How to Get All Combinations of a List in Python
-
Use the
itertools.combinations()
Function to Get All Combinations of a List in Python -
Use the
itertools.combinations_with_replacement()
Function to Get All Combinations of a List in Python - Use Recursion and Backtracking to Get All Combinations of a List in Python
- Use a Recursive Generator Function to Get All Combinations of a List in Python
-
Create a User-Defined
powerset()
Function to Get All Combinations of a List in Python - Conclusion
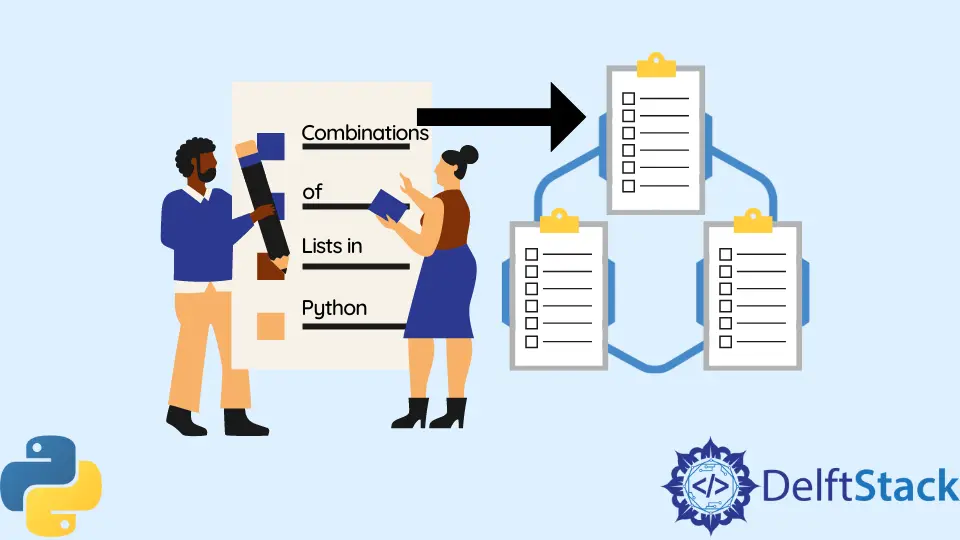
A combination determines the number of possible arrangements in a collection of elements. In a combination of elements, the elements are selected in an arbitrary order.
Getting all possible combinations of elements from a given list is not an uncommon problem in Python. It can be useful for several tasks, such as creating subsets, generating permutations, or exploring combinations to increase problem-solving efficiency.
In this tutorial, we will demonstrate the various approaches available to obtain all combinations of a list in Python.
Use the itertools.combinations()
Function to Get All Combinations of a List in Python
The function combinations(list_name, x)
from the itertools
module takes the list name and a number x
as the parameters and returns a list of tuples each of length x
containing all the possible combinations of one element in the list with the other elements.
For example:
from itertools import combinations
A = [10, 5, "Hi"]
temp = combinations(A, 2)
for i in list(temp):
print(i)
Output:
A sorted list will output the combination tuples in sorted order. A combination of one element in the list with itself is not possible using the combinations()
function.
Use the itertools.combinations_with_replacement()
Function to Get All Combinations of a List in Python
The function combinations_with_replacement(list_name, x)
from the itertools
module takes the list name and a number x
as the parameters and returns a list of tuples each of length x
containing all possible combinations of the list’s elements. A combination of one element in the list with itself is possible using this function.
For example:
from itertools import combinations_with_replacement
A = [1, 5, "Hi"]
temp = combinations_with_replacement(A, 2)
for i in list(temp):
print(i)
Output:
Use Recursion and Backtracking to Get All Combinations of a List in Python
Another approach that we can make use of to generate all combinations of the elements of a Python list is to use recursion and backtracking.
This can be done by simply iterating through each element and recursively generating combinations without considering the current element. This way, we can build the complete set of combinations.
Consider the following code.
def get_combinations(lst, r):
result = []
def backtrack(comb, start):
if len(comb) == r:
result.append(tuple(comb))
return
for i in range(start, len(lst)):
comb.append(lst[i])
backtrack(comb, i + 1)
comb.pop()
backtrack([], 0)
return result
my_list = [10, 5, "Hi"]
combinations = get_combinations(my_list, 2)
print(combinations)
Output:
Use a Recursive Generator Function to Get All Combinations of a List in Python
You can also implement a recursive generator function to obtain all possible combinations of the elements of a list in Python. This approach tends to avoid generating the entire list of combinations upfront, which saves a lot of memory, making this the more optimum approach.
Consider the following code.
def generate_combinations(lst, r):
if r == 0:
yield []
return
for i in range(len(lst)):
element = lst[i]
remaining = lst[i + 1 :]
for comb in generate_combinations(remaining, r - 1):
yield [element] + comb
my_list = [10, 5, "Hi"]
combinations = list(generate_combinations(my_list, 2))
print(combinations)
Output:
Create a User-Defined powerset()
Function to Get All Combinations of a List in Python
In mathematics, a powerset of any set is a set that contains all the possible subsets of a given set along with an empty set. Power set of set S = {2, 5, 10}
is {{}, {2}, {5}, {10}, {2, 5}, {2, 10}, {5, 10}, {2, 5, 10}}
.
The following function, powerset()
, is used to loop through all the lengths r
of the list and print all the possible combinations of the list’s elements.
For example:
from itertools import chain, combinations
def powerset(list_name):
s = list(list_name)
return chain.from_iterable(combinations(s, r) for r in range(len(s) + 1))
A = [60, 7, "Hi"]
for x in powerset(A):
print(x)
Output:
Conclusion
Obtaining all list combinations in Python is a common task that can be accomplished using various methods. The itertools.combinations()
function from the itertools
module provides a convenient way to generate combinations efficiently.
However, if you prefer to implement your solution, recursion, backtracking, and recursive generator functions offer flexible and customizable approaches.
Consider the size of your list and the desired combination length when choosing a method, as the number of combinations can grow exponentially. Remember the memory usage and runtime performance, especially for large lists or long combinations.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python