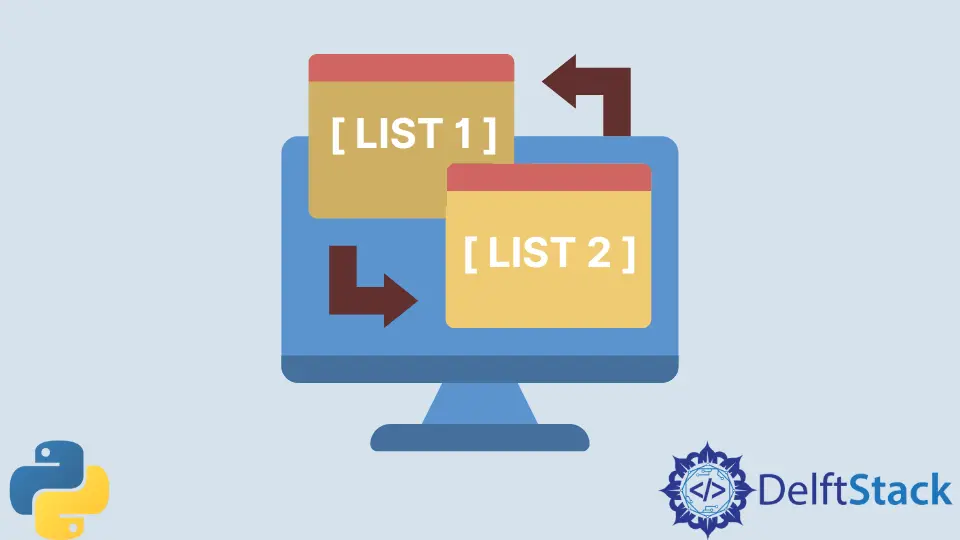
A list is a flexible data type available in Python and is enclosed in square brackets []
with comma-separated values. The values in the list can be different data types. List indexing starts at 0, and it can be concatenated and sliced. List values can be copied as a new list value using different built-in functions.
This article will introduce different methods to copy a list in Python.
Copy List in Python Using the copy()
Method
The most direct way to copy a Python list is through the copy()
method. We can find a scenario where we want to make a copy of the list. The equals sign =
can be used to construct a duplicate of a list. The new list will, however, be linked to the existing one. It means that the new list will also be altered if you update the original list. The new list refers to an item similar to the old list.
A copy of an existing list is generated by the list copy()
method. The copy()
method does not take any parameters.
Let’s take an example of fruit names as a Python list. The new list will copy all the fruit names that exist in the previous list. The example code is as follows.
fruits_spring = ["carrots", "kiwi", "grapes", "cherry"]
fruits_summer = fruits_spring.copy()
print(fruits_summer)
Output:
['carrots', 'kiwi', 'grapes', 'cherry']
Copy List in Python Using the Slicing Method
In Python, slicing is a method that we use to extract objects from a list. We can retrieve individual objects, several items, or all items contained inside a list using the slicing method.
Let’s use the above example to illustrate how we can copy a list using slicing.
The complete example code is given below.
fruits_spring = ["carrots", "kiwi", "grapes", "cherry"]
fruits_summer = fruits_spring[:]
print(fruits_summer)
Output:
['carrots', 'kiwi', 'grapes', 'cherry']
It uses the cloning form, a colon enclosed inside square brackets ([:]). This process generates a replica of the old list.
Copy List in Python Using the list()
Function
We can also construct a copy of a list in Python using the list()
function. The list()
function takes one parameter: the object you would like to convert to a list.
Let’s use the example of our fruit stand to demonstrate this approach.
fruits_spring = ["carrots", "kiwi", "grapes", "cherry"]
fruits_summer = list(fruits_spring)
print(fruits_summer)
Output:
['carrots', 'kiwi', 'grapes', 'cherry']
Copy List in Python Using the List Comprehension Method
This approach uses list comprehension. It iterates through all the existing list elements by the for
loop and adds the new list elements.
The complete example code is given as:
fruits_spring = ["carrots", "kiwi", "grapes", "cherry"]
fruits_summer = [i for i in fruits_spring]
print(fruits_summer)
Output:
['carrots', 'kiwi', 'grapes', 'cherry']
Copy List in Python Using the copy.copy()
Method
We can also use the copy()
method in the copy
module to copy a Python list.
The complete example code is given as:
import copy
fruits_spring = ["carrots", "kiwi", "grapes", "cherry"]
fruits_summer = copy.copy(fruits_spring)
print(fruits_summer)
Output:
['carrots', 'kiwi', 'grapes', 'cherry']
Python List Deep Copy vs Shallow Copy
All above-introduced methods are shallow copies. The shallow copy means if the list element is mutable and this element is modified in the original list, this modification will also be reflected in the copied list. Shallow copy only copies the pointer or the reference to the list elements.
original_list = [["carrots", "apple"], "kiwi", "grapes", "cherry"]
print("The original list is")
print(original_list)
copied_list = original_list.copy()
original_list[0][0] = "banana"
original_list[0][1] = "apple"
original_list[1] = "orange"
print("The original list after modification is")
print(original_list)
print("The copied list is")
print(copied_list)
Output:
The original list is
[['carrots', 'apple'], 'kiwi', 'grapes', 'cherry']
The original list after modification is
[['banana', 'apple'], 'orange', 'grapes', 'cherry']
The copied list is
[['banana', 'apple'], 'kiwi', 'grapes', 'cherry']
The first element of the original list is also a list, which is mutable. When we change its content, the corresponding element in the copied list is modified. Meanwhile, the original list’s second element is an immutable object, so its change will not be reflected in the copied list.
Deep copy, in contrast to the shallow copy, copies the objects in the original list recursively to the new list. So the modification in the original list will not be reflected in the copied list. The deepcopy()
method of the copy
module performs the deep copy operation.
import copy
original_list = [["carrots", "apple"], "kiwi", "grapes", "cherry"]
print("The original list is")
print(original_list)
copied_list = copy.deepcopy(original_list)
original_list[0] = ["banana", "apple"]
original_list[1] = "orange"
print("The original list after modification is")
print(original_list)
print("The copied list is")
print(copied_list)
Output:
The original list is
[['carrots', 'apple'], 'kiwi', 'grapes', 'cherry']
The original list after modification is
[['banana', 'apple'], 'orange', 'grapes', 'cherry']
The copied list is
[['carrots', 'apple'], 'kiwi', 'grapes', 'cherry']
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python