How to Bind Multiple Commands to Tkinter Button
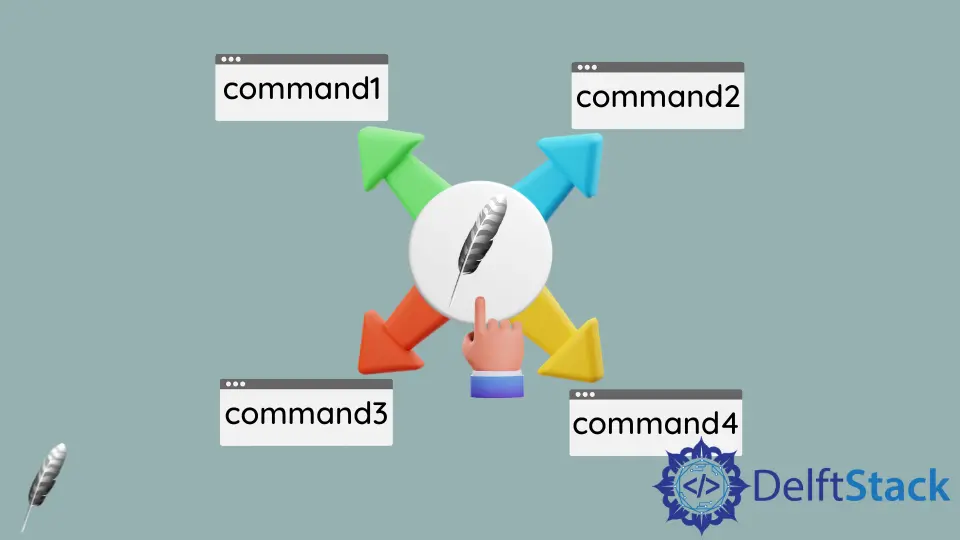
In this tutorial, we will demonstrate how to bind multiple commands to a Tkinter button. Multiple commands could be executed after the button is clicked.
Bind Multiple Commands to Tkinter Button
The Tkinter button has only one command
property so that multiple commands or functions should be wrapped to one function that is bound to this command
.
We could use lambda
to combine multiple commands as,
def command():
return [funcA(), funcB(), funcC()]
This lambda
function will execute funcA
, funcB
, and funcC
one by one.
labmda
Bind Multiple Commands to Tkinter Button Example
try:
import Tkinter as tk
except:
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.root.geometry("200x100")
self.button = tk.Button(
self.root,
text="Click Me",
command=lambda: [self.funcA(), self.funcB(), self.funcC()],
)
self.button.pack()
self.labelA = tk.Label(self.root, text="A")
self.labelB = tk.Label(self.root, text="B")
self.labelC = tk.Label(self.root, text="C")
self.labelA.pack()
self.labelB.pack()
self.labelC.pack()
self.root.mainloop()
def funcA(self):
self.labelA["text"] = "A responds"
def funcB(self):
self.labelB["text"] = "B responds"
def funcC(self):
self.labelC["text"] = "C responds"
app = Test()
Combine Functions to One Single Function in Tkinter
def combineFunc(self, *funcs):
def combinedFunc(*args, **kwargs):
for f in funcs:
f(*args, **kwargs)
return combinedFunc
The above function defines a function inside a function and then returns the function object.
for f in funcs:
f(*args, **kwargs)
It executes all the functions given in the parenthesis of combineFunc
.
try:
import Tkinter as tk
except:
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.root.geometry("200x100")
self.button = tk.Button(
self.root,
text="Click Me",
command=self.combineFunc(self.funcA, self.funcB, self.funcC),
)
self.button.pack()
self.labelA = tk.Label(self.root, text="A")
self.labelB = tk.Label(self.root, text="B")
self.labelC = tk.Label(self.root, text="C")
self.labelA.pack()
self.labelB.pack()
self.labelC.pack()
self.root.mainloop()
def combineFunc(self, *funcs):
def combinedFunc(*args, **kwargs):
for f in funcs:
f(*args, **kwargs)
return combinedFunc
def funcA(self):
self.labelA["text"] = "A responds"
def funcB(self):
self.labelB["text"] = "B responds"
def funcC(self):
self.labelC["text"] = "C responds"
app = Test()
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook