How to Check if Index Exists in Python List
- Check if Index Exists in Python List Using the List Range
-
Check if Index Exists in Python List Using
try-except
Block - Check if Index Exists in Python List Using Custom Function
- Conclusion
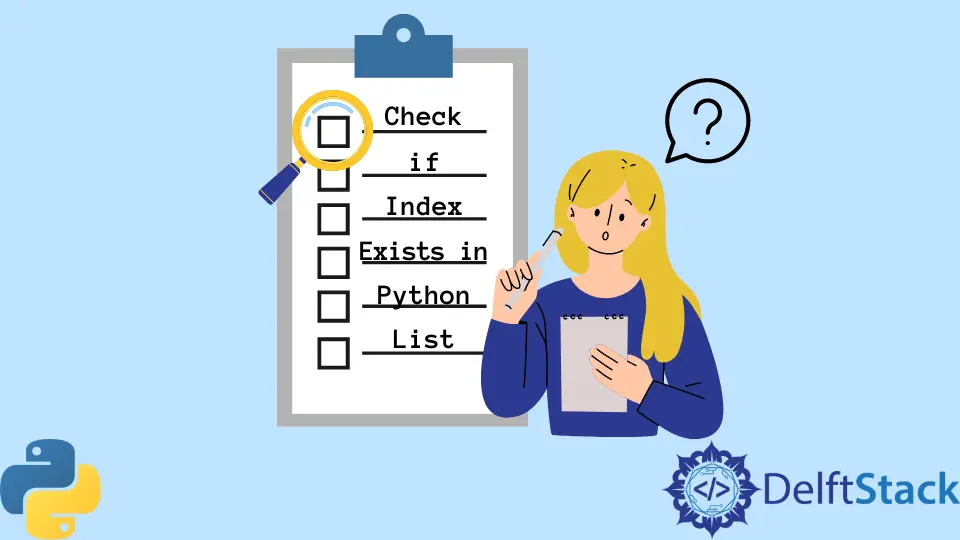
Checking if an index exists in a Python list is a common task to ensure that the index is within the acceptable range before trying to modify or access an element. Python lists are zero-indexed, meaning the first element is at index 0
, the second at index 1
, and so on.
Failing to validate an index could lead to an IndexError
. In this article, we’ll introduce various ways to verify if an index exists in a list in Python.
Check if Index Exists in Python List Using the List Range
We will have to check if the index exists in the range of 0
and the length of the list. In the following example, we will use the built-in range
function to define a valid range of indices for the list.
This technique ensures that the index being checked is within the permissible bounds of the list to prevent IndexError
.
Code:
fruit_list = ["Apple", "Banana", "Pineapple"]
for index in range(0, 5):
if 0 <= index < len(fruit_list):
print("Index ", index, " in range")
else:
print("Index ", index, " not in range")
Output:
Index 0 in range
Index 1 in range
Index 2 in range
Index 3 not in range
Index 4 not in range
In the code, we initialize a list called fruit_list
containing the elements ["Apple", "Banana", "Pineapple"]
. Subsequently, we iterate over a range of indices from 0
to 4
using the for
loop.
Inside the for
loop, we use an if
statement to verify if the current index is within the valid range of the list. If true, we print a message stating that the index is in range; otherwise, we print a message indicating that the index is not in range.
The output confirms that indices 0
, 1
, and 2
are in range, while indices 3
and 4
are not in the range of the list.
Check if Index Exists in Python List Using try-except
Block
Using a try-except
block is a robust approach to handle potential IndexError
exceptions when checking if an index exists in a list. This method involves attempting to access the element at the specified index within a try
block and catching any raised IndexError
within the associated except
block.
Code:
fruit_list = ["Apple", "Banana", "Pineapple"]
for index in range(0, 5):
try:
fruit_list[index]
print("Index ", index, " in range")
except IndexError:
print("Index ", index, " does not exist")
Output:
Index 0 in range
Index 1 in range
Index 2 in range
Index 3 does not exist
Index 4 does not exist
In this code, we utilize a try-except
block to handle potential IndexError
exceptions while accessing elements in the list fruit_list
. Within a for
loop iterating over indices from 0
to 4
, we attempt to access the element at the current index within the try
block.
If the index is within the valid range, we print a message indicating that the index is in range. Conversely, if an IndexError
occurs, we catch it in the except
block and print a message stating that the index does not exist.
The output confirms that indices 0
, 1
, and 2
are in range, while indices 3
and 4
do not exist in the list.
Check if Index Exists in Python List Using Custom Function
We will use a custom function to check if an index exists in a list by creating a specialized function that performs the validation. This custom function, often named index_exists
or something similar, takes a list and an index as input parameters and provides a Boolean value that signifies whether the index is within the valid range of the list.
Code:
def index_exists(my_list, index_to_check):
return 0 <= index_to_check < len(my_list)
fruit_list = ["Apple", "Banana", "Pineapple"]
index_to_check = 2
if index_exists(fruit_list, index_to_check):
print(f"Index {index_to_check} exists in the list.")
else:
print(f"Index {index_to_check} does not exist in the list.")
Output:
Index 2 exists in the list.
In this code, we define a custom function called index_exists
that checks if a given index is within the valid range of a specified list. The function takes two parameters: the list to be checked (my_list
) and the index to be validated (index_to_check
), and it returns a Boolean value based on whether the index is within the valid range.
In the main part of the code, we create a list called fruit_list
containing elements ["Apple", "Banana", "Pineapple"]
. We then set the variable index_to_check
to 2
.
Next, using an if-else
statement, we call the index_exists
function with the list and index as arguments. If the index exists in the list, we print a message stating that the index exists; otherwise, we’ll print a message indicating that the index does not exist.
The output confirms that the index 2
exists in the provided list of fruits.
Conclusion
The article explores three methods to check if an index exists in a Python list: using the list range, a try-except
block, and a custom function. The list range method leverages the range
function to validate indices, preventing potential IndexError
.
The try-except
block offers robust error handling by attempting to access elements and catching any raised IndexError
. The custom function, index_exists
, encapsulates the validation logic for modularity and clarity. Each method provides a distinct approach, emphasizing code reliability and stability when working with lists.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python