FFmpeg in Python Script
- Install the FFmpeg Python Package
- Use FFmpeg to Trim a Video in Python
- Use FFmpeg to Get the Width and Height of a Video in Python
- Use FFmpeg to Save the Thumbnail From a Video in Python
- Use FFmpeg to Flip a Video in Python
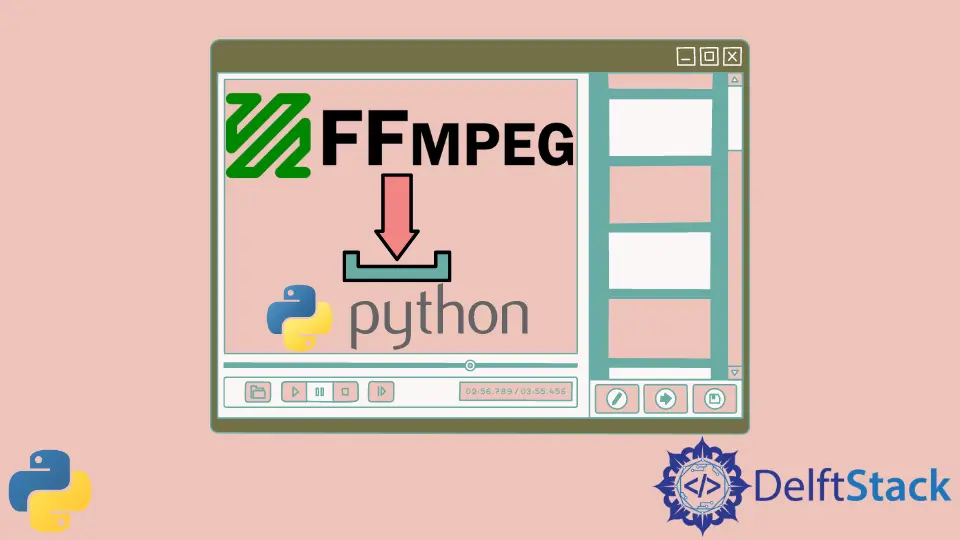
FFmpeg is short for Fast Forward Moving Picture Experts Group. It is an open-source project that provides tools such as ffmpeg
, ffplay
, and ffprobe
to deal with multimedia files.
FFmpeg is a command-line utility that helps to convert video/audio format, compress a video, extract audio from a video, create a GIF, cut a video, and more.
This tutorial will teach you to use FFMPEG commands in Python.
Install the FFmpeg Python Package
First, you have to install FFmpeg on your system. Open the command prompt as an administrator and run the following command to install FFmpeg using choco
.
choco install ffmpeg
Next, install the ffmpeg-python
package using the Python package manager tool pip
.
Run the following command in the prompt to install the package with pip
.
pip install ffmpeg-python
Output:
Successfully built ffmpeg
Installing collected packages: ffmpeg
Successfully installed ffmpeg-1.4
Use FFmpeg to Trim a Video in Python
Since we have already configured FFmpeg on our system, let’s use some FFmpeg commands to work with videos in Python.
The following example cuts the video Pencil.mp4
from 5s to 10s and saves it as output.mp4
.
import ffmpeg
video = ffmpeg.input("Pencil.mp4")
video = video.trim(start=5, duration=5)
video = ffmpeg.output(video, "output.mp4")
ffmpeg.run(video)
Use FFmpeg to Get the Width and Height of a Video in Python
The following example prints the width and height of a specified video in Python.
import ffmpeg
probe = ffmpeg.probe("output.mp4")
video = next(
(stream for stream in probe["streams"] if stream["codec_type"] == "video"), None
)
width = int(video["width"])
height = int(video["height"])
print("Width:", width)
print("Height:", height)
Output:
Width: 1280
Height: 720
Use FFmpeg to Save the Thumbnail From a Video in Python
You can also save the thumbnail from a video using FFmpeg in Python.
The following example generates the thumbnail of width 500px from time 4s in a video.
import ffmpeg
video = ffmpeg.input("Pencil.mp4", ss=4)
video = video.filter("scale", 500, -1)
video = ffmpeg.output(video, "output.png", vframes=1)
ffmpeg.run(video)
The height is automatically determined by the aspect ratio.
Output image:
Use FFmpeg to Flip a Video in Python
You can use ffmpeg.hflip()
to flip the video horizontally and ffmpeg.vflip()
to flip the video vertically in Python.
Flip the video horizontally:
import ffmpeg
video = ffmpeg.input("Pencil.mp4")
video = ffmpeg.hflip(video)
video = ffmpeg.output(video, "horizontal.mp4")
ffmpeg.run(video)
Flip the video vertically:
import ffmpeg
video = ffmpeg.input("Pencil.mp4")
video = ffmpeg.vflip(video)
video = ffmpeg.output(video, "vertical.mp4")
ffmpeg.run(video)
FFmpeg is a handy tool for performing different operations on multimedia files. It can quickly trim the video, change file format, extract audio, create GIFs, and more.
By this point, you should have clearly understood how to use FFmpeg commands in Python script. We hope you find this tutorial helpful.