How to Pass Arguments to Tkinter Button Command
-
Pass Arguments to
command
in Tkinter Button Withpartials
-
Pass Arguments to
command
in Tkinter Button Withlambda
Function
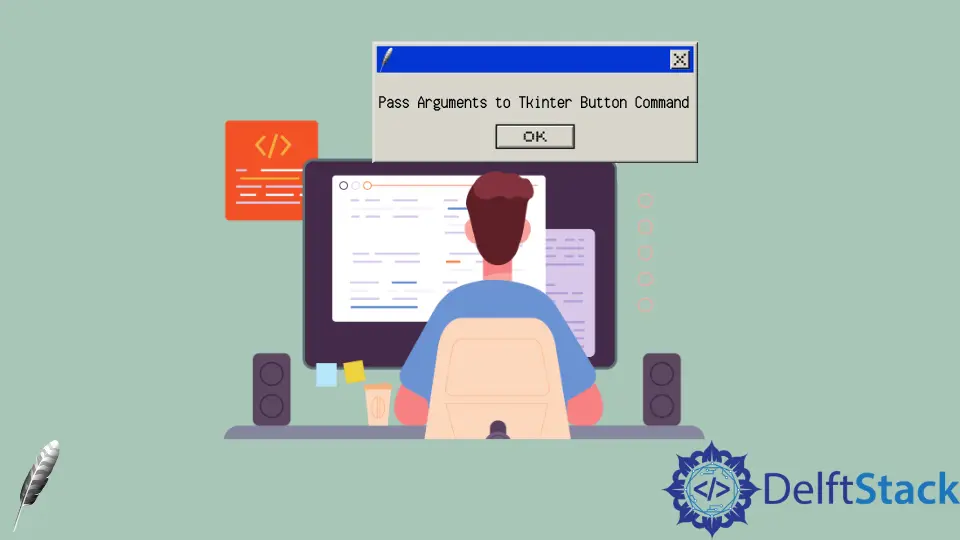
command
option in Tkinter Button
widget is triggered when the user presses the button. In some scenarios, you need to pass arguments to the attached command function, but you couldn’t simply pass the arguments like below,
button = tk.Button(app, text="Press Me", command=action(args))
We will introduce two ways to pass the arguments to the command function.
Pass Arguments to command
in Tkinter Button With partials
As demonstrated in Python Tkinter tutorial, you have the option to use the partial
object from the functools
module.
from sys import version_info
if version_info.major == 2:
import Tkinter as tk
elif version_info.major == 3:
import tkinter as tk
from functools import partial
app = tk.Tk()
labelExample = tk.Button(app, text="0")
def change_label_number(num):
counter = int(str(labelExample["text"]))
counter += num
labelExample.config(text=str(counter))
buttonExample = tk.Button(
app, text="Increase", width=30, command=partial(change_label_number, 2)
)
buttonExample.pack()
labelExample.pack()
app.mainloop()
buttonExample = tk.Button(
app, text="Increase", width=30, command=partial(change_label_number, 2)
)
partial(change_label_numer, 2)
returns a callable object that behaves like a func
when it is called.
Pass Arguments to command
in Tkinter Button With lambda
Function
You could also use Python lambda
operator or function to create a temporary, one-time simple function to be called when the Button
is clicked.
from sys import version_info
if version_info.major == 2:
import Tkinter as tk
elif version_info.major == 3:
import tkinter as tk
app = tk.Tk()
labelExample = tk.Button(app, text="0")
def change_label_number(num):
counter = int(str(labelExample["text"]))
counter += num
labelExample.config(text=str(counter))
buttonExample = tk.Button(
app, text="Increase", width=30, command=lambda: change_label_number(2)
)
buttonExample.pack()
labelExample.pack()
app.mainloop()
The syntax of lambda
function is
lambda: argument_list: expression
You don’t need any arguments in this example, therefore, you could leave the argument list empty and only give the expression.
buttonExample = tk.Button(
app, text="Increase", width=30, command=lambda: change_label_number(2)
)
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook