Pandas Map Python
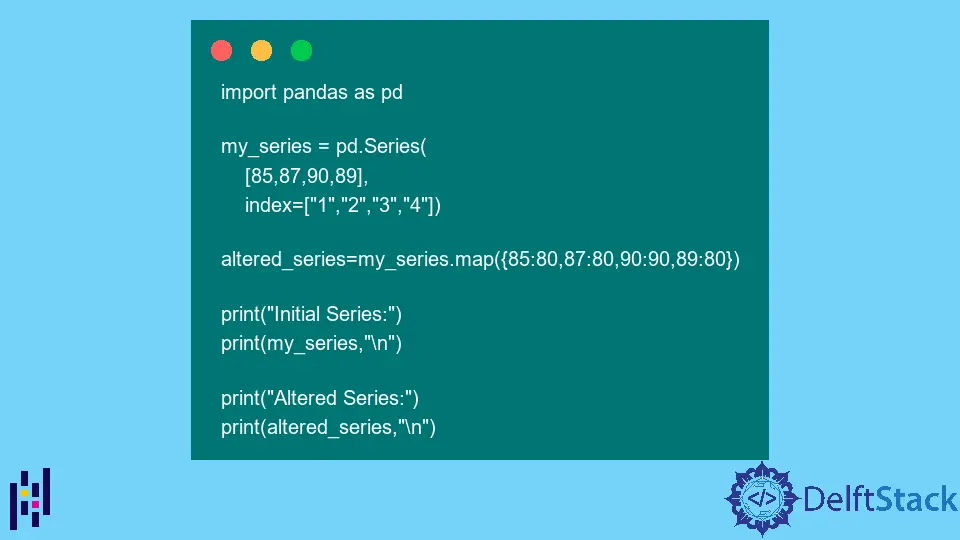
This tutorial explains how we can replace values of a Pandas Series with another value using the Series.map()
method.
import pandas as pd
my_series = pd.Series([85, 87, 90, 89], index=["1", "2", "3", "4"])
print(my_series, "\n")
Output:
1 85
2 87
3 90
4 89
dtype: int64
We will be using the Series my_series
displayed in the above example to explain the working of the map()
method in Pandas.
pandas.Series.map()
Syntax
Series.map(arg, na_action=None)
It returns a Series
object by replacing the values in the caller Series
object based on the arg
parameter. The arg
may be a function
, dictionary
or Series
object which determines what are the new values of the Series
object.
The na_action
parameter can take None
or 'ignore'
as its value. The 'ignore'
value of the na_action
indicates ignoring the NaN
values in the Series
and do nothing to them.
Example: Use map()
Method for a Pandas Series
import pandas as pd
my_series = pd.Series([85, 87, 90, 89], index=["1", "2", "3", "4"])
altered_series = my_series.map({85: 80, 87: 80, 90: 90, 89: 80})
print("Initial Series:")
print(my_series, "\n")
print("Altered Series:")
print(altered_series, "\n")
Output:
Initial Series:
1 85
2 87
3 90
4 89
dtype: int64
Altered Series:
1 80
2 80
3 90
4 80
dtype: int64
It substitutes the elements of the my_series
depending upon the values specified in the dictionary passed as an argument to the map()
method.
We can also use functions to change the values of the Pandas Series by using the map()
method.
import pandas as pd
my_series = pd.Series([85, 87, 90, 89], index=["1", "2", "3", "4"])
altered_series = my_series.map(lambda x: str(x) + ".00")
print("Initial Series:")
print(my_series, "\n")
print("Altered Series:")
print(altered_series, "\n")
Output:
Initial Series:
1 85
2 87
3 90
4 89
dtype: int64
Altered Series:
1 85.00
2 87.00
3 90.00
4 89.00
dtype: object
It takes every element of my_series
and appends .00
at the end of each element in my_series
.
Example: Use map()
Method to Alter Particular Column of a DataFrame
import pandas as pd
df_1 = pd.DataFrame(
{
"Column 1": [85, 87, 90, 89],
"Column 2": [55, 54, 56, 66],
"Column 3": [23, 95, 65, 45],
},
index=["1", "2", "3", "4"],
)
print("Initial DataFrame:")
print(df_1, "\n")
df_1["Column 1"] = df_1["Column 1"].map(lambda x: x * 10)
print("DataFrame after changing Column 1:")
print(df_1)
Output:
Initial DataFrame:
Column 1 Column 2 Column 3
1 85 55 23
2 87 54 95
3 90 56 65
4 89 66 45
DataFrame after changing Column 1:
Column 1 Column 2 Column 3
1 850 55 23
2 870 54 95
3 900 56 65
4 890 66 45
It will apply the lambda
function only to Column 1
of the DataFrame using the map()
method. As single column is a Series
object, we can use the map()
method with a column of DataFrame. We then assign the returned Series
object from the map()
method back to the Column 1
of the df_1
DataFrame. In this way, we can change values of the particular column only of a DataFrame.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn