How to Update the Tkinter Button Text
-
Changing
Tkinter
Button Text Using Functions -
Changing
Tkinter
Button Text Using Lambda Functions -
Changing
Tkinter
Button Text Using Class and Instance Methods -
Changing
Tkinter
Button Text UsingStringVar
- Conclusion
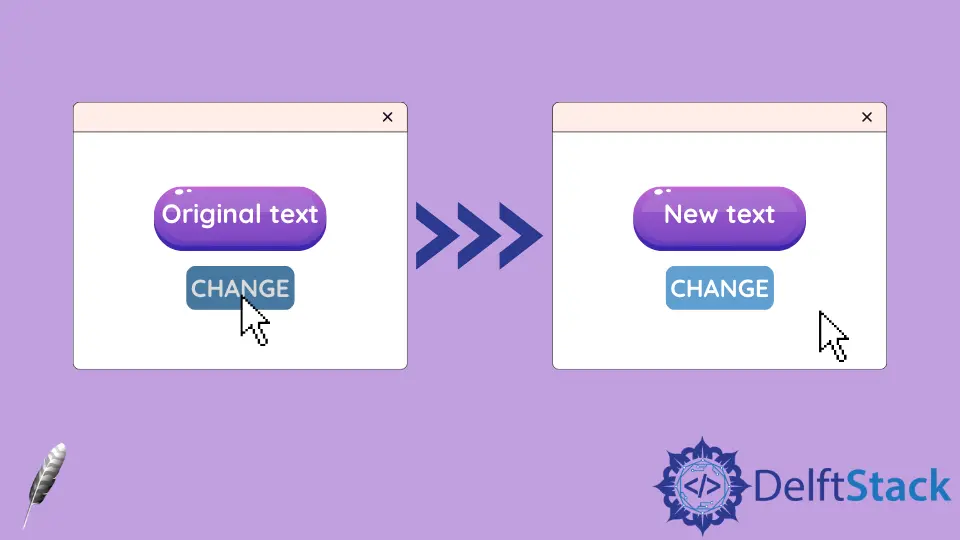
In this tutorial, we will introduce how to change the Tkinter button text. It is similar to the methods to change the Tkinter label text.
Graphical User Interfaces (GUIs) play a crucial role in enhancing user interaction with software applications. Tkinter
, a standard GUI toolkit for Python, provides a range of widgets, including buttons that allow users to trigger actions.
In many cases, dynamically updating the button text after a click event can provide a more interactive and responsive user experience. In this article, we’ll explore methods for achieving this in Tkinter
.
Changing Tkinter
Button Text Using Functions
When working with Tkinter
buttons, the ability to change the text dynamically after a click is a powerful feature, this can be particularly useful in scenarios where the button serves multiple purposes or provides feedback based on user actions.
By associating a function with the button’s click event, we can seamlessly update the button text, offering a more dynamic and intuitive user interface.
Using functions to change button text after clicking is a best practice in software development. It promotes code organization, readability, reusability, and maintainability.
This approach aligns with fundamental principles of good software design and contributes to the creation of robust and scalable GUI applications.
Let’s delve into a complete code example demonstrating how to change the Tkinter
button text after a click using the functions method:
import tkinter as tk
def change_text():
button.config(text="New Text")
root = tk.Tk()
button = tk.Button(root, text="Click me", command=change_text)
button.pack()
root.mainloop()
The script starts by importing the Tkinter
module and renaming it as tk
for brevity. Tkinter
provides essential tools for building graphical user interfaces in Python.
Next, a function named change_text
is defined to handle the logic for changing the button text. Within this function, the config
method is employed to set the new text for the button.
Following that, the main Tkinter
window is created using tk.Tk()
and assigned to the variable root
, serving as the container for the GUI elements.
The tk.Button
constructor is then utilized to create a button called button
with the initial text Click me
. The command
parameter is set to the change_text
function, indicating that this function should execute when the button is clicked.
In order to organize the button within the Tkinter
window, the pack
method is employed, ensuring proper display and sizing.
Finally, the root.mainloop()
call initiates the Tkinter
event loop, enabling the GUI to respond to user interactions.
Upon running this script, a GUI window will appear with a single button labeled Click me
.
When the button is clicked, the change_text
function will be executed, updating the button text to New Text
.
This immediate visual feedback demonstrates the dynamic nature of the Tkinter
button text modification.
Changing Tkinter
Button Text Using Lambda Functions
Using lambda functions in Tkinter
provides an elegant way to achieve dynamic behavior while keeping the code concise and readable.
Unlike traditional functions that require a separate definition, lambda functions are anonymous and can be defined directly within the button creation, making the code more compact and readable. This approach is preferred when the logic is short and doesn’t warrant a dedicated function.
Lambda functions are especially handy for on-the-fly actions, offering a quick and clear way to express the intended behavior, aligning with the principle of simplicity and readability in Python code.
Let’s dive into a complete code example demonstrating how to change the Tkinter
button text after a click using lambda functions:
import tkinter as tk
root = tk.Tk()
button = tk.Button(
root, text="Click me", command=lambda: button.config(text="You clicked me!")
)
button.pack()
root.mainloop()
The process begins by importing the tkinter
module as tk
to streamline code. This module equips us with the tools needed for creating graphical user interfaces in Python.
The main Tkinter
window is crafted using the tk.Tk()
constructor, assigned to the variable root
, acting as the container for GUI elements. Employing the tk.Button
constructor, we create a button named button
initially labeled Click me
.
Here, a lambda function is introduced within the command
parameter. This lambda succinctly encapsulates the logic for changing the button text, executed on a click.
The lambda function (lambda: button.config(text="You clicked me!")
) is a concise way to define a small, one-time-use function. The lambda function employs the config
method, updating the button’s text property to New Text
.
Using the pack
method organizes the button within the Tkinter
window for proper display and sizing.
Finally, the root.mainloop()
call launches the Tkinter
event loop, enabling the GUI to respond to user interactions.
Upon execution, the GUI displays a button labeled Click me
.
Upon clicking, the lambda function triggers, updating the button text to You clicked me!
, exemplifying the brevity and clarity of lambda functions in achieving dynamic behavior.
Changing Tkinter
Button Text Using Class and Instance Methods
Using classes
and instance methods
in changing button text after clicking offers a structured and organized approach to GUI development. This method promotes code encapsulation, ensuring that related functionality is contained within a class.
Instance methods
facilitate the modification of specific instances, enhancing modularity and readability. This approach aligns with object-oriented principles, enabling code reusability and maintaining a clear separation of concerns.
The use of classes and instance methods provides a scalable solution for larger GUI applications, promoting a more organized and maintainable codebase compared to other methods.
Let’s delve into a complete code example demonstrating how to change the Tkinter
button text after a click using a class and instance method:
import tkinter as tk
class ButtonApp:
def __init__(self, master):
self.master = master
self.button = tk.Button(master, text="Click me", command=self.change_text)
self.button.pack()
def change_text(self):
self.button.config(text="Button clicked")
root = tk.Tk()
app = ButtonApp(root)
root.mainloop()
In this script, we begin by importing the tkinter
module and aliasing it as tk
to streamline code.
The core of the application is a class called ButtonApp
, featuring two methods: __init__
and change_text
.
The __init__
method initializes the class when an instance is created, setting up variables and creating a button with the initial text Click me
. The change_text
method, an instance method, updates the button text to Button clicked!
using the config
method, ensuring specificity to the instance’s button.
The main Tkinter
window is then created, serving as the container for GUI elements. An instance of the ButtonApp
class is instantiated with the Tkinter
window as the master
.
Upon execution, the GUI displays a button labeled Click me
, and upon clicking, the change_text
method is triggered, updating the button text to Button clicked!
.
This approach demonstrates the benefits of encapsulation within a class, showcasing code modularity and maintainability.
Changing Tkinter
Button Text Using StringVar
The StringVar
method is commonly employed for changing button text after clicking due to its seamless integration with Tkinter
widgets. By linking a StringVar
to the button’s textvariable
property, developers establish a dynamic connection, allowing automatic updates to both the variable and the button text.
This approach is particularly useful for straightforward scenarios where a single property, like text, needs to be modified. The simplicity and directness of the StringVar
method make it an efficient choice, offering a clean and effective solution for achieving real-time updates in response to user interactions.
Let’s explore a complete code example demonstrating how to change the Tkinter
button text after a click using the StringVar
method:
import tkinter as tk
def change_text():
var.set("Clicked")
root = tk.Tk()
var = tk.StringVar()
var.set("Click me")
button = tk.Button(root, textvariable=var, command=change_text)
button.pack()
root.mainloop()
The script begins by importing the tkinter
module and aliasing it as tk
for brevity, providing essential tools for GUI creation.
A function named change_text
is defined to update a StringVar
(var
) to Clicked
when the button is clicked. The main Tkinter
window is then created as the container for GUI elements.
A StringVar
named var
is initialized with the text Click me
, linked to the button’s textvariable
property for dynamic control.
Using the tk.Button
constructor, a button named button
is created with this dynamic link. The change_text
function is set as the command to execute on button click.
The pack
method organizes the button, and the root.mainloop()
call initiates the Tkinter
event loop.
When executed, the GUI displays a button labeled Click me
, and on clicking, the change_text
function updates the StringVar
, dynamically reflecting the change in the button’s text to New Text
.
Conclusion
In conclusion, dynamically changing a Tkinter
button’s text after clicking is a common requirement in GUI development. Various methods offer diverse approaches, including using functions, lambda functions, class and instance methods, and the StringVar
method.
The choice depends on factors like code simplicity, modularity, and specific use cases. Each method contributes to creating responsive and user-friendly interfaces in Python, allowing developers to tailor their solutions based on the project’s requirements and coding preferences.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook