KEYDOWN Function in Pygame
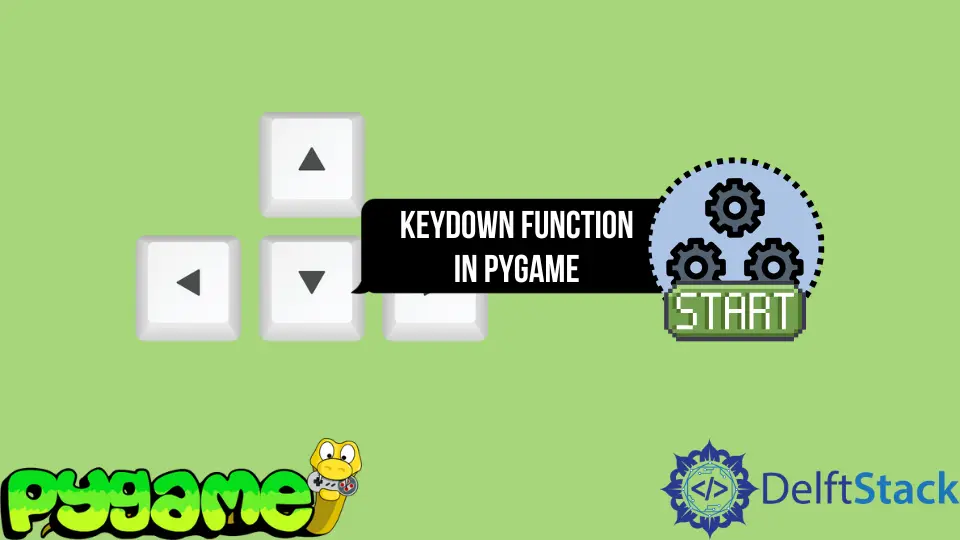
This short tutorial shows how to use KEYDOWN
in Pygame.
the KEYDOWN
Function in Pygame
Using the KEYDOWN
function, you capture the moment the key was pressed down, and it is a constant. We test it against the event types returned by the pygame.event.get()
method.
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
# your code
pass
There’ll be no output when the key is held down. We have two options to change that.
We can call the pygame.key.set_repeat(delay)
method and pass it the time in milliseconds, after which the event should be called again if held down. Then it will fire after pressing down after the amount of time we specified.
It is not best practice to do it this way, and it’s better to use pygame.key.get_pressed
and check for the key portrayed below.
keys = pygame.key.get_pressed()
if keys[pygame.K_w]:
print("W Pressed")
Complete Code:
# Imports
import sys
import pygame
# Configuration
pygame.init()
fps = 60
fpsClock = pygame.time.Clock()
width, height = 640, 480
screen = pygame.display.set_mode((width, height))
pygame.key.set_repeat(10)
# Game loop.
while True:
screen.fill((20, 20, 20))
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
# your code
pass
keys = pygame.key.get_pressed()
if keys[pygame.K_w]:
print("W Pressed")
pygame.display.flip()
fpsClock.tick(fps)
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub