surface.blit() Function in Pygame
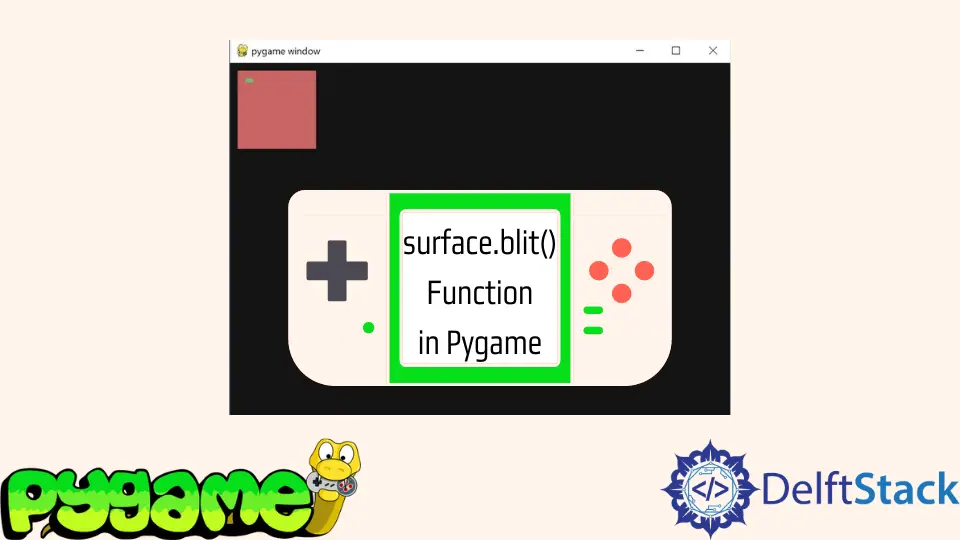
This tutorial teaches you what the blit()
function does in Pygame.
The code displayed here is not the complete code for a valid Pygame window. If you are interested in a bare-bones framework, consult this article.
the surface.blit()
Function in Pygame
Pygame revolves around surfaces, and the blit()
function overlaps these surfaces. The most prominent example of this would be our screen which is essentially just a surface, and we blit all the things that we see onto it.
But, we can also create other surfaces and overlap them with blit()
. Before we do this, we will explain the different arguments we can pass to this method.
Later, we will look at a concrete example, but we will focus on the function for now.
Below you see the structure of the blit()
method. Remember that this code won’t work because we didn’t set up the surfaces.
We call the blit()
function on one surface and start by providing the overlayed surface. Then we specify where the top left of surfaceTwo
will be on surfaceOne
.
We could end it here, and blit()
would work, but we can pass two more arguments. The area is a rect
representing the area of surfaceTwo
which will be blitted.
So, with just a portion of it and the special_flags
, we can define the blend mode of the blit.
Code snippet:
surfaceOne.blit(surfaceTwo, destination, area=none, special_flags=none)
Use the surface.blit()
Function in Pygame
Now, we will look at a concrete example. Let’s start by making some surfaces; keep in mind that this code is before the main loop.
We can make new surfaces with the Surface()
method, in which we have to provide the desired dimensions. We also fill both surfaces with a color to see them later.
In the last five lines of the code, you will see the blit()
function in action.
Code snippet:
surfaceOne = pygame.Surface((100, 100))
surfaceOne.fill(
(
200,
100,
100,
)
)
surfaceTwo = pygame.Surface((30, 30))
surfaceTwo.fill(
(
100,
200,
100,
)
)
surfaceOne.blit(surfaceTwo, [10, 10], [0, 0, 10, 5])
Now, we only need to blit surfaceOne
onto the screen, and we will see the result. This code happens in the main loop.
screen.blit(surfaceOne, [10, 10])
Output:
Complete Example Code
# Imports
import sys
import pygame
# Configuration
pygame.init()
fps = 60
fpsClock = pygame.time.Clock()
width, height = 640, 480
screen = pygame.display.set_mode((width, height), pygame.RESIZABLE)
surfaceOne = pygame.Surface((100, 100))
surfaceOne.fill(
(
200,
100,
100,
)
)
surfaceTwo = pygame.Surface((30, 30))
surfaceTwo.fill(
(
100,
200,
100,
)
)
surfaceOne.blit(surfaceTwo, [10, 10], [0, 0, 10, 5])
# Game loop.
while True:
screen.fill((20, 20, 20))
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.blit(surfaceOne, [10, 10])
pygame.display.flip()
fpsClock.tick(fps)
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub