Array or List of Dictionaries in Python
- Create an Array or List of Dictionaries in Python
- Access Dictionary Elements From an Array or List of Dictionaries in Python
- Append Dictionaries to the Array or List of Dictionaries in Python
- Use List Comprehension to Create an Array or List of Dictionaries in Python
- Use a Loop to Create an Array or List of Dictionaries in Python
-
Use the
dict()
Constructor to Create an Array or List of Dictionaries in Python - Conclusion
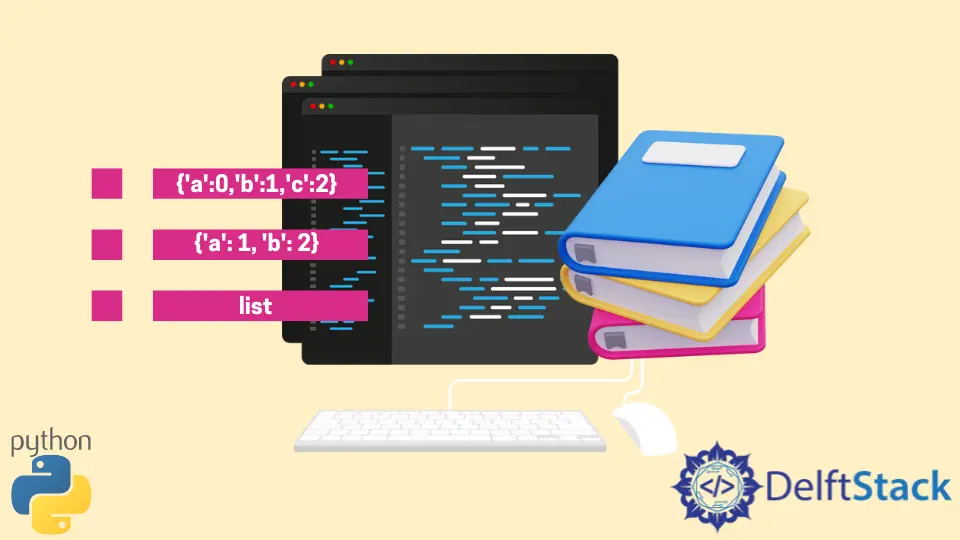
Dictionaries in Python are versatile data structures that allow you to store key-value pairs. Sometimes, you may need to work with a list of dictionaries to manage and manipulate data efficiently.
In this article, we’ll cover various aspects of creating and working with lists of dictionaries in Python. We’ll explore methods to create them, access dictionary elements within the list, create lists with multiple dictionaries, and perform operations like appending and updating dictionaries in a list.
Create an Array or List of Dictionaries in Python
To create an array of dictionaries in Python, you need to define a list where each element is a dictionary. These dictionaries can contain different keys and values.
Let’s take a look at an example:
lst = [{"a": 0, "b": 1, "c": 2}, {"d": 3, "c": 4, "b": 5}, {"a": 5, "d": 6, "e": 1}]
In the example above, we have created a list where each element is a dictionary. It’s important to note that each dictionary within the list is a separate entity; there is no relationship between them, so they can have similar keys or values.
Access Dictionary Elements From an Array or List of Dictionaries in Python
Accessing dictionary elements from a list of multiple dictionaries is a common operation when working with structured data. Let’s dive deeper into this topic to understand different scenarios and techniques for accessing elements effectively.
Assuming we have a list of dictionaries like this:
list = [{"a": 0, "b": 1, "c": 2}, {"d": 3, "c": 4, "b": 5}, {"a": 5, "d": 6, "e": 1}]
Access a Single Element by Index
To access a single dictionary element in the list, you can use indexing. For example:
# Access the second dictionary in the list
second_element = list[1]
print(second_element)
Output:
{'d': 3, 'c': 4, 'b': 5}
This code retrieves the dictionary at index 1 (the second dictionary) in the list
.
Access a Specific Value Within a Dictionary
To access a specific value within a dictionary in the list, you can use indexing to select the dictionary and then specify the key:
# Access the 'c' key of the second dictionary
c_value = list[1]["c"]
print(c_value)
Output:
4
Here, we first access the second dictionary (list[1]
) and then access the 'c'
key within that dictionary.
Append Dictionaries to the Array or List of Dictionaries in Python
You can also add dictionaries to the list using the append()
function. Here’s an example:
lst = [{"a": 0, "b": 1, "c": 2}, {"d": 3, "c": 4, "b": 5}, {"a": 5, "d": 6, "e": 1}]
lst.append({"f": 4, "g": 5, "c": 2}) # Appending a new dictionary to the list
print(lst)
Output:
[{'a': 0, 'b': 1, 'c': 2}, {'d': 3, 'c': 4, 'b': 5}, {'a': 5, 'd': 6, 'e': 1}, {'f': 4, 'g': 5, 'c': 2}]
This demonstrates how you can dynamically add dictionaries to your list of dictionaries.
Depending on your specific use case and coding style preferences, you can create an array or list of dictionaries using various methods. Below are some common ways to create an array of dictionaries.
Use List Comprehension to Create an Array or List of Dictionaries in Python
Creating an array or list of dictionaries can sometimes be a repetitive process. Python’s list comprehension can simplify this task by allowing you to create a list of empty dictionaries or repeat the same dictionary as an element of the list for a specified number of times.
Let’s take a look at an example:
lst1 = [dict() for i in range(4)] # Create a list of 4 empty dictionaries
lst2 = [
{"a": 1, "b": 2} for i in range(4)
] # Create a list with the same dictionary repeated 4 times
print(lst1)
print(lst2)
Output:
[{}, {}, {}, {}]
[{'a': 1, 'b': 2}, {'a': 1, 'b': 2}, {'a': 1, 'b': 2}, {'a': 1, 'b': 2}]
Here, we use list comprehension to create a list of empty dictionaries and a list where the same dictionary is repeated multiple times as list elements. You can then populate these dictionaries with values as needed.
Use a Loop to Create an Array or List of Dictionaries in Python
You can also create an array of dictionaries by using a loop. This approach is useful when you want more control over the dictionary creation process or need to create dictionaries dynamically.
Here’s the complete working code:
# Initialize an empty list to store dictionaries
array_of_dicts = []
# Define the number of dictionaries you want in the array
num_dicts = 3
# Create dictionaries using a loop and append them to the list
for i in range(num_dicts):
dictionary = {"key": i, "value": i * 2}
array_of_dicts.append(dictionary)
# Print the resulting array of dictionaries
for dictionary in array_of_dicts:
print(dictionary)
Output:
{'key': 0, 'value': 0}
{'key': 1, 'value': 2}
{'key': 2, 'value': 4}
We used a for
loop to create the dictionaries. The loop runs num_dicts
(3) times, creating one dictionary in each iteration.
Inside the loop, we also defined the structure of each dictionary.
In this code snippet, i
is used as the key and i * 2
as the value. You can customize these key-value pairs to match your specific data requirements.
After creating each dictionary, we appended it to the array_of_dicts
list using the append()
method.
Use the dict()
Constructor to Create an Array or List of Dictionaries in Python
Another way to create an array of dictionaries in Python is by using the dict()
constructor, which offers a straightforward and flexible way to define dictionaries.
You can use the dict()
constructor to create an empty dictionary or to create dictionaries with initial key-value pairs.
Here are some basic examples:
-
Create an empty dictionary:
empty_dict = dict()
This creates an empty dictionary named
empty_dict
.
-
Create a dictionary with initial key-value pairs:
person = dict(name="John", age=30, city="New York")
Here, we create a dictionary called
person
with three key-value pairs:'name': 'John'
,'age': 30
, and'city': 'New York'
.
Here’s a working code to create an array of dictionaries using the dict()
constructor:
# Create an array of dictionaries using the dict() constructor
array_of_dicts = [
dict(key1="value1", key2="value2"),
dict(key3="value3", key4="value4"),
dict(key5="value5", key6="value6"),
]
# Print the resulting array of dictionaries
for dictionary in array_of_dicts:
print(dictionary)
Output:
{'key1': 'value1', 'key2': 'value2'}
{'key3': 'value3', 'key4': 'value4'}
{'key5': 'value5', 'key6': 'value6'}
When using the dict()
constructor, you can add as many key-value pairs as needed, separated by commas.
Keep in mind that dictionary keys must be hashable and unique. This means you cannot use mutable objects like lists as keys because they are not hashable.
Additionally, if you provide duplicate keys when using the dict()
constructor, the last value for a key will overwrite any previous values.
For example,
# This will result in a dictionary with one key-value pair, 'key': 3
dictionary = dict(key=1, key=2, key=3)
Whether you need to create dictionaries from scratch, modify existing ones, or convert data from other structures into dictionaries, the dict()
constructor is a valuable resource for Python developers.
Conclusion
Working with lists of dictionaries in Python provides a flexible way to manage and manipulate structured data. Whether you’re handling data records or configuration settings, lists of dictionaries allow you to organize and work with data efficiently.
Understanding how to create, access, and append dictionaries in such lists is a fundamental skill for many Python programming tasks.
Related Article - Python Dictionary
- How to Check if a Key Exists in a Dictionary in Python
- How to Convert a Dictionary to a List in Python
- How to Get All the Files of a Directory
- How to Find Maximum Value in Python Dictionary
- How to Sort a Python Dictionary by Value
- How to Merge Two Dictionaries in Python 2 and 3
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python