How to Change Tkinter Button Color
-
Set Tkinter
Button
Color -
Change Tkinter
Button
Color Withconfigure
Method -
Change Tkinter
Button
Color Withbg
/fg
Attributes
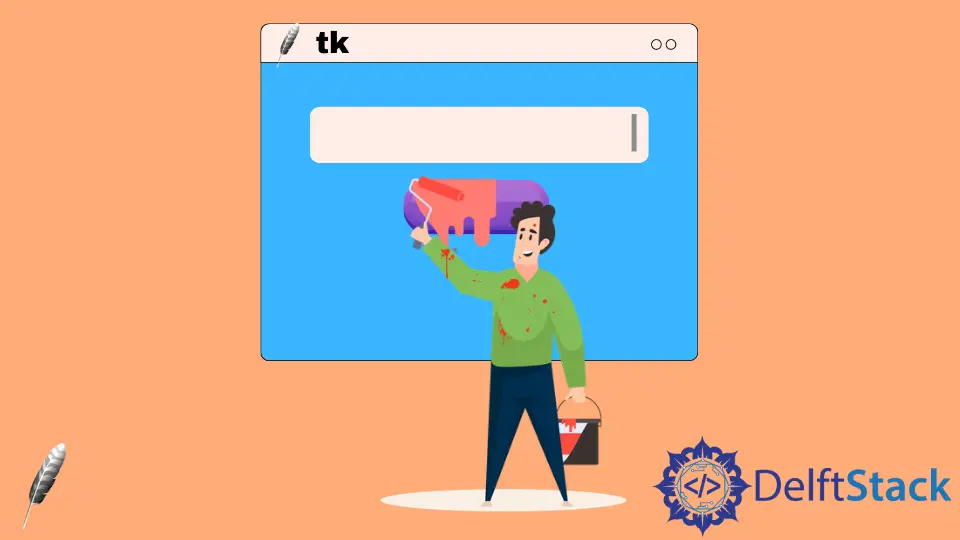
Tkinter Button
widget has attributes bg
and fg
to set the background and foreground colors. We could assign colors to bg
and fg
when we initialize the Button
object, and change Tkinter Button
color with configure
method or assign new values to bg
and fg
keys.
Set Tkinter Button
Color
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.root.geometry("250x100")
self.buttonA = tk.Button(self.root, text="Color", bg="blue", fg="red")
self.buttonB = tk.Button(
self.root, text="Click to change color", bg="gray", fg="purple"
)
self.buttonA.pack(side=tk.LEFT)
self.buttonB.pack(side=tk.RIGHT)
self.root.mainloop()
app = Test()
You could also replace bg
with background
, fg
with foreground
to set the Tkinter Button
background and foreground color.
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.root.geometry("250x100")
self.buttonA = tk.Button(
self.root, text="Color", background="blue", foreground="red"
)
self.buttonB = tk.Button(
self.root,
text="Click to change color",
background="gray",
foreground="purple",
)
self.buttonA.pack(side=tk.LEFT)
self.buttonB.pack(side=tk.RIGHT)
self.root.mainloop()
app = Test()
Change Tkinter Button
Color With configure
Method
After the Tkinter Button
widget is created, we could change its color by using the configure
method.
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.root.geometry("250x100")
self.buttonA = tk.Button(self.root, text="Color", bg="blue", fg="red")
self.buttonB = tk.Button(
self.root, text="Click to change color", command=self.changeColor
)
self.buttonA.pack(side=tk.LEFT)
self.buttonB.pack(side=tk.RIGHT)
self.root.mainloop()
def changeColor(self):
self.buttonA.configure(bg="yellow")
app = Test()
self.buttonA.configure(bg="yellow")
It configures the background
or equally bg
to be yellow
.
Change Tkinter Button
Color With bg
/fg
Attributes
bg
and fg
are keys
of Tkinter Button
widget object dictionary, therefore, we could change the Tkinter Button
color by assigning new values of these keys
.
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.root.geometry("250x100")
self.buttonA = tk.Button(self.root, text="Color", bg="blue", fg="red")
self.buttonB = tk.Button(
self.root, text="Click to change color", command=self.changeColor
)
self.buttonA.pack(side=tk.LEFT)
self.buttonB.pack(side=tk.RIGHT)
self.root.mainloop()
def changeColor(self):
self.buttonA["bg"] = "gray"
self.buttonA["fg"] = "cyan"
app = Test()
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook