Mousebuttondown Event in PyGame
- Install PyGame in Python 2.7
- Install PyGame in Python 3.5
-
Detect the
MOUSEBUTTONDOWN
Event in PyGame
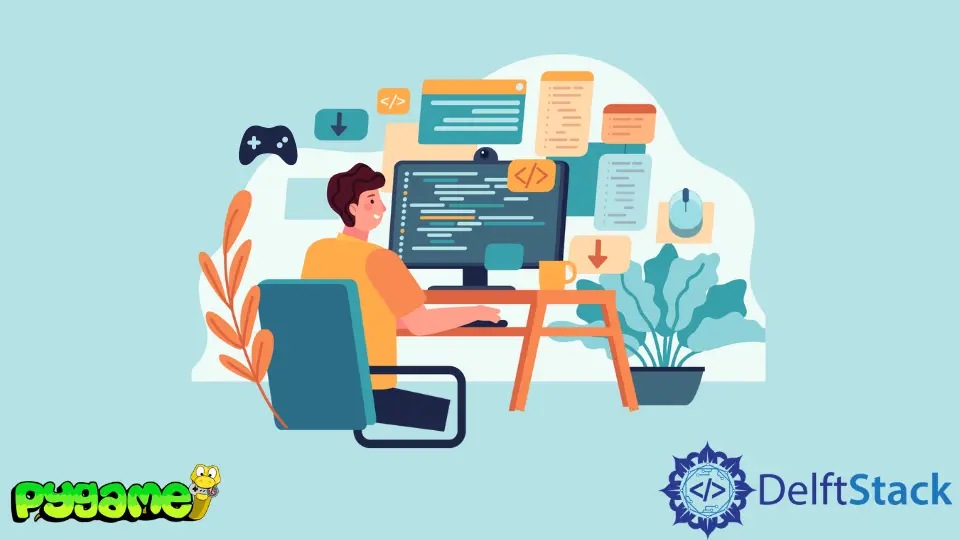
PyGame, as its name shows, is an open-source multimedia library in Python mainly used to make video games that include graphics, sounds, visuals, etc. These days, game programming is quite successful.
It is a cross-platform library that will work on multiple platforms with a single code-base. This library contains a lot of modules for graphics and sounds, as any game is meaningless without sounds or graphics.
This tutorial demonstrates to detect the MOUSEBUTTONDOWN
event using PyGame and trigger an action in response.
Install PyGame in Python 2.7
To use this library, we should install it first. If you are running Python 2.7
version, you should use the following command to install PyGame.
None
Install PyGame in Python 3.5
If you are running Python version 3.5, you should use the following command.
#Python 3.x
pip3 install pygame
Detect the MOUSEBUTTONDOWN
Event in PyGame
In any game, taking input from the player and performing an action is the main part of the game. The MOUSEBUTTONDOWN
event occurs when you click the mouse button, either the left or right, regardless of how much time you hold it after clicking.
In the following code, we set up the game window and defined the length and height of the window in pixels. We have created the main
loop (while
loop) and the event loop (for
loop) to capture the events.
In the for
loop, we have checked the event’s type using the if
conditions. When the MOUSEBUTTONDOWN
event is triggered, a message will display MOUSEBUTTONDOWN event occurred
.
If the user quits the game by pressing the X
button in the game’s window, the QUIT
event is triggered, and in response, the game is finished, and the window will exit. Here we have pressed the mouse button one time in the PyGame window.
Example Code:
# Python 3.x
import pygame
import sys
pygame.init()
display = pygame.display.set_mode((500, 500))
while True:
for event in pygame.event.get():
if event.type == pygame.MOUSEBUTTONDOWN:
print("MOUSEBUTTONDOWN event occured")
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
Output:
#Python 3.x
MOUSEBUTTONDOWN event occured
If you want to check which mouse button is pressed, left or right, you can check the values returned by the pygame.mouse.get_pressed()
method. Three values are returned by this method, one for each mouse button.
Here we have stored the returned values in a list. Each mouse button has associated a value, 1, 2, 3, 4, 5 for the left mouse button, middle mouse button, right mouse button, mouse wheel up, and mouse wheel down, respectively.
In the following code, we have checked which mouse button was clicked and printed a message accordingly. We have pressed the left and right mouse buttons.
Example Code:
# Python 3.x
import pygame
import sys
pygame.init()
display = pygame.display.set_mode((500, 500))
while True:
for event in pygame.event.get():
if event.type == pygame.MOUSEBUTTONDOWN:
mouse_presses = pygame.mouse.get_pressed()
if mouse_presses[0]:
print("Left mouse button pressed")
if mouse_presses[2]:
print("Right mouse button pressed")
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
Output:
#Python 3.x
Left mouse button pressed
Right mouse button pressed
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn