How to Convert MP3 to WAV in Python
-
Use the
pydub
Module to Convert MP3 to WAV in Python -
Use
ffmpeg
Directly to Convert MP3 to WAV -
Use the
subprocess
Module to Convert MP3 to WAV in Python - Conclusion
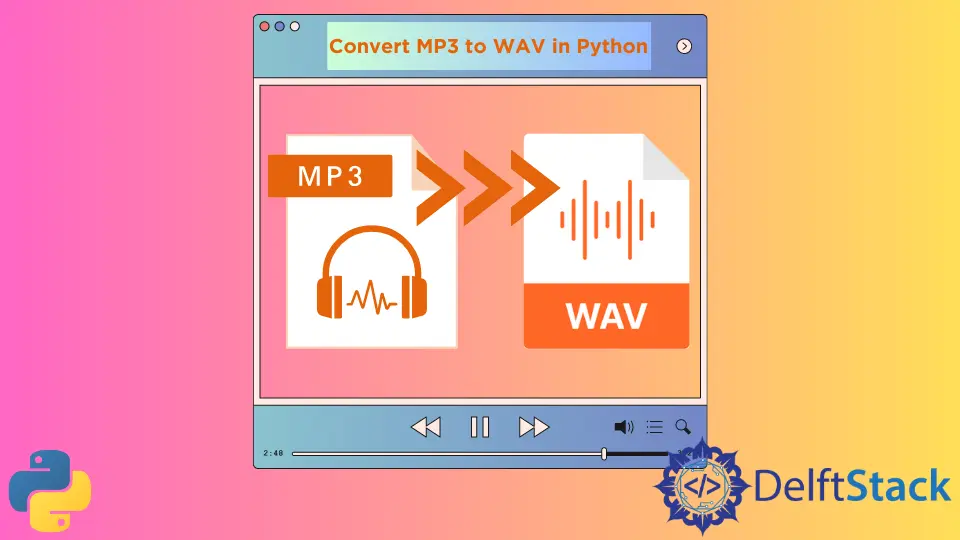
In the realm of audio processing, converting audio files from one format to another is a common practice. It’s particularly prevalent to convert MP3 files to WAV, given the distinct characteristics and use cases of these two formats. MP3, known for its compression efficiency, is widely used for music and podcasts where file size is a critical factor. On the other hand, WAV offers uncompressed audio, making it a preferred choice for professional audio editing and applications demanding high-quality sound.
In this article, we will explore different methods to convert MP3 to WAV in Python, catering to intermediate Python developers familiar with basic audio formats. We will delve into practical examples using libraries like pydub
, ffmpeg
, and the subprocess
module.
Use the pydub
Module to Convert MP3 to WAV in Python
pydub
is a high-level audio library that makes it easy to work with different audio file formats. One of its classes, AudioSegment
, is particularly useful for reading and manipulating audio files.
pydub
can be easily installed using pip
. The installation process is the same across all major operating systems.
pip install pydub
Here’s a step-by-step breakdown using pydub
.
from pydub import AudioSegment
# Specify the source and destination file paths
src = "transcript.mp3"
dst = "test.wav"
# Load the MP3 file
audSeg = AudioSegment.from_mp3(src)
# Convert and export as WAV
audSeg.export(dst, format="wav")
Explanation:
AudioSegment
is a class inpydub
that allows you to import and manipulate audio files easily. It abstracts the complex details of audio processing, providing a user-friendly API.from_mp3(src)
is a method that creates anAudioSegment
instance from an MP3 file.src
is the path to the MP3 file.export(dst, format="wav")
is a method that exports the audio segment to a file specified bydst
, converting it to the format specified by theformat
parameter.
Use ffmpeg
Directly to Convert MP3 to WAV
ffmpeg
is a powerful multimedia framework capable of dealing with various audio and video formats. It can be invoked directly from Python using the os.system()
method.
The installation of ffmpeg
varies depending on the operating system.
-
For Windows:
- Download the executable file from the official ffmpeg website .
- Add the bin folder containing
ffmpeg.exe
to the system’s PATH.
-
For macOS:
- Use Homebrew to install
ffmpeg
:
brew install ffmpeg
- Use Homebrew to install
-
For Linux:
- Use the package manager to install
ffmpeg
. For Ubuntu and Debian-based systems, use:
sudo apt install ffmpeg
- Use the package manager to install
Here’s how to employ ffmpeg
effectively.
import os
# Construct the ffmpeg command
command = "ffmpeg -i transcript.mp3 test.wav"
# Execute the command
os.system(command)
Explanation:
ffmpeg
is invoked by constructing a command string that specifies the input file (-i transcript.mp3
) and the output file (test.wav
).os.system(command)
is used to execute the command in the system’s terminal. Thecommand
is a string that contains the completeffmpeg
command.
Use the subprocess
Module to Convert MP3 to WAV in Python
The subprocess
module provides more flexibility and control over the invocation of external processes, making it a preferred choice for complex applications.
import subprocess
# Invoke ffmpeg using subprocess
subprocess.call(["ffmpeg", "-i", "transcript.mp3", "test.wav"])
Explanation:
- The
subprocess.call()
function is used to run the command described by args. Here, args is a sequence of program arguments, where the program to be executed is the first item in the args sequence. - The list
["ffmpeg", "-i", "transcript.mp3", "test.wav"]
contains theffmpeg
command and its arguments, similar to how they would be written in the terminal.
Conclusion
Each method to convert MP3 to WAV in Python comes with its unique advantages. pydub
offers simplicity and abstraction, making it a go-to for quick implementations. Direct use of ffmpeg
caters to those comfortable with command-line operations and looking for granular control. The subprocess
module bridges the gap, offering programmatic access to command-line functionalities within a Python script.