Python 中列表的组合
Samyak Jain
2023年1月30日
Python
Python List
-
在 Python 中使用
itertools.combinations()
函数查找列表的组合 -
在 Python 中使用
itertools.combinations_with_replacement()
函数查找列表的组合 -
在 Python 中创建用户定义的
powerset()
函数以查找列表的组合
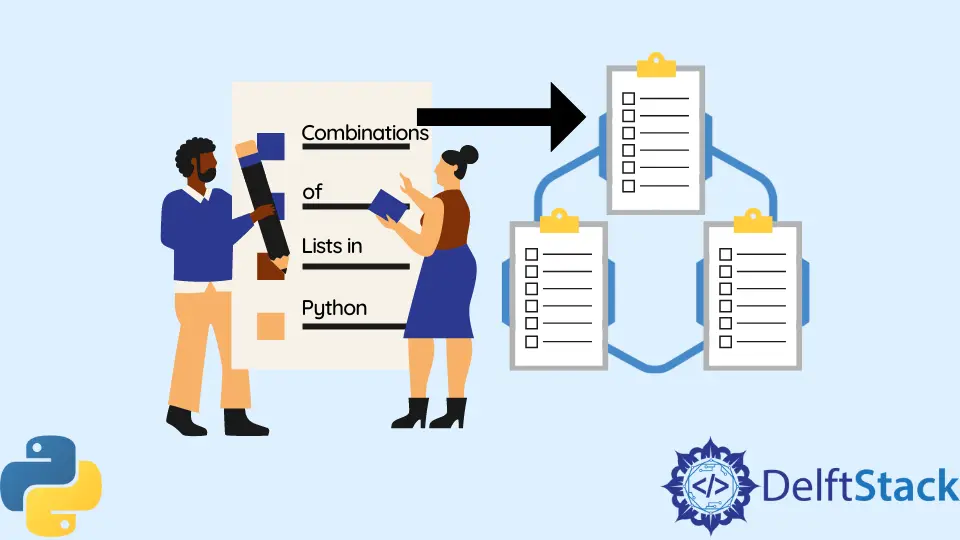
组合是一种确定元素集合中可能的排列数量的技术。在元素的组合中,元素以任意顺序被选择。
在本教程中,我们将在 Python 中找到列表元素的总组合。
在 Python 中使用 itertools.combinations()
函数查找列表的组合
来自 itertools
模块的函数 combinations(list_name, x)
将列表名称和数字 ‘x’ 作为参数,并返回一个元组列表,每个元组的长度为 ‘x’,其中包含一个元素的所有可能组合。包含其他元素的列表。
例如,
from itertools import combinations
A = [10, 5, "Hi"]
temp = combinations(A, 2)
for i in list(temp):
print(i)
输出:
(10, 5)
(10, 'Hi')
(5, 'Hi')
排序列表将按排序顺序输出组合元组。使用 combinations()
函数无法将列表中的一个元素与其自身组合。
在 Python 中使用 itertools.combinations_with_replacement()
函数查找列表的组合
来自 itertools
模块的函数 combinations_with_replacement(list_name, x)
将列表名称和数字 x
作为参数,并返回一个元组列表,每个元组的长度为 x
,其中包含列表元素的所有可能组合。使用此功能可以将列表中的一个元素与其自身组合。
例如,
from itertools import combinations_with_replacement
A = [1, 5, "Hi"]
temp = combinations_with_replacement(A, 2)
for i in list(temp):
print(i)
输出:
(1, 1)
(1, 5)
(1, 'Hi')
(5, 5)
(5, 'Hi')
('Hi', 'Hi')
在 Python 中创建用户定义的 powerset()
函数以查找列表的组合
在数学中,任何集合的幂集是一个包含给定集合的所有可能子集以及一个空集的集合。集合 S = {2, 5, 10}
的幂集是 {{}, {2}, {5}, {10}, {2, 5}, {2, 10}, {5, 10}, {2, 5, 10}}
。下面的函数 powerset()
用于遍历列表的所有长度 ‘r’ 并打印列表元素的所有可能组合。
例如,
from itertools import chain, combinations
def powerset(list_name):
s = list(list_name)
return chain.from_iterable(combinations(s, r) for r in range(len(s) + 1))
A = [60, 7, "Hi"]
for x in powerset(A):
print(x)
输出:
()
(1,)
(5,)
('Hi',)
(1, 5)
(1, 'Hi')
(5, 'Hi')
(1, 5, 'Hi')
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe