在 Python 中複製列表
-
在 Python 中使用
copy()
方法複製列表 - 在 Python 中使用切片方法複製列表
-
在 Python 中使用
list()
函式複製列表 - 在 Python 中使用列表推導方法複製列表
-
在 Python 中使用
copy.copy()
方法複製列表 - Python 列表深層複製與淺層複製
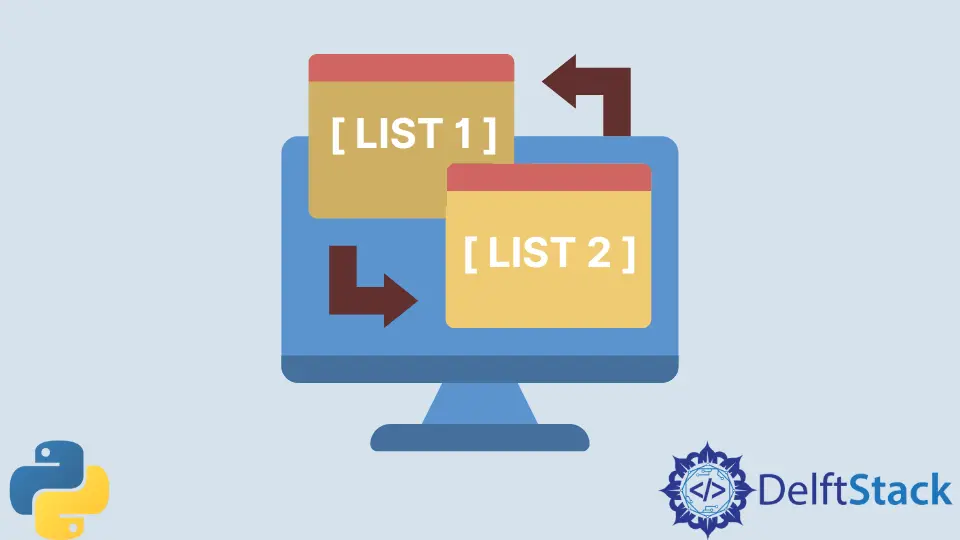
列表是 Python 中一個靈活的資料型別,用方括號 []
括起來,用逗號分隔的值。列表中的值可以是不同的資料型別。列表索引從 0 開始,它可以被連線和分片。可以使用不同的內建函式將列表值複製為新的列表值。
本文將介紹在 Python 中複製列表的不同方法。
在 Python 中使用 copy()
方法複製列表
複製 Python 列表最直接的方法是通過 copy()
方法。我們可以找到一個場景,我們要對列表進行復制。等號 =
可以用來構造一個列表的副本。然而,新的列表將與現有的列表相連線。這意味著如果你更新原始列表,新的列表也會被改變。新列表指的是與舊列表相似的專案。
現有列表的副本是由列表 copy()
方法生成的。copy()
方法不接受任何引數。
讓我們以水果名稱為 Python 列表為例。新的列表將複製前一個列表中存在的所有水果名稱。示例程式碼如下。
fruits_spring = ["carrots", "kiwi", "grapes", "cherry"]
fruits_summer = fruits_spring.copy()
print(fruits_summer)
輸出:
['carrots', 'kiwi', 'grapes', 'cherry']
在 Python 中使用切片方法複製列表
在 Python 中,切片是我們用來從列表中提取物件的一種方法。我們可以使用切片方法檢索單個物件、幾個專案或列表中包含的所有專案。
讓我們用上面的例子來說明我們如何使用切片方法複製一個列表。
完整的示例程式碼如下。
fruits_spring = ["carrots", "kiwi", "grapes", "cherry"]
fruits_summer = fruits_spring[:]
print(fruits_summer)
輸出:
['carrots', 'kiwi', 'grapes', 'cherry']
它使用的是克隆形式,即方括號內的冒號([:])。這個過程會生成一箇舊列表的副本。
在 Python 中使用 list()
函式複製列表
我們也可以在 Python 中使用 list()
函式來構造一個列表的副本。list()
函式只需要一個引數:你想轉換為列表的物件。
讓我們用水果攤的例子來演示這種方法。
fruits_spring = ["carrots", "kiwi", "grapes", "cherry"]
fruits_summer = list(fruits_spring)
print(fruits_summer)
輸出:
['carrots', 'kiwi', 'grapes', 'cherry']
在 Python 中使用列表推導方法複製列表
這種方法使用列表推導。它通過 for
迴圈迭代所有現有的列表元素,並新增新的列表元素。
完整的示例程式碼給出如下。
fruits_spring = ["carrots", "kiwi", "grapes", "cherry"]
fruits_summer = [i for i in fruits_spring]
print(fruits_summer)
輸出:
['carrots', 'kiwi', 'grapes', 'cherry']
在 Python 中使用 copy.copy()
方法複製列表
我們也可以使用 copy
模組中的 copy()
方法來複制一個 Python 列表。
完整的示例程式碼給出如下。
import copy
fruits_spring = ["carrots", "kiwi", "grapes", "cherry"]
fruits_summer = copy.copy(fruits_spring)
print(fruits_summer)
輸出:
['carrots', 'kiwi', 'grapes', 'cherry']
Python 列表深層複製與淺層複製
以上介紹的方法都是淺層複製。淺層複製的意思是如果列表元素是可變的,而這個元素在原列表中被修改,這個修改也會反映在複製的列表中。淺層複製只複製列表元素的指標或引用。
original_list = [["carrots", "apple"], "kiwi", "grapes", "cherry"]
print("The original list is")
print(original_list)
copied_list = original_list.copy()
original_list[0][0] = "banana"
original_list[0][1] = "apple"
original_list[1] = "orange"
print("The original list after modification is")
print(original_list)
print("The copied list is")
print(copied_list)
輸出:
The original list is
[['carrots', 'apple'], 'kiwi', 'grapes', 'cherry']
The original list after modification is
[['banana', 'apple'], 'orange', 'grapes', 'cherry']
The copied list is
[['banana', 'apple'], 'kiwi', 'grapes', 'cherry']
原列表的第一個元素也是一個列表,它是可變的。當我們改變它的內容時,複製列表中相應的元素也會被修改。同時,原列表的第二個元素是一個不可變的物件,所以它的變化不會反映在複製的列表中。
深層複製與淺層複製相反,它將原列表中的物件遞迴複製到新列表中。所以原列表中的修改不會反映在複製的列表中。copy
模組的 deepcopy()
方法執行深層複製操作。
import copy
original_list = [["carrots", "apple"], "kiwi", "grapes", "cherry"]
print("The original list is")
print(original_list)
copied_list = copy.deepcopy(original_list)
original_list[0] = ["banana", "apple"]
original_list[1] = "orange"
print("The original list after modification is")
print(original_list)
print("The copied list is")
print(copied_list)
輸出:
The original list is
[['carrots', 'apple'], 'kiwi', 'grapes', 'cherry']
The original list after modification is
[['banana', 'apple'], 'orange', 'grapes', 'cherry']
The copied list is
[['carrots', 'apple'], 'kiwi', 'grapes', 'cherry']