How to Convert Datetime to String in Python
-
Use the
strftime()
Function to Convertdatetime
to String in Python -
Use the
format()
Function to Convertdatetime
to String in Python -
Use
f-strings
to Convertdatetime
to String in Python
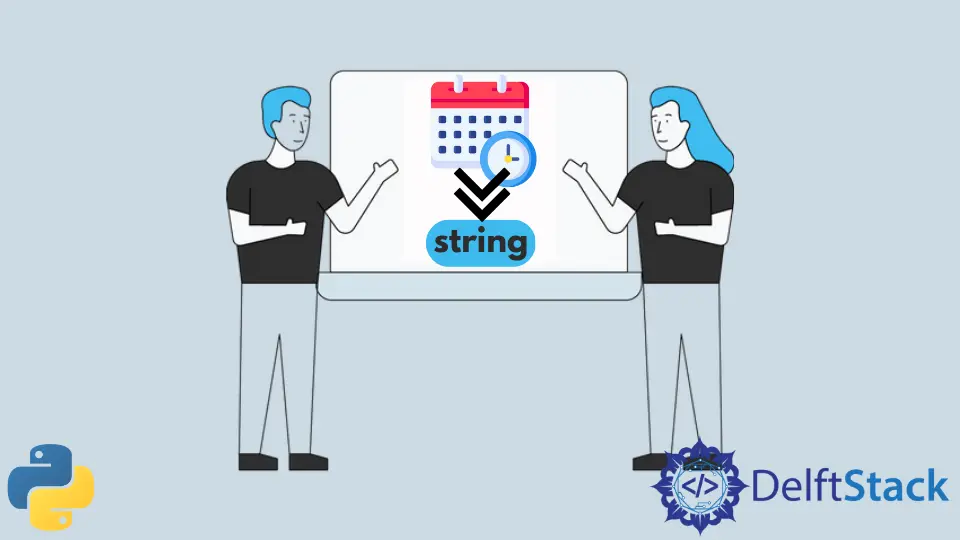
Python does not provide any default built-in data type to store Time and Date. However, the datetime
module can be imported into the Python code that is utilized to supply the programmer with classes capable of manipulating the date and time in Python.
This article demonstrates the different ways available to convert a datetime object to a String object in Python.
Use the strftime()
Function to Convert datetime
to String in Python
The strftime()
function uses different format codes to convert a datetime
object to a string
object. A formatted string is returned as the output after applying the strftime()
function on a datetime
object.
The several format codes that might be useful when using this conversion process are mentioned below.
%Y
: Formats to the value of theYear
of the given date.%m
: Formats to the value of theMonth
of the given date.%d
: Formats to the value of theDay
of the given date.%H
: Formats to the value of theHour
of the given time.%M
: Formats to the value of theMinute
of the given time.%S
: Formats to the value of theSecond
of the given time.
You may come across some of them in the example code of this article. The following snippet uses the strftime()
function to convert datetime to string in Python.
import datetime
x = datetime.datetime(2022, 7, 27, 0, 0)
x = x.strftime("%m/%d/%Y")
print(x)
Output:
07/27/2022
Use the format()
Function to Convert datetime
to String in Python
The format()
function is one of the several ways available to implement string formatting in Python. The format()
function can also convert a datetime
object into a string object.
The format()
function uses the same format codes mentioned above and can return a string. The following snippet uses the format()
function to convert datetime
to string in Python.
import datetime
x = datetime.datetime(2022, 7, 27, 0, 0)
print("{:%m/%d/%Y}".format(x))
Output:
07 / 27 / 2022
Use f-strings
to Convert datetime
to String in Python
Introduced in Python 3.6, f-strings
are the newest addition to the methods used to implement string formatting in Python. It is available and can be utilized in all the newer functions of Python.
f-strings
are more efficient than its other two peers, namely the %
sign and the str.format()
function, as it is more efficient and simpler to implement. Moreover, it helps implement the concept of string formatting in Python faster than the other two methods.
Let’s look at the code example below.
import datetime
x = datetime.datetime(2022, 7, 27, 0, 0)
print(f"{x:%m/%d/%Y}")
Output:
07/27/2022
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn