How to Convert Pandas Series to DataFrame
-
Convert a Single Pandas
Series
toDataFrame
Usingpandas.DataFrame()
-
Convert a Single Pandas
Series
toDataFrame
Usingpandas.Series.to_frame()
- Convert Multiple Pandas Series to Dataframes
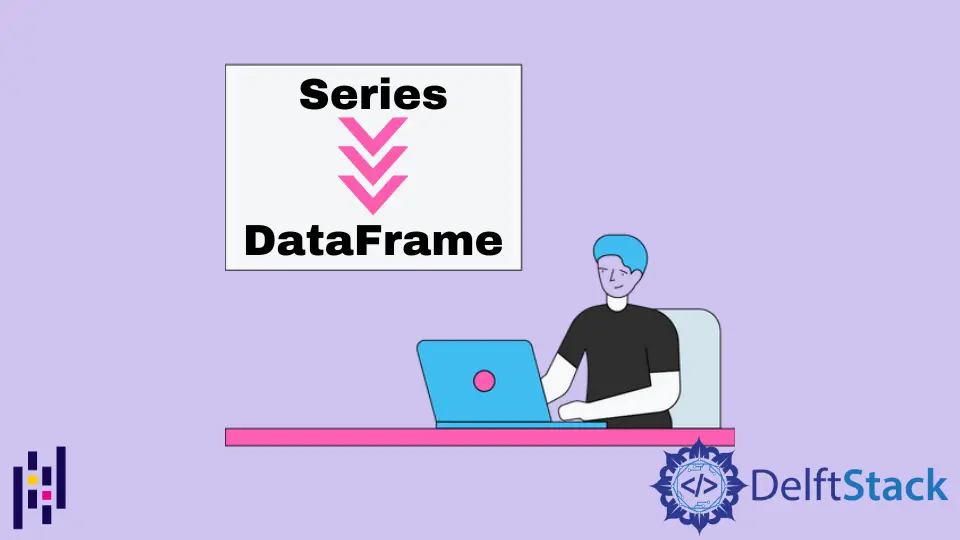
The creation of newer columns out of the derived or existing Series
is a formidable activity in feature engineering. The newly created Series
or column can be converted into Dataframe using pandas’ native functions.
In this article, We will introduce how to convert Pandas Series
to Dataframe.
In the following examples, we will work on the dataframe created using the following snippet.
import pandas as pd
import numpy as np
np.random.seed(0)
df_series = pd.Series(
np.random.randint(0, 100, size=(10)),
index=["a", "b", "c", "d", "e", "f", "g", "h", "i", "j"],
)
print(df_series)
Output:
a 44
b 47
c 64
d 67
e 67
f 9
g 83
h 21
i 36
j 87
dtype: int64
Convert a Single Pandas Series
to DataFrame
Using pandas.DataFrame()
The Series
can be transformed into a Dataframe using the DataFrame()
constructor by sending the Pandas Series
as an argument.
import pandas as pd
import numpy as np
np.random.seed(0)
df_series = pd.Series(
np.random.randint(0, 100, size=(10)),
index=["a", "b", "c", "d", "e", "f", "g", "h", "i", "j"],
)
print(pd.DataFrame(df_series, columns=["A"]))
Output:
A
a 81
b 37
c 25
d 77
e 72
f 9
g 20
h 80
i 69
j 79
As seen above, the output of the function returns a Dataframe.
Convert a Single Pandas Series
to DataFrame
Using pandas.Series.to_frame()
This to_frame()
function converts the given Pandas Series
to a Dataframe. The name of the column can be set with the name
argument.
import pandas as pd
import numpy as np
np.random.seed(0)
df_series = pd.Series(
np.random.randint(0, 100, size=(10)),
index=["a", "b", "c", "d", "e", "f", "g", "h", "i", "j"],
)
print(df_series.to_frame(name="A"))
Output:
A
a 44
b 47
c 64
d 67
e 67
f 9
g 83
h 21
i 36
j 87
There can be scenarios where the given series doesn’t have any name to it. In situations like these, the reset_index()
method can come in handy.
import pandas as pd
import numpy as np
np.random.seed(0)
df_series = pd.Series(
np.random.randint(0, 100, size=(10)),
index=["a", "b", "c", "d", "e", "f", "g", "h", "i", "j"],
).rename_axis("index")
print(df_series)
print("\n")
print(df_series.reset_index())
Output:
index
a 44
b 47
c 64
d 67
e 67
f 9
g 83
h 21
i 36
j 87
dtype: int64
index 0
0 a 44
1 b 47
2 c 64
3 d 67
4 e 67
5 f 9
6 g 83
7 h 21
8 i 36
9 j 87
As seen above, the created dataframe contains a new column named 0
. The column named 0
can be renamed using the name
argument that can be provided to the reset_index()
function, as shown below.
import pandas as pd
import numpy as np
np.random.seed(0)
df_series = pd.Series(
np.random.randint(0, 100, size=(10)),
index=["a", "b", "c", "d", "e", "f", "g", "h", "i", "j"],
).rename_axis("index")
print(df_series)
print("\n")
print(df_series.reset_index(name="A"))
Output:
index
a 44
b 47
c 64
d 67
e 67
f 9
g 83
h 21
i 36
j 87
dtype: int64
index A
0 a 44
1 b 47
2 c 64
3 d 67
4 e 67
5 f 9
6 g 83
7 h 21
8 i 36
9 j 87
Convert Multiple Pandas Series to Dataframes
The above examples have demonstrated the ability to convert just a single Pandas Series
to a Dataframe. What if there were multiple Series
, and these need to be stitched together to one single Dataframe? Upon creating the individual Series, the dataframe can be created by concatenating using the concat()
function.
import pandas as pd
import numpy as np
np.random.seed(0)
df_series1 = pd.Series(
np.random.randint(0, 100, size=(10)),
index=["a", "b", "c", "d", "e", "f", "g", "h", "i", "j"],
)
df_series2 = pd.Series(
np.random.randint(40, 100, size=(10)),
index=["a", "b", "c", "d", "e", "f", "g", "h", "i", "j"],
)
df_series3 = pd.Series(
np.random.randint(80, 100, size=(10)),
index=["a", "b", "c", "d", "e", "f", "g", "h", "i", "j"],
)
df_stitched = pd.concat([df_series1, df_series2, df_series3], axis=1)
print(df_stitched)
Output:
0 1 2
a 44 46 97
b 47 64 85
c 64 64 93
d 67 52 88
e 67 98 89
f 9 41 99
g 83 78 96
h 21 79 99
i 36 63 85
j 87 86 95
Note that the pd.concat()
function included an axis=1
argument, which corresponds to the append along the column. If axis
is not provided, there will be a row merge or union across all the Dataframes.
Related Article - Pandas DataFrame
- How to Get Pandas DataFrame Column Headers as a List
- How to Delete Pandas DataFrame Column
- How to Convert Pandas Column to Datetime
- How to Convert a Float to an Integer in Pandas DataFrame
- How to Sort Pandas DataFrame by One Column's Values
- How to Get the Aggregate of Pandas Group-By and Sum