How to Process Real-Time Audio in Python
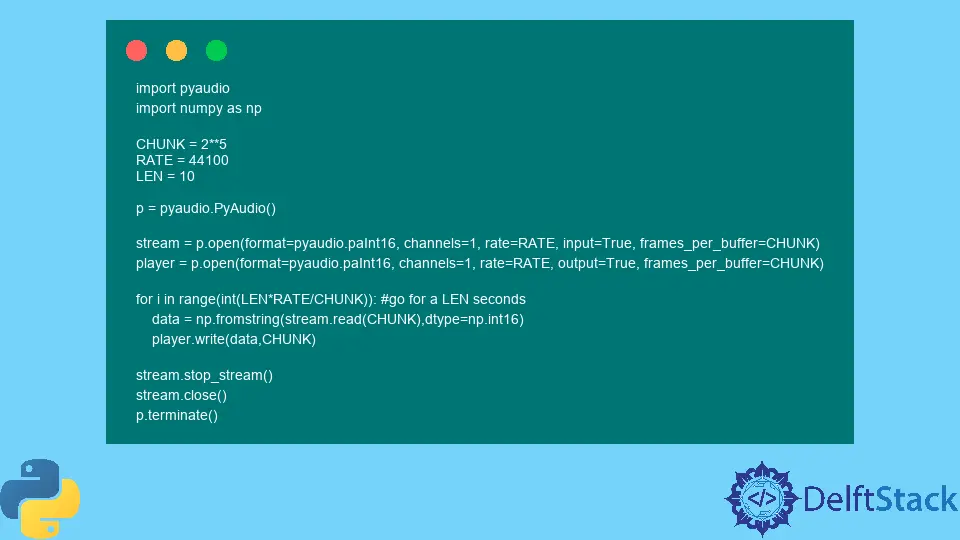
Python is a straightforward programming language that is perfect for audio-processing tasks. Python’s built-in libraries and easy-to-use syntax make it easy to develop audio processing applications quickly.
One of the great specialties of Python is that it can be used for real-time audio processing. It means you can take an audio signal, process it, and immediately hear the results.
It is perfect for applications like live music processing, where you want to be able to tweak the sound in real time.
Python also has several powerful libraries for audio processing, such as the popular PyAudio
library. It makes it easy to develop complex audio applications with Python.
So if you’re looking for a powerful and easy-to-use language for audio processing, Python is a perfect choice.
Real-Time Audio Processing in Python
Real-time audio processing python manipulates and extracts information from audio signals in real-time. This can be done using various programming languages.
Still, Python is one of the most popular languages for real-time audio processing due to its ease of use and powerful libraries. Python has many applications for real-time audio processing, such as speech recognition, audio effects, and sound classification.
Python makes it relatively easy to develop these applications, as many libraries can be used for signal processing and machine learning. It is necessary to convert the signals to digital form before performing real-time audio processing in Python.
This can be done using an audio interface, which converts the analog signal into a digital signal. So, once the signal is digital, it can be manipulated using various programming techniques.
Create Real-Time Audio Processing in Python
There are a few ways to create real-time audio processing in Python. The common way is to use the built-in audio processing libraries with the python installation.
One of Python’s most popular techniques for real-time audio processing is to use the FFT (Fast Fourier Transform) algorithm. This algorithm can extract information from the signal, such as the frequency components.
The FFT algorithm is very fast, which makes it well-suited for real-time applications.
Another popular technique is the Mel Frequency Cepstral Coefficients (MFCCs) algorithm. This algorithm is commonly used for speech recognition, as it can extract information about the human voice.
The MFCCs algorithm is also high-speed, which makes it suitable for real-time applications. Many other algorithms can be used for real-time audio processing in Python, such as the Linear Predictive Coding (LPC) algorithm.
However, the FFT and MFCC algorithms are the most commonly used.
However, these libraries are not always well-suited for real-time audio processing. So it is also possible to use other libraries explicitly designed for real-time audio processing.
Python’s most common library for real-time audio processing is PyAudio
, which provides a straightforward interface for accessing the sound card and processing audio in real time.
PyAudio
is available for Windows, Linux, and OS X. It can install using the pip package manager.
But before using PyAudio
, don’t forget to install it on your local machine using the pip
command.
pip install pyaudio
Example Code:
import pyaudio
import numpy as np
CHUNK = 2 ** 5
RATE = 44100
LEN = 10
p = pyaudio.PyAudio()
stream = p.open(
format=pyaudio.paInt16, channels=1, rate=RATE, input=True, frames_per_buffer=CHUNK
)
player = p.open(
format=pyaudio.paInt16, channels=1, rate=RATE, output=True, frames_per_buffer=CHUNK
)
for i in range(int(LEN * RATE / CHUNK)): # go for a LEN seconds
data = np.fromstring(stream.read(CHUNK), dtype=np.int16)
player.write(data, CHUNK)
stream.stop_stream()
stream.close()
p.terminate()
The above code example will generate a rough sound for a few seconds.
Conclusion
This article concludes that you can create real-time audio processing python. This article also gives you an idea about the algorithms for creating real-time audio processing.
You can also read about the Fast Fourier Transform (FFT) algorithm. It is an efficient way to compute a signal’s Discrete Fourier Transform (DFT).
On the other side, the Mel frequency cepstral coefficients (MFCCs) algorithm is a real-time audio processing technique used to extract features from an audio signal.
You can also get the code for creating audio processing by PyAudio
.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn