Action Chains in Selenium Python
- How to Create a Simple Action Chain Object in Selenium Python
- Implement an Action Chain in Selenium Python
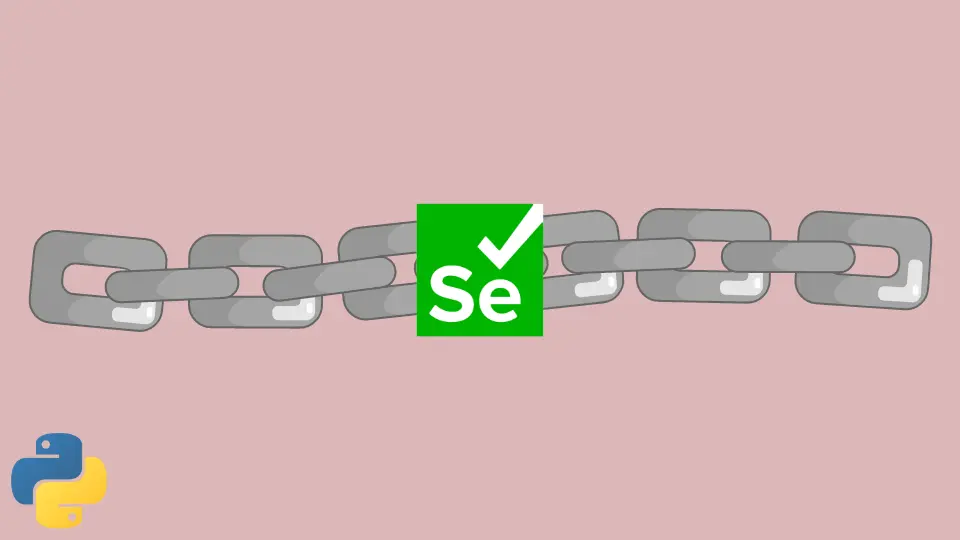
Selenium is a standalone web-based tool utilized for automation. It is an open-source tool that anyone can utilize.
When combined with the Python language, this tool is utilized for testing. Action chains are a fundamental part of Selenium and offer a way to manage low-level interactions like keypress, mouse movements, mouse button actions, and interactions with the context menu.
This tutorial demonstrates how to implement action chains in Selenium with Python.
We usually use action chains when there is a need to automate advanced scripts in which we need to either click or drag on any of the elements.
Action chain objects are utilized to implement Action Chains in Python. An action chain object can store all the actions in a queue, which performs all the stored operations by calling the perform()
function.
How to Create a Simple Action Chain Object in Selenium Python
A simple Action Chain
object can be created by importing the necessary modules and passing some values in Python code.
The following code creates a simple Action Chain
object.
from selenium import webdriver # webdriver is imported from selenium
from selenium.webdriver.common.action_chains import (
ActionChains,
) # ActionChains is imported from webdriver
driver = webdriver.Firefox() # a webdriver object is then created
action = ActionChains(
driver
) # An action chain object is finally created with the driver
- The
Action Chain
class is firstly imported from theselenium.webdriver
module. - A
driver
is then defined. - This
driver
is then passed as the key argument to theaction chain
object. - The
action chain
object is created and ready to perform any feasible operation.
Apart from the generic utilization, action chains can also be used in chain or queue patterns.
Implement an Action Chain in Selenium Python
To explain this better, let us take an example of the website https://www.delftstack.com/
and do some experiments in the Python code.
The example code taken below runs the website https://www.delftstack.com/
first and then clicks on the TUTORIALS
button in the header, due to which the browser then redirects us to the https://www.delftstack.com/tutorial/
link of the website on its own.
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
driver = webdriver.Firefox()
driver.get("https://www.delftstack.com/")
clicker1 = driver.find_element_by_link_text("TUTORIALS")
action = ActionChains(driver)
action.click(on_element=clicker1)
action.perform()
The above code provides the following output:
The code first runs and opens the website’s home page https://www.delftstack.com/
.
Then, we are automatically redirected to the https://www.delftstack.com/tutorials/
webpage as per the manipulations in the above code.
- The
Action Chain
class is firstly imported from theselenium.webdriver
module. - A
driver
is then defined, which is the websitehttps://www.delftstack.com/
. We use theget()
function for this. - Then, we define an element
clicker1
, theTUTORIALS
button in the website’s header. - This
driver
is passed as the key argument to theaction chain
object. - Then, we utilize the
action.click()
function and pass the previously definedclicker1
element as its argument. - We then execute the
perform()
function so that the manipulations defined in the code can take place.
In this part of the article, we took a real-life example of using action chains in Selenium with Python.
However, just implementing an action chain in Selenium is not enough; we also need to know the Action Chain methods performed after creating an Action Chain
object. We have described some of the important ones for you below.
click
- the method for clicking an element.click_and_hold
- the method for holding the left mouse button down on the given element.double_click
- the method for double-clicking an element.drag_and_drop
- holds the LMB on an element, drags it to the target site, and releases it afterward.move_to_element
- The mouse is moved to the element’s center.perform
- all the actions stored in theaction chain
object are performed using this method.pause
- all the inputs are paused for a given duration. The time duration is taken as a unit of seconds.release
- if a mouse button is held, it releases it.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python